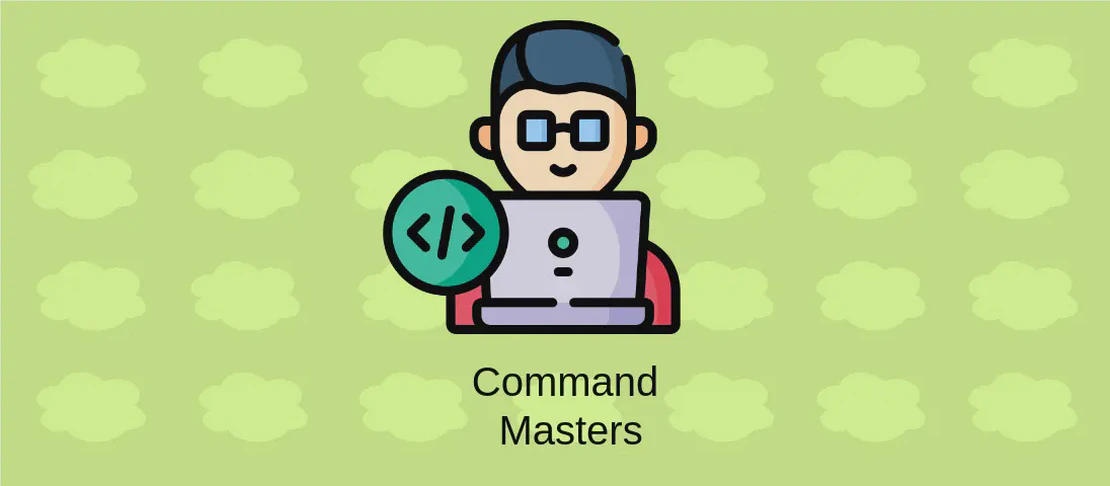
How to Use the Command 'ltrace' (with Examples)
- Linux
- December 17, 2024
ltrace
is a powerful diagnostic tool used to monitor and intercept dynamic library calls made by a program during its execution. By tracing these calls, developers can gain insights into how a program interacts with shared libraries, allowing for debugging, performance analysis, and troubleshooting. This tool is particularly useful for diagnosing misconfigurations or unexpected behaviors in applications, as well as for educational purposes to learn more about dynamic linking and program dependencies.
Trace the Library Calls of a Program
Code:
ltrace ./program
Motivation:
Tracing library calls of a program provides a clear picture of which shared libraries are being utilized during the execution of a binary. This is especially useful for developers who wish to debug or analyze a program’s behavior in detail. By observing the flow of dynamic library calls, developers can pinpoint performance bottlenecks or understand the sequence of operations carried out by the program. Furthermore, this can assist in diagnosing why a program might not be functioning as intended, by identifying incorrect or unexpected calls to shared libraries.
Explanation:
ltrace
: This command invokes theltrace
tool to monitor library calls../program
: This specifies the executable thatltrace
will monitor. The./
denotes that the program is located in the current working directory.
Example Output:
__libc_start_main(0x400540, 1, 0x7fff25a9dc38, 0x400680 <unfinished ...>
puts("Hello, world!"Hello, world!
) = 14
+++ exited (status 0) +++
In this output, ltrace
shows that the program calls the puts
function from the C library to print “Hello, world!” to the console, providing valuable insights into the program’s interaction with shared libraries.
Count Library Calls with Summary
Code:
ltrace -c path/to/program
Motivation:
Understanding the frequency and distribution of library calls is fundamental for performance tuning and optimization. Using ltrace
to count library calls provides developers and analysts with a statistical summary of how many times each call is made without being overwhelmed by individual call details. This leads to a more data-driven approach to identifying performance latency related to dynamic libraries and aids in recognizing trends in library usage.
Explanation:
ltrace
: Executes theltrace
command for tracing library calls.-c
: This flag tellsltrace
to count the number of calls to each library function and provide a summary.path/to/program
: Specifies the path to the executable program being traced.
Example Output:
% time seconds usecs/call calls function
------ ----------- ----------- --------- --------------------
100.00 0.000052 0 3 malloc
0.00 0.000000 0 4 free
This output shows that three calls to malloc
and four calls to free
were made, concentrating more on the frequency rather than the specifics of each call.
Trace Specific Library Calls While Omitting Others
Code:
ltrace -e malloc+free-@libc.so* path/to/program
Motivation:
Sometimes, tracing every library call is not necessary or can result in overwhelming output, especially for programs with extensive dynamic library usage. Therefore, focusing on specific calls of interest—like memory allocations and deallocations using malloc
and free
—can be more effective. By excluding those calls that are already known or irrelevant, developers can hone in on particular interactions that may be problematic or crucial for analysis.
Explanation:
ltrace
: The tool being used to trace library calls.-e malloc+free-@libc.so*
: This option instructsltrace
to trace onlymalloc
andfree
functions, avoiding any calls to these functions made by thelibc
library, providing more targeted and filtered output.path/to/program
: This represents the path to the specific program to be analyzed.
Example Output:
malloc(24) = 0x5563c686b270
free(0x5563c686b270) = <void>
This result provides a selective view of relevant library interactions, focusing only on the malloc
and free
calls outside the standard C library, simplifying the debugging process.
Write Trace Output to a File
Code:
ltrace -o file path/to/program
Motivation:
When managing large outputs from ltrace
, redirecting the output to a file is advantageous. Doing so enables developers to keep logs of program execution for further analysis, comparison, or record-keeping. This method also helps when running ltrace
in automated scripts, where results need to be stored and analyzed post-process. Moreover, storing large volumes of output data in files makes it easier to apply text processing and analysis tools for deeper insights.
Explanation:
ltrace
: Calls theltrace
tool for monitoring dynamic library calls.-o file
: Redirects the output ofltrace
to the specified file instead of displaying it on the terminal, thereby creating an archive for later review.path/to/program
: Specifies the path of the executable whose library calls will be traced and logged.
Example Output:
Assuming the result is written to a file named output.txt
, the file contains:
open("file.txt", O_RDONLY) = 3
read(3, "The quick brown fox jumps over the lazy dog", 4096) = 44
close(3) = 0
The contents of output.txt
capture all intercepted calls to open
, read
, and close
, which are stored for subsequent examination.
Conclusion
The ltrace
command is a versatile and essential tool for monitoring dynamic library calls within a software application. By offering various options like summarizing call counts, filtering specific library calls, and storing output in files, it supports multiple strategies for performance analysis, debugging, and understanding dynamic linking, allowing developers to optimize and troubleshoot their applications effectively.