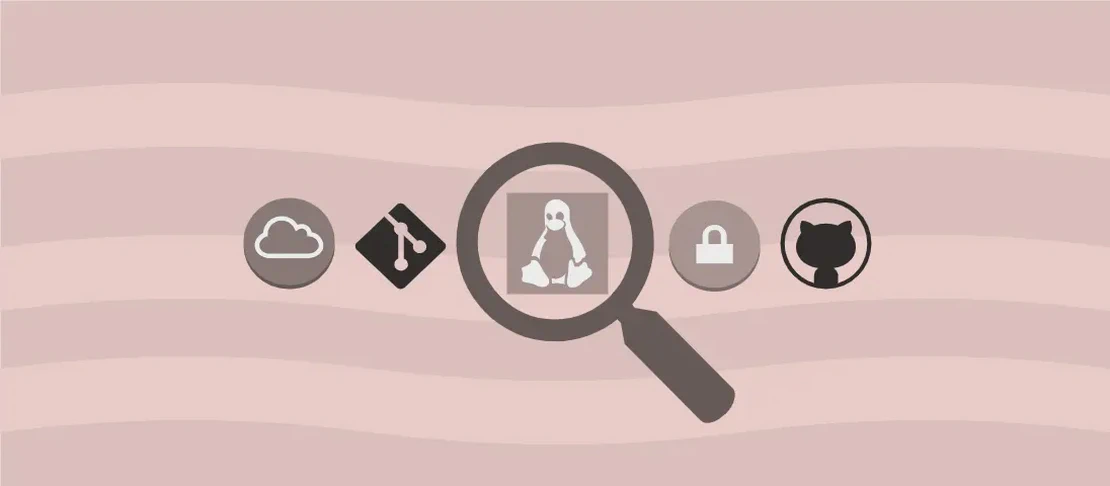
How to use the command 'luajit' (with examples)
LuaJIT is a Just-In-Time (JIT) compiler for the Lua programming language. Lua is renowned for its lightweight and embeddable capabilities, making it a popular choice for embedding into applications. LuaJIT enhances the performance of Lua by converting Lua scripts into machine code at runtime, greatly improving execution speed. LuaJIT can be used in various scenarios including interactive development, script execution, and evaluating expressions. This article provides practical examples on how to leverage LuaJIT effectively.
Use case 1: Start an interactive Lua shell
Code:
luajit
Motivation:
Starting an interactive Lua shell with LuaJIT can be immensely useful for developers who need to quickly test snippets of Lua code, experiment with language features, or debug specific logic without the overhead of running an entire script. This interactive environment allows for immediate feedback, facilitating a rapid development cycle and promotes an exploratory programming approach, which is particularly beneficial for newcomers to the language who are still getting accustomed to Lua’s syntax and semantics.
Explanation:
There are no additional arguments in this command since simply typing luajit
and executing it launches the Lua interactive shell. This opens a shell interface where commands can be typed and executed in real-time.
Example Output:
Upon executing the command, the screen will display a Lua interactive prompt, which will look something like this:
JIT: ON dinst, vmatch, folding "FFI"
At this stage, a user can input Lua code directly into the console and see immediate results. For example, typing print("Hello from LuaJIT")
will output:
Hello from LuaJIT
Use case 2: Execute a Lua script
Code:
luajit path/to/script.lua --optional-argument
Motivation:
Executing a Lua script using LuaJIT can dramatically improve the performance of Lua programs due to its efficient compilation process. This is particularly important in production environments where performance is critical, such as in gaming engines, embedded systems, or large-scale web services where Lua is used, and speed is of the essence.
Explanation:
luajit
: This command calls the LuaJIT interpreter and compiler.path/to/script.lua
: This specifies the path to the Lua script you intend to execute. It must be a valid path to an existing.lua
file.--optional-argument
: Any additional arguments required by the Lua script can be passed after the script path. These arguments can be accessed within the script using thearg
table.
Example Output:
If you have a Lua script at path/to/script.lua
containing:
print("Lua script executed with argument: ", arg[1])
Running luajit path/to/script.lua hello
will produce:
Lua script executed with argument: hello
Use case 3: Execute a Lua expression
Code:
luajit -e 'print("Hello World")'
Motivation:
Executing Lua expressions directly from the command line is a convenient way for developers to perform quick calculations, generate small outputs, or conduct simple tasks without the need to create and save an entire script file separately. This efficiency is beneficial for automating tasks and integrating Lua functionality within shell scripts or other command-line pipelines.
Explanation:
luajit
: Invokes the LuaJIT interpreter.-e
: This flag indicates that the following text is a Lua expression to be executed directly. It tells LuaJIT to evaluate the subsequent string as a Lua program.'print("Hello World")'
: This is the Lua expression being executed, which in this case is a simple print statement to output “Hello World”.
Example Output:
Executing this command in the terminal will instantly output:
Hello World
Conclusion
LuaJIT is an incredibly versatile tool for anyone working with Lua, enhancing its speed and providing flexible options for executing code. Whether you’re tinkering with code snippets in an interactive shell, running scripts with possible arguments, or executing small expressions on-the-fly, LuaJIT provides a powerful feature set that serves a broad range of development needs. Understanding these use cases can greatly benefit everyday Lua programming tasks and improve overall productivity.