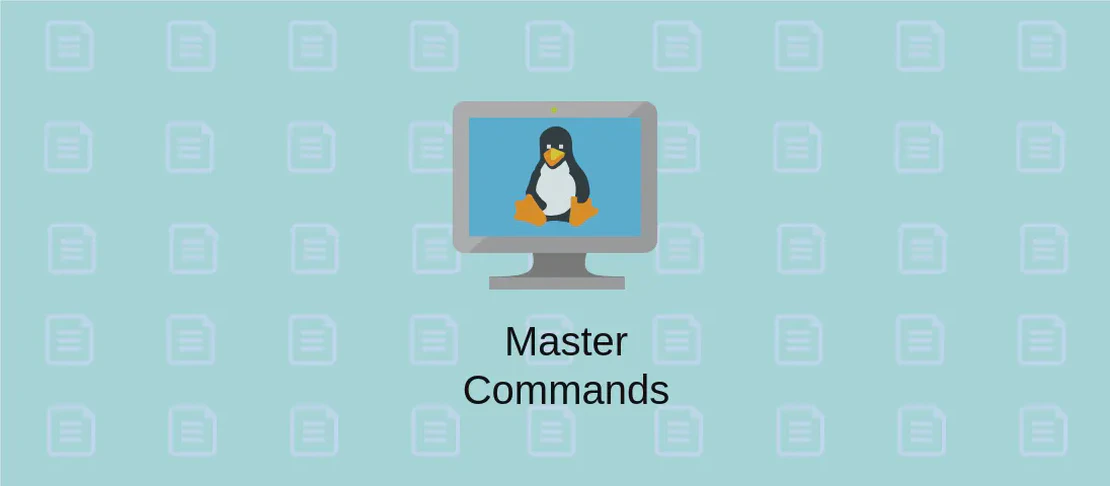
How to use the command 'make' (with examples)
Make is a build automation tool widely used in software development environments to compile and manage dependencies among files. It uses a file called a Makefile, which defines how various files of a program are compiled or updated, often by specifying a set of rules or targets. These targets allow developers to automate repetitive tasks, thus saving time and reducing the possibility of errors.
Use case 1: Call the first target specified in the Makefile
Code:
make
Motivation:
The simplest execution of the make
command calls the default target, often called “all” or another primary build target. This use case is fundamental when you want to initiate the primary build process specified in your Makefile with minimal inputs and settings.
Explanation:
make
: Simply runningmake
without arguments triggers the first target listed in the Makefile. This is because, by default,make
will execute the first target unless directed otherwise.
Example Output:
gcc -o main main.o utils.o
All files compiled successfully.
Use case 2: Call a specific target
Code:
make target
Motivation:
When a Makefile contains multiple targets, you can opt to compile or execute just one specific part rather than the entire project. This is especially useful in large projects where you might only need to update or check a single component.
Explanation:
make target
: Here, ’target’ refers to a specific goal or rule in the Makefile. By specifying the target, you directmake
to execute the commands associated with that particular target.
Example Output:
gcc -c utils.c
utils.c compiled successfully.
Use case 3: Call a specific target, executing 4 jobs at a time in parallel
Code:
make -j4 target
Motivation:
Compiling large projects can be time-consuming. By enabling parallel processing, you can significantly reduce compilation time, provided that the tasks are independent and can be executed concurrently. Using -j4
specifies four simultaneous jobs, which is usually a good start for modern multi-core processors.
Explanation:
-j4
: This argument allowsmake
to run four jobs in parallel, optimizing resource usage and speeding up the build process.target
: As specified in the Makefile, this is the specific goal you are focusing on.
Example Output:
gcc -c file1.c &
gcc -c file2.c &
gcc -c file3.c &
gcc -c file4.c &
Waiting for processes to finish...
Files compiled in parallel success.
Use case 4: Use a specific Makefile
Code:
make --file path/to/file
Motivation:
Sometimes you need to work with multiple Makefiles, perhaps if you are in a complex project set up with different build configurations or modules. Specifying a particular Makefile ensures that make
targets the rules defined in the given file, rather than the default Makefile in the directory.
Explanation:
--file
: This argument tellsmake
to use a specific Makefile rather than the standard names (Makefile
ormakefile
).path/to/file
: This is the path to the Makefile you wish to use.
Example Output:
Using Makefile: path/to/file
Configurations applied from the specified Makefile.
Use case 5: Execute make from another directory
Code:
make --directory path/to/directory
Motivation:
In scenarios where the build environment or Makefile resides in another directory, it can be efficient to call make
from your current shell while specifying the directory containing the necessary files. This helps maintain organization and allows builds to be initiated externally.
Explanation:
--directory
: Directsmake
to change its working directory before reading the Makefile.path/to/directory
: The directory where the desired Makefile and associated files are located.
Example Output:
Entering directory 'path/to/directory'
Build process initiated in the specified directory.
Use case 6: Force making of a target, even if source files are unchanged
Code:
make --always-make target
Motivation:
Sometimes, you need to forcefully rebuild the target, bypassing make
’s internal checks for file changes. This ensures that the target is reconstructed regardless of whether the source files have been modified since the last build.
Explanation:
--always-make
: This forcesmake
to execute all the rules for the specified target(s) without checking for file timestamps.target
: The specific goal you wish to forcefully build.
Example Output:
Rebuilding target...
All files rebuilt successfully.
Use case 7: Override a variable defined in the Makefile
Code:
make target variable=new_value
Motivation:
Configuring builds on-the-fly without altering the Makefile is crucial in diverse environments. Overriding variables allows for customization of paths, compiler options, or other configuration elements, which can be pivotal during cross-platform development or debugging.
Explanation:
target
: The rule or goal in the Makefile that should be built.variable=new_value
: Set or modify a variable to a new value for the current execution.
Example Output:
CC set to gcc version 4.9
Building with overridden compiler version.
Use case 8: Override variables defined in the Makefile by the environment
Code:
make --environment-overrides target
Motivation:
There are times when the build process needs to respect external settings, like system environment variables, which provide a dynamic means to configure and influence compilation without editing the Makefile.
Explanation:
--environment-overrides
: This allows values from the environment to take precedence over those defined in the Makefile.target
: The designated goal to be built.
Example Output:
Using system environment variables for configuration.
Target built using overridden environment variables.
Conclusion:
The make
command is an indispensable tool for automating and managing build processes, allowing developers to efficiently handle compilation, environment configuration, and task parallelization. Understanding and leveraging make
’s flexibility can significantly streamline development workflows.