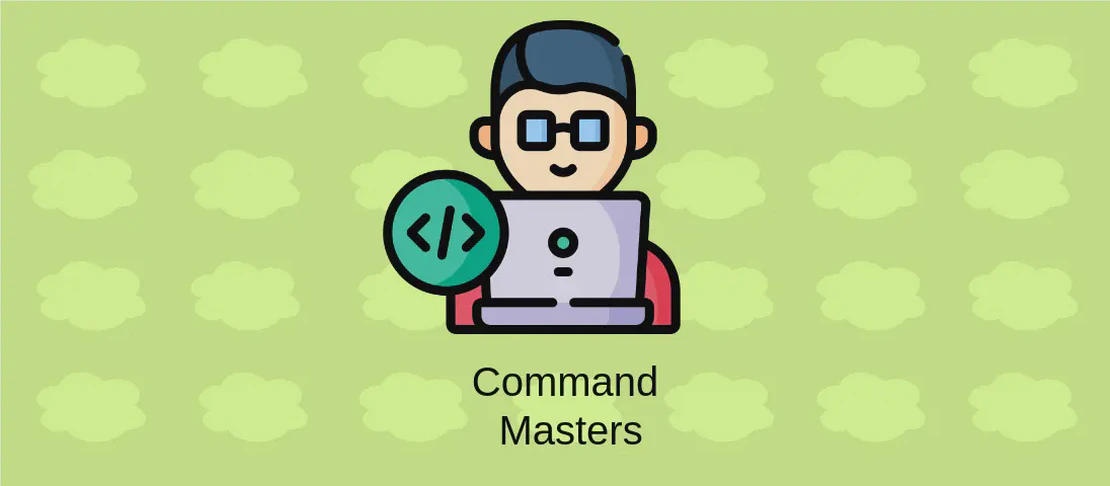
How to use the command 'mcs' (with examples)
The Mono C# Compiler, abbreviated as mcs
, is a key tool used for compiling C# source files in the Mono development framework. It allows developers to transform C# code into executable programs or libraries that run on the Common Language Runtime (CLR). Developers often use mcs
to compile .NET applications cross-platform, taking advantage of Mono’s broader compatibility.
Use case 1: Compiling the specified files
Code:
mcs path/to/input_file1.cs path/to/input_file2.cs ...
Motivation:
As a C# developer, there are instances when you need to compile multiple source files simultaneously. This command allows you to efficiently compile all necessary files in one go, streamlining the development process by reducing manual steps and saving time.
Explanation:
mcs
: Invokes the Mono C# Compiler to start the compilation process.path/to/input_file1.cs path/to/input_file2.cs ...
: These paths indicate the C# source files you want to compile. You can specify multiple files by separating them with spaces. Each file path should be correctly specified relative to your current working directory.
Example output:
Upon successful compilation, this command will produce a single executable file named output.exe
by default. If any syntax or semantic errors are detected during the process, the compiler will output error messages specifying the nature and location of each issue.
Use case 2: Specify the output program name
Code:
mcs -out:path/to/file.exe path/to/input_file1.cs path/to/input_file2.cs ...
Motivation:
Often, developers need to designate a specific name for the compiled executable for better organization, maintainability, or to adhere to certain naming conventions within their project. By specifying the output name, you avoid the default naming, creating files that are easier to identify and manage in larger projects.
Explanation:
mcs
: Activates the Mono C# Compiler to initiate file compilation.-out:path/to/file.exe
: This flag specifies the desired output file name and path for the compiled executable. The output path should be correctly attributed.path/to/input_file1.cs path/to/input_file2.cs ...
: These are the source files that will be compiled, and you can include multiple files.
Example output:
This command compiles the input files into a single executable with a user-defined name, such as path/to/file.exe
. An accurate output will depend on coding contributions; errors in the source code will display the respective error messages.
Use case 3: Specify the output program type
Code:
mcs -target:exe|winexe|library|module path/to/input_file1.cs path/to/input_file2.cs ...
Motivation:
Depending on your application’s purpose, you might need to compile your source files into different types of outputs. For example, you may want a console application, a GUI application, or a library. This command permits the compiler to target different output types, making it favorable for scenarios involving diverse deployment environments or modular architecture designs.
Explanation:
mcs
: Calls upon the Mono C# Compiler to compile given C# source files.-target:exe|winexe|library|module
: This option designates the type of output program the compiler should produce:exe
: A console application.winexe
: A Windows GUI application.library
: A dynamic link library (DLL).module
: An intermediate file not directly executable.
path/to/input_file1.cs path/to/input_file2.cs ...
: These are the C# source files marked for compilation, which can be numerous as needed.
Example output:
The resultant output file could be an executable, shared library, or a module, contingent upon the selected target type. For instance, specifying -target:library
creates a .dll
file, integrating seamlessly into other projects or solutions while respecting any compiling errors that flag similar to prior use cases.
Conclusion:
Engaging the mcs
command aligns with crucial facets of managing C# projects within Mono, furnishing developers with multiple options for compiling code into executable programs or libraries. Each use case simplifies work on complex projects, whether it involves compiling multiple files, customizing output names, or producing specific types of compilations to meet distinct requirements.