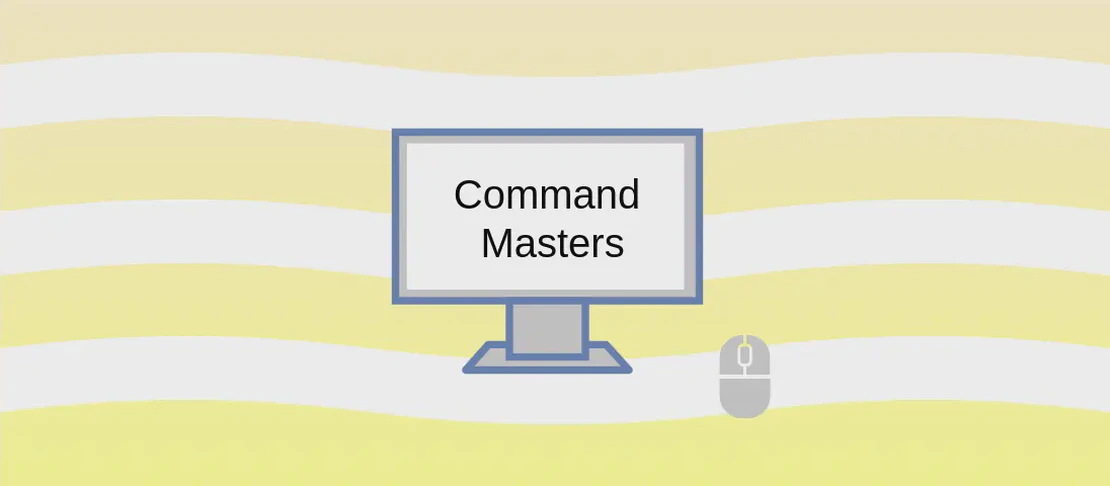
How to Use the `Measure-Command` in PowerShell (with examples)
- Windows
- December 17, 2024
The Measure-Command
cmdlet in PowerShell is a powerful tool that allows users to evaluate the efficiency and performance of script blocks and cmdlets by measuring the time it takes to execute them. This cmdlet is particularly useful for optimizing code, comparing different approaches, and identifying bottlenecks. By providing execution time in milliseconds, Measure-Command
makes it easier to fine-tune scripts for optimal performance.
Use case 1: Measure the time it takes to run a command
Code:
Measure-Command { Get-Process }
Motivation:
Understanding how long it takes for a particular command to execute is crucial for efficiency and performance optimization, especially when dealing with complex scripts or systems where processing time might be a limiting factor. For instance, measuring how long it takes for the Get-Process
command to retrieve all running processes can help assess system performance and the impact of running this query within larger scripts. For IT administrators and developers, knowing these details can inform decisions about script structure and the timing for executing specific commands within a sequence.
Explanation:
Measure-Command
: The cmdlet used to measure the execution time of the provided script block.{ Get-Process }
: This script block encompasses theGet-Process
cmdlet within curly braces. The cmdlet lists all processes currently running on the system. By using it withMeasure-Command
, we evaluate how long it takes to retrieve this information.
Example Output:
The output typically contains information such as:
Days : 0
Hours : 0
Minutes : 0
Seconds : 0
Milliseconds : 405
Ticks : 4052800
TotalDays : 4.69074074074074E-06
TotalHours : 0.000112577777777778
TotalMinutes : 0.00675466666666667
TotalSeconds : 0.40528
TotalMilliseconds : 405.28
The output shows that the Get-Process
command took approximately 405 milliseconds to execute. Such data can be valuable when analyzing system performance and script efficiency.
Use case 2: Pipe input to Measure-Command
Code:
10, 20, 50 | Measure-Command -Expression { for ($i=0; $i -lt $_; $i++) {$i} }
Motivation:
In scenarios where a command or script needs to process multiple inputs, measuring how it performs with a range of data can be insightful. This example demonstrates how we can measure the execution time of a script block that processes piped data inputs through looping logic. Developers can benefit from using this technique to understand the scalability and efficiency of loops or data processing mechanisms within their scripts, particularly when dealing with arrays or collections that vary in size.
Explanation:
10, 20, 50
: These are the input numbers piped intoMeasure-Command
. Each number represents a separate case or workload for the script block to process.Measure-Command
: This cmdlet measures how long the execution of the following block takes, iteratively processing each piped value.-Expression
: The parameter that holds the script block.{ for ($i=0; $i -lt $_; $i++) {$i} }
: This script block contains a loop that iterates from 0 to each of the piped values (10, then 20, then 50). The$_
variable within the block represents each item piped in, causing the loop to adjust its iterations based on these specific inputs.
Example Output:
The output will provide time measurements for the block as:
Days : 0
Hours : 0
Minutes : 0
Seconds : 0
Milliseconds : 2
Ticks : 24100
TotalDays : 2.78935185185185E-08
TotalHours : 6.69444444444444E-07
TotalMinutes : 4.01666666666667E-05
TotalSeconds : 0.0024103
TotalMilliseconds : 2.4103
This shows that the expression, consisting primarily of a loop from 0 to the piped values, took approximately 2 milliseconds. This is useful information for optimizing loop performance as data sizes change.
Conclusion:
Understanding the execution time of PowerShell commands through Measure-Command
is crucial for optimizing scripts and maintaining system efficiency. Whether testing standalone commands or complex loops with varying inputs, Measure-Command
provides detailed insights on execution duration, facilitating informed decisions on efficient command structuring and system resource management.