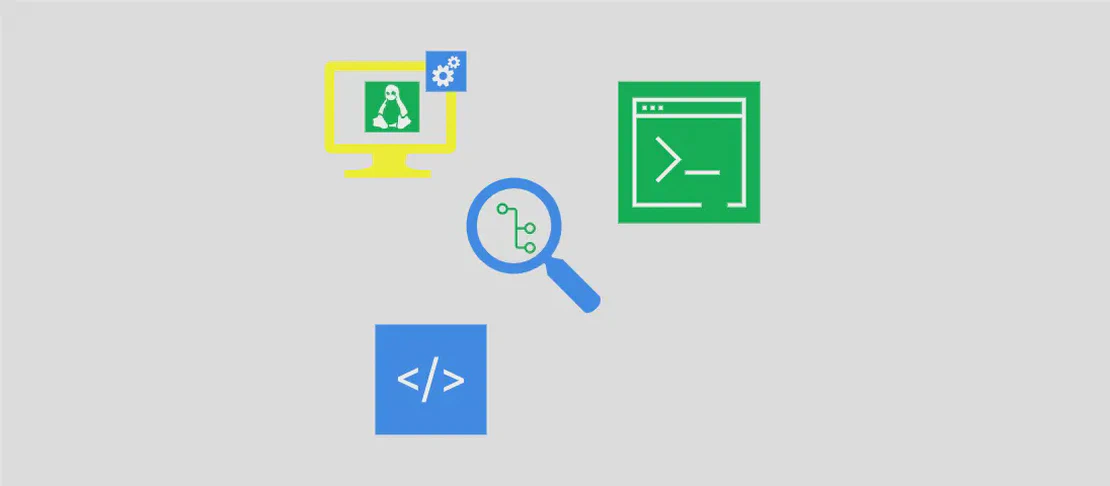
How to use the command 'monodis' (with examples)
The monodis
command is a tool associated with the Mono project, which is an open-source implementation of Microsoft’s .NET Framework. The primary function of monodis
is to disassemble assemblies in the Mono Common Intermediate Language (CIL) format into a human-readable textual representation. It aids developers in understanding and analyzing CIL code and provides valuable insights into the structure of .NET assemblies. This tool is particularly useful for those interested in delving into the inner workings of .NET applications, debugging, or simply learning more about assembly-level programming within the .NET ecosystem.
Use case 1: Disassemble an assembly to textual CIL
Code:
monodis path/to/assembly.exe
Motivation:
Disassembling an assembly into CIL is incredibly valuable for developers seeking to understand the low-level implementation details of .NET applications. Whether you are in the process of debugging complex logic or just curious about how high-level code translates into CIL, this command provides a clear and readable output of the assembly’s CIL instructions.
Explanation:
monodis
: This is the command used to invoke the Mono Common Intermediate Language disassembler.path/to/assembly.exe
: This argument specifies the path to the assembly file you wish to disassemble. The assembly can be any CLI file with compiled .NET code, typically an.exe
or.dll
.
Example Output:
.method public hidebysig static void Main(string[] args) cil managed
{
.entrypoint
// Code size 11 (0xb)
.maxstack 8
IL_0000: ldstr "Hello, World!"
IL_0005: call void [mscorlib]System.Console::WriteLine(string)
IL_000a: ret
}
Use case 2: Save the output to a file
Code:
monodis --output=path/to/output.il path/to/assembly.exe
Motivation:
Saving the disassembled output to a file can be beneficial for documentation purposes, thorough offline analysis, or further processing by other tools or scripts. It allows you to revisit the CIL code without disassembling the assembly again, thereby saving time.
Explanation:
monodis
: The command for the CIL disassembler.--output=path/to/output.il
: This flag specifies the file path where the disassembled output should be saved. The generated file will contain the text representation of the CIL.path/to/assembly.exe
: Indicates which assembly file should be disassembled.
Example Output:
A file located at path/to/output.il
with content similar to:
.method public hidebysig static void Main(string[] args) cil managed
{
.entrypoint
// Code size 11 (0xb)
.maxstack 8
IL_0000: ldstr "Hello, World!"
IL_0005: call void [mscorlib]System.Console::WriteLine(string)
IL_000a: ret
}
Use case 3: Show information about an assembly
Code:
monodis --assembly path/to/assembly.dll
Motivation:
Understanding metadata about an assembly is crucial for developers and reverse engineers. The metadata includes information such as the assembly version, culture, and public key token. This data can help you understand the assembly’s identity and dependencies, which is vital for compatibility and security analysis.
Explanation:
monodis
: The command for accessing the Mono CIL disassembler.--assembly
: This option instructsmonodis
to display the assembly metadata instead of disassembling its method bodies.path/to/assembly.dll
: The path to the assembly whose metadata you wish to inspect.
Example Output:
Assembly Name: MyAssembly
Version: 1.0.0.0
Culture: neutral
Public Key Token: null
Use case 4: List the references of an assembly
Code:
monodis --assemblyref path/to/assembly.exe
Motivation:
Listing references helps developers understand which external assemblies a given assembly relies on. This is particularly useful when troubleshooting dependency issues or when preparing an application for deployment to ensure all necessary components are included.
Explanation:
monodis
: The command that provides insight into the assembly.--assemblyref
: A flag indicating the command should output the referenced assemblies by the target.path/to/assembly.exe
: Specifies the path to the assembly being examined.
Example Output:
Referenced Assembly: mscorlib, Version=2.0.0.0, Culture=neutral, Public Key Token=b77a5c561934e089
Referenced Assembly: System, Version=2.0.0.0, Culture=neutral, Public Key Token=b77a5c561934e089
Use case 5: List all the methods in an assembly
Code:
monodis --method path/to/assembly.exe
Motivation:
Listing all methods in an assembly provides a high-level overview of the assembly’s functionalities and can be crucial for reverse engineering tasks or understanding the potential attack surface of the application from a security perspective.
Explanation:
monodis
: The primary command for retrieving information about the assembly.--method
: This flag tellsmonodis
to enumerate all methods implemented in the assembly.path/to/assembly.exe
: The path to the assembly from which you want to list methods.
Example Output:
Method 1: void Main(string[])
Method 2: int CalculateSum(int, int)
Method 3: string GetGreeting()
Use case 6: List resources embedded within an assembly
Code:
monodis --manifest path/to/assembly.dll
Motivation:
Knowing what resources are embedded within an assembly is important for understanding the full extent of the assembly’s capabilities and data. Resources may include images, files, strings, and other data that the application might use during execution.
Explanation:
monodis
: The command used to inspect the CIL assembly.--manifest
: This option is used to list all the embedded resources detailed in the assembly’s manifest.path/to/assembly.dll
: The path to the target assembly file.
Example Output:
Resource 1: MyAssembly.Resources.Strings.en-US.resources
Resource 2: MyAssembly.Resources.Images.Logo.png
Use case 7: Extract all the embedded resources to the current directory
Code:
monodis --mresources path/to/assembly.dll
Motivation:
Extracting resources can be useful for developers needing to access data contained within an assembly without running the application. It can also aid in resource editing or retrieval for localization purposes or to inspect data security.
Explanation:
monodis
: The command for handling Mono CIL assemblies.--mresources
: A directive to extract and output all embedded resources to the current directory from the given assembly.path/to/assembly.dll
: The target assembly containing the resources you wish to extract.
Example Output: Files extracted in the current directory, such as:
- Strings.en-US.resources
- Logo.png
Conclusion:
The monodis
command is an invaluable tool for developers and reverse engineers working within the .NET framework, offering crucial insights into assembly structure, dependencies, methods, metadata, and resources. By leveraging monodis
, you can investigate, document, and analyze assemblies for a variety of purposes, ranging from debugging and learning to security analysis and application deployment preparation.