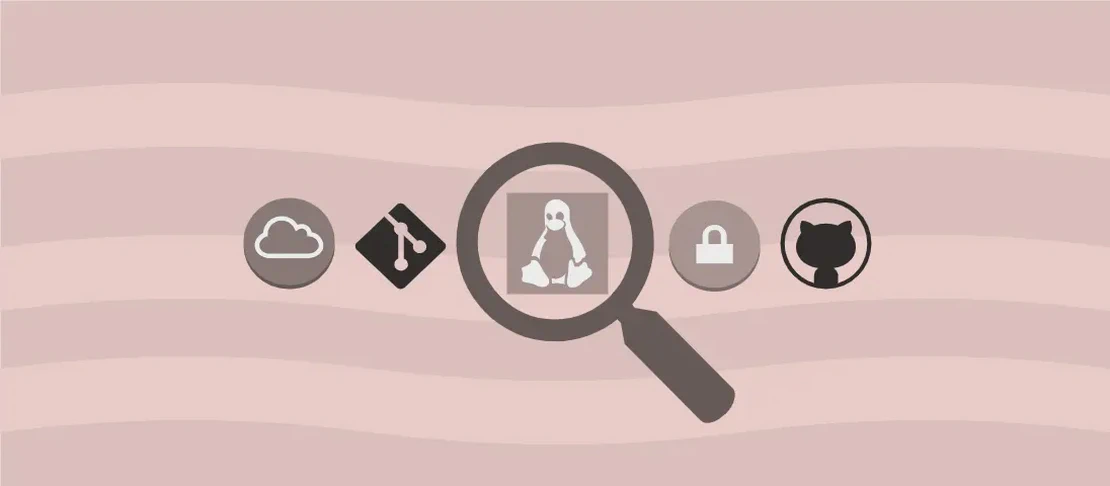
Exploring .NET Assemblies with Monop (with examples)
Introduction
Monop is a command-line tool that allows developers to find and display signatures of types and methods within .NET assemblies. This tool is particularly useful for inspecting and understanding the structure of .NET assemblies and their associated types. In this article, we will explore different use cases of the monop
command with code examples.
Use Case 1: Showing the Structure of a Built-in Type
To show the structure of a built-in type in the .NET Framework, you can use the following command:
monop System.String
Motivation: This use case is useful for developers who want to understand the structure and available members of a specific built-in type. For example, understanding the members of the System.String
type can help developers utilize its functionality effectively.
Explanation:
monop
is the command that invokes themonop
tool.System.String
is the name of the built-in type in the .NET Framework that we want to explore.
Example Output:
Type System.String
public string ToString(): string
public object Clone(): object
...
The output provides a list of members available in the System.String
type.
Use Case 2: Listing Types in an Assembly
To list all the types present in an assembly, you can use the following command:
monop -r:path/to/assembly.exe
Motivation: This use case is helpful when you want to get an overview of all the types present in a specific assembly. It allows you to quickly explore the structure of an assembly without diving into individual types.
Explanation:
monop
is the command that invokes themonop
tool.-r
is the option that specifies the path to the assembly file to analyze.path/to/assembly.exe
is the path to the assembly file.
Example Output:
Types in path/to/assembly.exe:
Namespace1.Type1
Namespace2.Type2
...
The output lists all the types present in the specified assembly.
Use Case 3: Showing the Structure of a Type in a Specific Assembly
To show the structure of a type in a specific assembly, you can use the following command:
monop -r:path/to/assembly.dll Namespace.Path.To.Type
Motivation: This use case allows you to inspect the structure of a specific type within an assembly. It is useful when you want to explore the members and signatures of a particular type without getting overwhelmed by the entire assembly.
Explanation:
monop
is the command that invokes themonop
tool.-r
is the option that specifies the path to the assembly file to analyze.path/to/assembly.dll
is the path to the assembly file.Namespace.Path.To.Type
is the fully qualified name of the type within the assembly.
Example Output:
Type Namespace.Path.To.Type
public void Method1(): void
public int Method2(string input): int
...
The output displays the structure of the specified type, including its methods and properties.
Use Case 4: Only Showing Members Defined in the Specified Type
To only show the members that are defined in the specified type, you can use the following command:
monop -r:path/to/assembly.dll --only-declared Namespace.Path.To.Type
Motivation: This use case is helpful when you want to focus only on the members that are directly defined in the specified type. It allows you to filter out inherited members and provides a cleaner view of the type’s structure.
Explanation:
monop
is the command that invokes themonop
tool.-r
is the option that specifies the path to the assembly file to analyze.path/to/assembly.dll
is the path to the assembly file.--only-declared
is the option that tellsmonop
to only show members that are declared in the specified type.Namespace.Path.To.Type
is the fully qualified name of the type within the assembly.
Example Output:
Type Namespace.Path.To.Type
public void Method1(): void
public int Method2(string input): int
...
The output displays only the members that are directly defined in the specified type.
Use Case 5: Showing Private Members
To show private members of a type, you can use the following command:
monop -r:path/to/assembly.dll --private Namespace.Path.To.Type
Motivation: This use case is useful when you want to inspect the private members of a type. It provides visibility into the implementation details of a type that may not be accessible in the public API.
Explanation:
monop
is the command that invokes themonop
tool.-r
is the option that specifies the path to the assembly file to analyze.path/to/assembly.dll
is the path to the assembly file.--private
is the option that tellsmonop
to show private members of the specified type.Namespace.Path.To.Type
is the fully qualified name of the type within the assembly.
Example Output:
Type Namespace.Path.To.Type
private int _privateField
private void PrivateMethod(): void
...
The output displays the private members of the specified type.
Use Case 6: Hiding Obsolete Members
To hide obsolete members in the output, you can use the following command:
monop -r:path/to/assembly.dll --filter-obsolete Namespace.Path.To.Type
Motivation: This use case is helpful when you want to filter out members that are marked as obsolete or deprecated. It provides a cleaner and more relevant view of the type’s structure.
Explanation:
monop
is the command that invokes themonop
tool.-r
is the option that specifies the path to the assembly file to analyze.path/to/assembly.dll
is the path to the assembly file.--filter-obsolete
is the option that tellsmonop
to hide members marked as obsolete.Namespace.Path.To.Type
is the fully qualified name of the type within the assembly.
Example Output:
Type Namespace.Path.To.Type
public void Method1(): void
public int Method2(string input): int
...
The output excludes members that are marked as obsolete.
Use Case 7: Listing Referenced Assemblies
To list the other assemblies that a specified assembly references, you can use the following command:
monop -r:path/to/assembly.dll --refs
Motivation: This use case is useful when you want to identify the dependencies of a specific assembly. It helps you understand the other assemblies that are required for the proper functioning of the specified assembly.
Explanation:
monop
is the command that invokes themonop
tool.-r
is the option that specifies the path to the assembly file to analyze.path/to/assembly.dll
is the path to the assembly file.--refs
is the option that tellsmonop
to list the other assemblies that the specified assembly references.
Example Output:
Referenced Assemblies for path/to/assembly.dll:
Assembly1.dll
Assembly2.dll
...
The output lists the other assemblies that the specified assembly references.
Conclusion
In this article, we explored different use cases of the monop
command with code examples. We saw how to show the structure of a built-in type, list types in an assembly, inspect a type’s structure in a specific assembly, filter members, and list referenced assemblies. The monop
command is a versatile tool that can greatly assist developers in understanding and analyzing .NET assemblies.