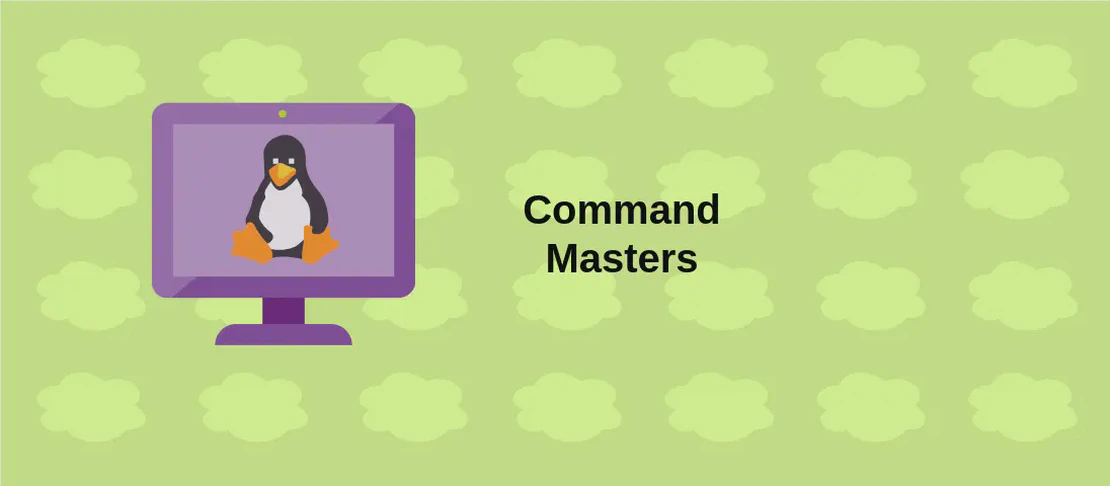
How to use the command Move-Item (with examples)
- Windows
- December 25, 2023
Move-Item is a PowerShell command that allows users to move or rename files, directories, registry keys, and other PowerShell data items. It is a versatile command that can be used for various purposes, such as organizing files, renaming files or directories, and manipulating registry keys. This command can only be executed through PowerShell.
Use case 1: Rename a file or directory when the target is not an existing directory
Code:
Move-Item path\to\source path\to\target
Motivation: This use case is useful when you want to rename a file or directory. It allows you to specify a new name for the source file or directory.
Explanation:
path\to\source
: This argument represents the path to the file or directory you want to rename.path\to\target
: This argument represents the new name or path of the file or directory.
Example output: The file or directory specified in the source path will be renamed with the new name or moved to the new target path.
Use case 2: Move a file or directory into an existing directory
Code:
Move-Item path\to\source path\to\existing_directory
Motivation: This use case is useful when you want to move a file or directory into an existing directory. It allows you to specify the target directory where you want to move the source file or directory.
Explanation:
path\to\source
: This argument represents the path to the file or directory you want to move.path\to\existing_directory
: This argument represents the path to the existing directory where you want to move the source file or directory.
Example output: The file or directory specified in the source path will be moved to the existing directory specified in the target path.
Use case 3: Rename or move file(s) with specific name (do not treat special characters inside strings)
Code:
Move-Item -LiteralPath "path\to\source" path\to\file_or_directory
Motivation: This use case is useful when you want to rename or move file(s) with a specific name, including special characters that should not be treated as part of the command.
Explanation:
-LiteralPath
: This parameter specifies that the path specified in the argument is treated literally and should not be treated as a wildcard or interpreted."path\to\source"
: This argument represents the path to the file or directory you want to rename or move.path\to\file_or_directory
: This argument represents the new name or path of the file or directory.
Example output: The file or directory specified in the source path will be renamed with the new name or moved to the new target path, treating the path literally and not interpreting any special characters.
Use case 4: Move multiple files into an existing directory, keeping the filenames unchanged
Code:
Move-Item path\to\source1 , path\to\source2 ... path\to\existing_directory
Motivation: This use case is useful when you want to move multiple files into an existing directory, without changing the filenames.
Explanation:
path\to\source1 , path\to\source2
: These arguments represent the paths to the files you want to move.path\to\existing_directory
: This argument represents the path to the existing directory where you want to move the files.
Example output: The files specified in the source paths will be moved to the existing directory specified in the target path, keeping the filenames unchanged.
Use case 5: Move or rename registry key(s)
Code:
Move-Item path\to\source_key1 , path\to\source_key2 ... path\to\new_or_existing_key
Motivation: This use case is useful when you want to move or rename registry key(s) in the Windows Registry.
Explanation:
path\to\source_key1 , path\to\source_key2
: These arguments represent the paths to the registry keys you want to move or rename.path\to\new_or_existing_key
: This argument represents the new or existing path of the registry key.
Example output: The registry keys specified in the source paths will be moved to the new or existing path specified in the target path.
Use case 6: Do not prompt for confirmation before overwriting existing files or registry keys
Code:
mv -Force path\to\source path\to\target
Motivation: This use case is useful when you want to overwrite existing files or registry keys without being prompted for confirmation.
Explanation:
-Force
: This parameter forces the command to overwrite existing files or registry keys without prompting for confirmation.path\to\source
: This argument represents the path to the source file or registry key.path\to\target
: This argument represents the path to the target file or registry key.
Example output: The source file or registry key will be moved or renamed to the target path without prompting for confirmation, even if it overwrites an existing file or registry key.
Use case 7: Prompt for confirmation before overwriting existing files, regardless of file permissions
Code:
mv -Confirm path\to\source path\to\target
Motivation: This use case is useful when you want to be prompted for confirmation before overwriting existing files, regardless of file permissions.
Explanation:
-Confirm
: This parameter prompts for confirmation before overwriting existing files, regardless of file permissions.path\to\source
: This argument represents the path to the source file.path\to\target
: This argument represents the path to the target file.
Example output: You will be prompted for confirmation before overwriting the existing file, regardless of file permissions.
Use case 8: Move files in dry-run mode, showing files and directories which could be moved without executing them
Code:
mv -WhatIf path\to\source path\to\target
Motivation: This use case is useful when you want to preview the files and directories that could be moved without actually executing the command.
Explanation:
-WhatIf
: This parameter shows what would happen if the command is executed, without actually executing the command.path\to\source
: This argument represents the path to the source file or directory.path\to\target
: This argument represents the path to the target file or directory.
Example output: The command will display a preview of the files and directories that could be moved without executing the command. It will show what would happen if the command is executed.