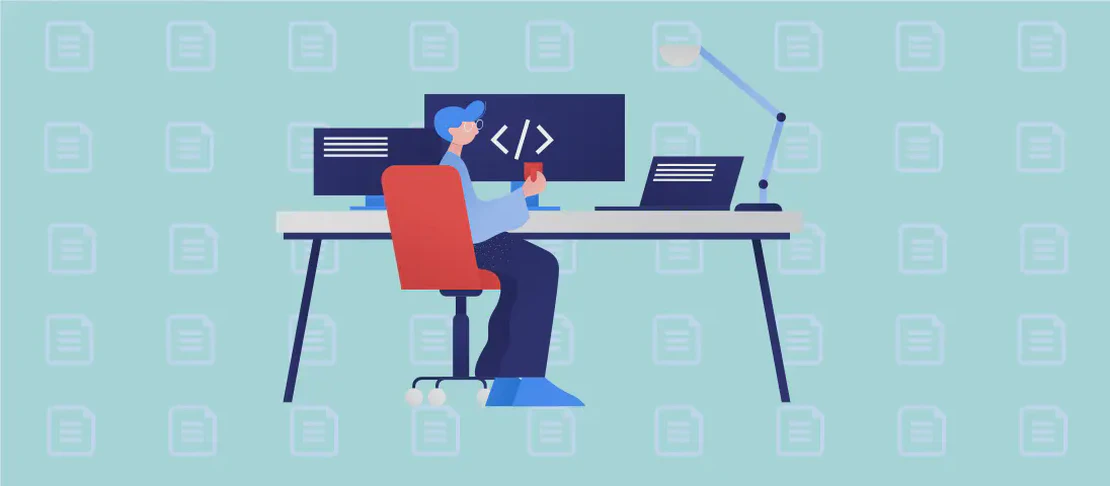
How to Use the `mpicc` Command (with Examples)
- Linux
- December 17, 2024
The mpicc
command is a widely used compiler for parallel programming that leverages the Message Passing Interface (MPI) standard. It serves as a C wrapper compiler for Open MPI applications, seamlessly integrating the necessary compiler and linker flags while employing an underlying C compiler. mpicc
simplifies the process of compiling and linking MPI programs by handling many details automatically, allowing developers to focus on coding their distributed applications. This is particularly useful in clusters and high-performance computing environments where parallel processing capabilities are critical.
Use Case 1: Compile a Source Code File into an Object File
Code:
mpicc -c path/to/file.c
Motivation:
When working on a large-scale parallel computing project, developers often split their code into multiple modules for better organization and maintainability. Compiling each module or source code file into an object file separately not only speeds up subsequent compilation steps when changes are made to the code but also helps in debugging and isolating errors in specific parts of the code. By creating object files first, developers can link them all together at a later stage to form the complete executable program.
Explanation:
mpicc
: Invokes the MPI C wrapper compiler to handle the compilation process, ensuring that all necessary MPI compiler and linker flags are included.-c
: Instructsmpicc
to compile the source file into an object file without linking. This step generates an intermediary file with a.o
extension, which is essential for creating the final executable later.path/to/file.c
: Specifies the path to the C source file that you want to compile. This file contains the code that utilizes MPI functions and calls necessary for parallel computation.
Example Output:
After executing the command, you’ll observe an object file with the .o
extension in the specified directory. This object file can then be used for linking in subsequent stages of the build process. The exact naming will resemble the source file name but with a .o
suffix (e.g., file.o
).
Use Case 2: Link an Object File and Make an Executable
Code:
mpicc -o executable path/to/object_file.o
Motivation:
Once you’ve established that individual modules or parts of your program compile correctly, the next logical step is to link them together to form an executable application. This stage combines the compiled object files into a single executable that can be run on a cluster or any distributed computing environment, leveraging multiple processors to perform tasks in parallel. Linking is crucial as it resolves function calls and symbols across different object files, creating a cohesive application.
Explanation:
mpicc
: Again, the MPI C wrapper compiler manages the linking process, ensuring all necessary paths and flags are included for MPI functionality.-o
: This option specifies the output file, allowing you to define the name of the executable being created. It helps you manage multiple versions or iterations of your application.executable
: The desired name for the output executable file. This is the file you will execute to run your MPI-based application.path/to/object_file.o
: The path to the object file produced in the previous step, serving as an input for the linking process. This object file must have been compiled with MPI in mind to ensure compatibility.
Example Output:
Executing this command generates an executable file with the specified name in your directory. This file is now ready to be run using appropriate MPI runtime commands, like mpirun
or mpiexec
, on your computing cluster.
Use Case 3: Compile and Link Source Code in a Single Command
Code:
mpicc -o executable path/to/file.c
Motivation:
For smaller projects or during the initial stages of development, developers might prefer to streamline their workflow by compiling and linking in a single step. This method is efficient for quick tests, prototyping new ideas, or in situations where there’s little need to separate compilation and linking due to the simplicity of the project. By combining these steps, one can produce an executable directly from the source code without intermediate files, which is often suitable for smaller programs or quick iterations.
Explanation:
mpicc
: Utilizes the MPI C wrapper to handle both compilation and linking, ensuring that MPI flags are properly managed.-o
: Specifies the output file name, as with linking, allowing for easy identification and management of the resulting executable.executable
: The target name for the resulting executable file, ready for execution.path/to/file.c
: Path to the source code file that requires compiling and linking, reducing multiple commands to a single, efficient command line input.
Example Output:
Upon successful execution, the command yields an executable in the directory, akin to the previous linking process. This executable combines both the compilation and linking stages, eliminating the need for separate object files.
Conclusion:
The mpicc
command is a powerful utility for handling the compilation and linking processes necessary to create high-performance, parallel applications using MPI. Through its flexible command-line options, developers can optimize their workflow, whether they’re working with modular codebases or rapid prototyping efforts. By mastering these use cases, one can efficiently manage the complexities of MPI programming, ultimately leading to robust and scalable distributed applications.