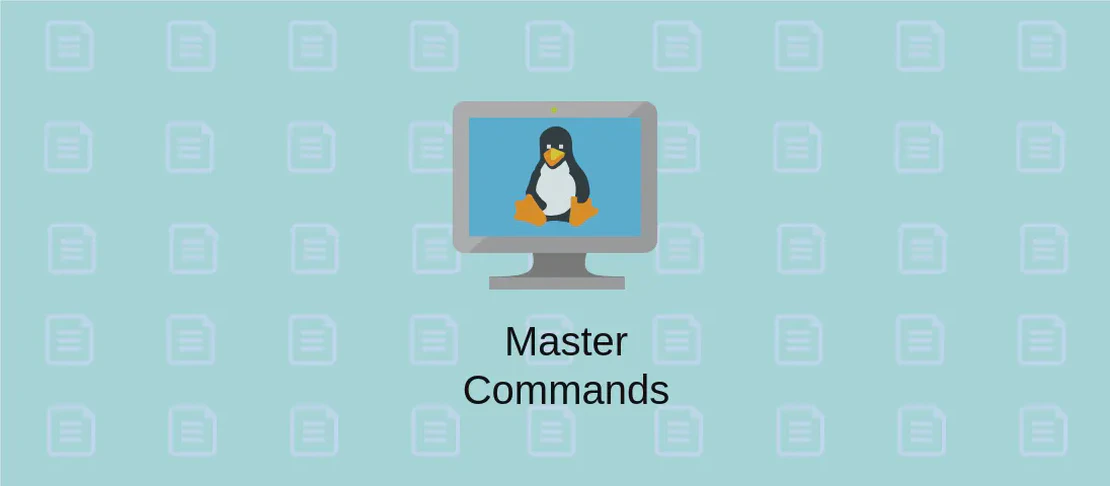
Mastering Apache Maven Commands (with examples)
Apache Maven is a powerful build automation tool primarily used for Java projects. It simplifies the build process, dependency management, and project configuration, making it easier for developers to handle complex system builds. Maven leverages the concept of a project object model (POM), which defines the structure and dependency management for your project in an XML file. Whether you’re compiling, testing, or packaging a project, Maven’s streamlined commands facilitate efficient project handling in various development stages.
Compile a project
Code:
mvn compile
Motivation:
Compiling is a crucial step in building any Java-based project because it transforms human-readable Java code into bytecode that the Java Virtual Machine (JVM) can execute. Running mvn compile
allows developers to ensure their source code is syntactically correct and ready for execution, and it provides early detection of errors in code by identifying syntax issues and other compile-time errors swiftly.
Explanation:
mvn
: Invokes the Maven command.compile
: This is a lifecycle phase in Maven that takes your project’s.java
files and compiles them into.class
files, placing them in thetarget/classes
directory according to the convention.
Example Output:
[INFO] Compiling 10 source files to /path/to/project/target/classes
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Compile and package the compiled code in its distributable format, such as a jar
Code:
mvn package
Motivation:
Once you have successfully compiled the code, the next logical step is creating a distributable format of your application, such as a Java Archive (jar
) file. This step is vital for deployment, as it bundles all the compiled code, resources, and necessary metadata into a single archive, simplifying distribution and execution.
Explanation:
mvn
: Initiates Maven.package
: This phase compiles the code, processes the resources, and archives the outcome, producing a.jar
or.war
file, depending on the project’s packaging configuration in the POM file.
Example Output:
[INFO] Building jar: /path/to/project/target/project-name.jar
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Compile and package, skipping unit tests
Code:
mvn package -DskipTests
Motivation:
Time constraints often necessitate faster build times. If a build is regularly running unit tests during the package phase, skipping them can save considerable time. This command is particularly useful during development or when you’re certain your code hasn’t changed in ways that affect functionality already covered by tests.
Explanation:
mvn
: Executes the Maven command.package
: Creates the distributable format.-DskipTests
: This argument tells Maven to skip any tests that would typically be executed. It effectively disables the testing phase, so testing reports will not be generated, and test results will not impact build success.
Example Output:
[INFO] Tests are skipped.
[INFO] Building jar: /path/to/project/target/project-name.jar
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Install the built package in a local Maven repository
Code:
mvn install
Motivation:
After building and packaging your project, utilizing it across multiple projects often requires installation into the local Maven repository. The install
phase takes the project’s output and dependencies and copies them into your local repository, making it readily available for other projects. This is especially useful for reusable libraries or modules.
Explanation:
mvn
: Calls Maven to action.install
: First runs thecompile
,test
, andpackage
phases, then places the resultant artifact(s) into the local repository defined in the Maven configuration (.m2/repository
by default).
Example Output:
[INFO] Installing /path/to/project/target/project-name.jar to /home/username/.m2/repository/com/example/project-name/1.0/project-name-1.0.jar
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Delete build artifacts from the target directory
Code:
mvn clean
Motivation:
To maintain a clean working directory and avoid potential issues from stale build artifacts, you may need to delete previous versions of compiled code and packaged files. mvn clean
is useful when you want to ensure that from one build to the next, no remnants of previous builds could affect current ones, thereby ensuring a fresh build environment.
Explanation:
mvn
: Launches the Maven build tool.clean
: Removes thetarget
directory, where compiled code and temporary files reside, ensuring the next build starts with no residual data from previous builds.
Example Output:
[INFO] Deleting /path/to/project/target
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Do a clean and then invoke the package phase
Code:
mvn clean package
Motivation:
Combining the clean
and package
phases results in a fresh build. This command is typically used when integrating changes and seeking a complete rebuild of the distributable artifact ensures the application package is freshly created without any previous artifacts that might otherwise exist from past build attempts.
Explanation:
mvn
: Initiates Maven operations.clean
: Deletes thetarget
directory to ensure compiled classes, test results, and packaged artifacts aren’t stale.package
: After cleaning, recompiles code, processes resources, and packages the output into a .jar or .war as required.
Example Output:
[INFO] Deleting /path/to/project/target
[INFO] Compiling 10 source files to /path/to/project/target/classes
[INFO] Building jar: /path/to/project/target/project-name.jar
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Clean and then package the code with a given build profile
Code:
mvn clean -P profile package
Motivation:
Sometimes, projects require different configurations (like database connections or API keys) based on the environment they are built for (development, testing, production). Maven profiles are extraordinarily useful for building a project in varying contexts. By specifying a profile, you can adapt your build to distinct needs without changing source code or configuration files.
Explanation:
mvn
: Deploys Maven.clean
: Erases existing build artifacts for a fresh start.-P profile
: Activates the specified profile from your POM file, which could adjust settings, dependencies, and directories according to the environment or requirements of the deployment.package
: Completes the cleanly started build by packaging it according to the specifics of the activated profile.
Example Output:
[INFO] Deleting /path/to/project/target
[INFO] Building jar: /path/to/project/target/project-name.jar
[INFO] Activated profiles: profile
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Run a class with a main method
Code:
mvn exec:java -Dexec.mainClass="com.example.Main" -Dexec.args="argument1 argument2 ..."
Motivation:
Developers often need to test or demonstrate parts of their application quickly. Running a specific main class directly via Maven without extensive configuration changes allows for quick execution and testing of applications with command-line arguments. This method helps streamline rapid prototyping and debugging.
Explanation:
mvn
: Executes Maven commands.exec:java
: Utilizes the Exec Maven Plugin to execute a Java program within the project’s context.-Dexec.mainClass="com.example.Main"
: Specifies the fully qualified name of the Java class containing themain
method you wish to run.-Dexec.args="argument1 argument2 ..."
: Provides necessary arguments to the main method (if any), simulating complete command-line invocation from within Maven.
Example Output:
[INFO] Java application com.example.Main started with arguments [argument1, argument2]
[INFO] Output from the application
[INFO] Java application com.example.Main finished successfully
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Conclusion:
Apache Maven is an essential tool for building and managing Java-based projects. Through its diverse set of streamlined commands, it facilitates numerous development tasks ranging from compilation to deployment. Mastering its commands not only enhances productivity but also improves the overall quality of the build process by leveraging its robust command execution framework
. By understanding and utilizing commands such as compile
, package
, clean
, and more, developers can maintain efficient, clean, and consistent build processes across various stages of development and deployment.