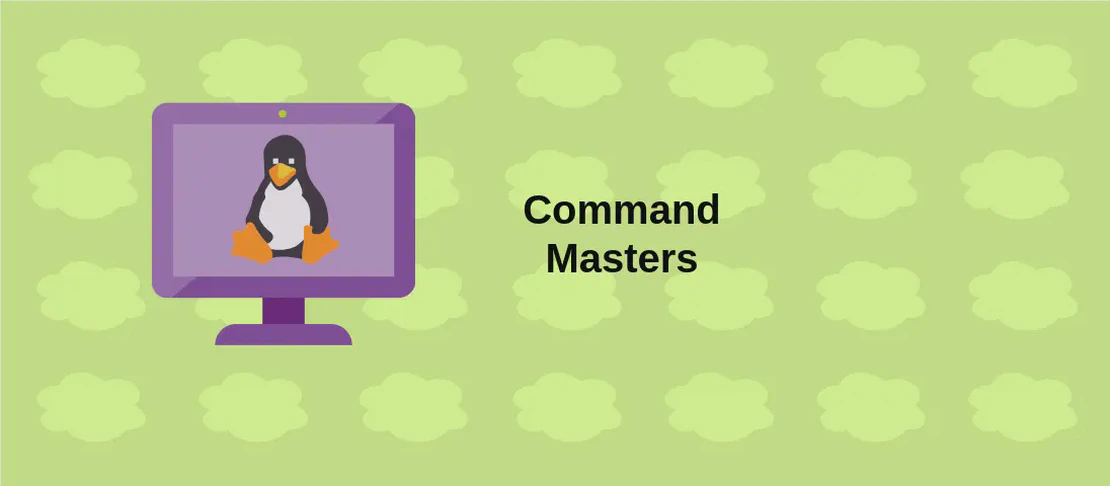
How to use the command 'mypy' (with examples)
Mypy is a static type checker for Python programming language. It is used to add optional type annotations to Python programs, which allows developers to catch type-related errors during the development process rather than at runtime. This tool provides a way to ensure that your code adheres to the type constraints you’ve specified, making your code more predictable and less error-prone.
Type check a specific file
Code:
mypy path/to/file.py
Motivation:
When working with a large codebase, focusing on one file at a time can help streamline the debugging process. Type-checking a specific file using Mypy is vital for isolating and identifying type-related issues within that segment of the code. This step is particularly helpful when you’re making changes to an individual file and want immediate feedback to ensure type correctness.
Explanation:
mypy
: This is the command to invoke the Mypy tool.path/to/file.py
: This specifies the path to the specific Python file you want to type check. Mypy will process only this file and report any detected type mismatches according to the annotations provided in the code.
Example output:
path/to/file.py:10: error: Incompatible types in assignment (expression has type "int", variable has type "str")
Found 1 error in 1 file (checked 1 source file)
Type check a specific module
Code:
mypy -m module_name
Motivation:
Applications are often structured into modules, each providing specific functionalities. Running Mypy on a module helps ensure all parts of the module adhere to type specifications. This practice is beneficial when you want to ensure consistency and integrity across an entire module rather than manually checking each file.
Explanation:
mypy
: The command to run Mypy.-m module_name
: This flag indicates that you are type checking a module. Themodule_name
is the module’s name you wish to check, which Mypy will locate and examine all the relevant code in that module.
Example output:
module_name/submodule.py:20: error: Argument 1 to "process_data" has incompatible type "List[int]"; expected "List[str]"
Found 1 error in 1 file (checked 3 source files)
Type check a specific package
Code:
mypy -p package_name
Motivation:
For larger projects that are organized into packages, checking an entire package ensures that all its modules and submodules adhere to the specified type annotations. This approach provides a comprehensive check to catch any discrepancies that might arise from interactions across multiple modules within a package.
Explanation:
mypy
: This is the command to initiate Mypy.-p package_name
: Using the-p
flag, Mypy will check all modules within the specified package. This is useful for ensuring that the package is type-consistent throughout its entire structure.
Example output:
package_name/module1.py:15: error: Incompatible types in assignment (expression has type "None", variable has type "int")
package_name/module2.py:8: error: Value of type "Optional[str]" is not indexable
Found 2 errors in 2 files (checked 8 source files)
Type check a string of code
Code:
mypy -c "code"
Motivation:
During the development or debugging processes, you might need to quickly verify type-checking rules for a small code snippet. Using Mypy to type check code fragments helps developers test hypotheses or understand how certain types interact within Python code.
Explanation:
mypy
: Invokes the Mypy type checker.-c "code"
: This flag allows you to provide code directly as a string argument. Mypy will type check this code snippet as if it were a standalone file.
Example output:
error: Incompatible types in assignment (expression has type "int", variable has type "str")
Found 1 error in 1 file (checked 1 source file)
Ignore missing imports
Code:
mypy --ignore-missing-imports path/to/file_or_directory
Motivation:
During the development process, you may come across scenarios where certain modules are not installed, or their types are not easily importable. Using Mypy’s --ignore-missing-imports
flag allows you to proceed with type checking while bypassing unresolved import issues, making it a practical solution when dealing with many optional dependencies.
Explanation:
mypy
: The tool being used to perform the type checking.--ignore-missing-imports
: This flag tells Mypy to ignore any missing module imports. It’s useful when working with external libraries whose stubs might not be available.path/to/file_or_directory
: This specifies the path to the file or directory you want Mypy to check while ignoring any import errors.
Example output:
path/to/file.py:5: error: Unsupported operand types for + ("str" and "int")
Found 1 error in 1 file (checked 1 source file)
Show detailed error messages
Code:
mypy --show-traceback path/to/file_or_directory
Motivation:
For complex projects, errors encountered during type checking might not be sufficiently explanatory. Showing the traceback gives developers deeper insights into the nature of the errors encountered, thereby facilitating a better understanding and quicker resolution.
Explanation:
mypy
: Command used for running the Mypy tool.--show-traceback
: Flag that tells Mypy to display detailed traceback information for any errors encountered. This helps in debugging complex issues by providing context about the error’s origin.path/to/file_or_directory
: The location of the file or directory you want to type-check while showing detailed error messages.
Example output:
path/to/file.py:17: error: Incompatible return value type (got "int", expected "str")
Traceback (most recent call last):
File "path/to/file.py", line 17, in <module>
result = some_function()
TypeError: Incompatible types
Found 1 error in 1 file (checked 1 source file)
Specify a custom configuration file
Code:
mypy --config-file path/to/config_file
Motivation:
In sizable projects, nuanced configurations are often necessary to adjust type-checking behavior according to project requirements. Utilizing a configuration file speeds up this process, allowing developers to maintain these settings in a structured and manageable way.
Explanation:
mypy
: Command line program for type checking.--config-file path/to/config_file
: This flag specifies a custom configuration file that Mypy should use to retrieve settings, ensuring a tailored type-checking process.
Example output:
Success: no issues found in 10 source files
Display help
Code:
mypy -h
Motivation:
The help command provides users with a quick summary of the command-line options available with Mypy. This functionality is crucial, particularly for new users, to understand how to efficiently utilize all features offered by the tool.
Explanation:
mypy
: The base command for the type-checking tool.-h
: A help flag that displays usage information for themypy
command, summarizing all the options you can use with it.
Example output:
Usage: mypy [options] [files or packages]
Options:
-m MODULE, --module MODULE
Type-check module; can repeat for more modules
-p PACKAGE, --package PACKAGE
Type-check package recursively
--ignore-missing-imports
Suppress error about imports that cannot be resolved
...
Conclusion:
Mypy is a powerful static type checker in the Python ecosystem. As demonstrated, it offers various command options tailored for specific use cases, from checking individual files to entire packages and modules. Type annotations and type checking improve code stability and maintainability by catching potential errors early in the development process. Through its flexibility in configuration and ease of use, Mypy integrates seamlessly into a developer’s workflow, enhancing code quality significantly.