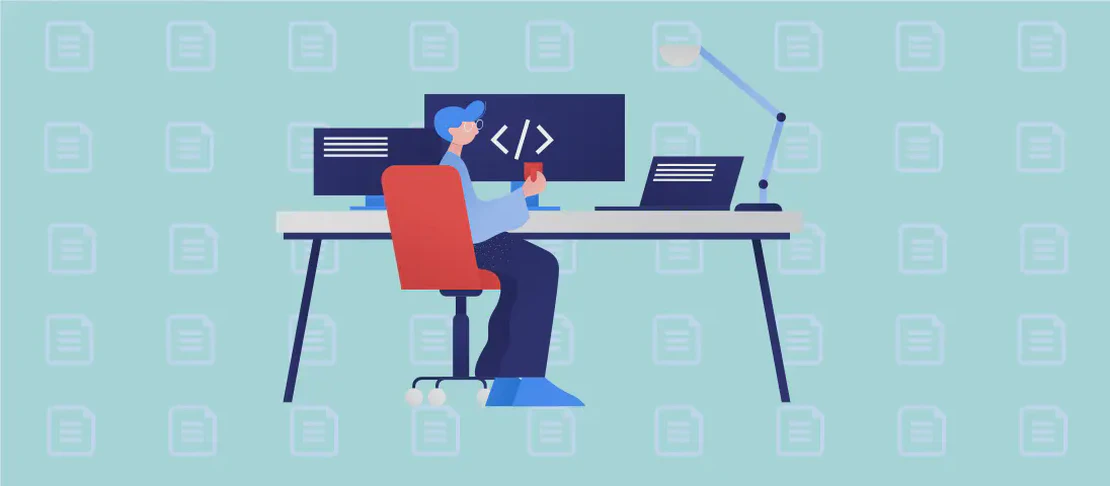
How to Use the NASM Command (with Examples)
The Netwide Assembler, commonly known as NASM, is a versatile assembler tailored for the Intel x86 architecture. It is a powerful tool for developers working with low-level programming and is widely used for creating applications that require direct hardware interaction, such as operating systems and high-performance software. NASM is prized not only for its portability across different systems but also for its support of a wide range of output file formats. Its extensive capabilities are balanced with ease of use, making it a staple in the toolbox of many systems programmers.
Use Case 1: Assemble source.asm
into a Binary File source
, in the Default Raw Binary Format
Code:
nasm source.asm
Motivation:
The primary motivation for assembling a .asm
file using NASM is to translate human-readable assembly language code into machine code, which the computer can execute directly. This process is essential in low-level programming tasks such as developing operating systems or embedded systems where direct hardware manipulation is necessary.
Explanation:
nasm
: This is the command that invokes the Netwide Assembler.source.asm
: This is the input file, containing the assembly language code that you want to assemble. The.asm
extension denotes that the file is written in assembly language.
Example Output:
When this command is executed successfully, NASM will produce a binary file named source
in the raw binary format. This file is a direct translation of the assembly instructions in source.asm
into machine code, which can be executed by the processor.
Use Case 2: Assemble source.asm
into a Binary File output_file
, in the Specified Format
Code:
nasm -f format source.asm -o output_file
Motivation:
This use case is particularly useful when you need the machine code output in a specific format compatible with other tools or operating systems. Different formats such as ELF, COFF, or Mach-O might be required depending on the target environment or for further processing in build systems.
Explanation:
-f format
: Specifies the output format. NASM supports several output formats, such as ELF, COFF, and Mach-O. This argument tells NASM to format the assembled binary in a particular way.source.asm
: The input assembly file to be processed.-o output_file
: Sets the name of the output file tooutput_file
. Without this, the default output name would be derived from the input file with a format-specific extension.
Example Output:
The command results in the creation of output_file
in the specified format, containing the compiled machine code. The output file will be suitably structured to be compatible with other software tools, aiding in further stages of development or integration.
Use Case 3: List Valid Output Formats (along with Basic NASM Help)
Code:
nasm -hf
Motivation:
Before starting an assembly project, it can be helpful to know the output formats NASM supports to ensure compatibility with the rest of your toolchain or the requirements of your project. Listing these formats helps in planning and configuring your build process correctly from the outset.
Explanation:
-hf
: This option requests NASM to display help information, focusing particularly on listing all valid output formats that NASM can generate, alongside general help hints for using NASM.
Example Output:
Executing this command results in a detailed display of available output formats, such as ELF, PE, and Mach-O, among others, as well as basic help on NASM usage. This output informs users about possible options for their assembler targets and provides guidance on general NASM usage.
Use Case 4: Assemble and Generate an Assembly Listing File
Code:
nasm -l list_file source.asm
Motivation:
While developing in assembly language, visibility into how code translates to machine instructions can be incredibly insightful. An assembly listing file provides a mapping between assembly code and its machine language equivalent, serving as a vital tool in debugging and understanding program behavior.
Explanation:
-l list_file
: Instructs NASM to create a listing file namedlist_file
. This file contains a detailed line-by-line translation of assembly code into machine code, with offsets and other pertinent data.source.asm
: The source assembly file to be converted into machine code and while producing an associated listing.
Example Output:
Running this command generates list_file
, a valuable artifact in development that can be referenced to understand binary representation of code, troubleshoot issues, or optimize assembly code by observing instruction translations and addresses.
Use Case 5: Add a Directory to the Include File Search Path Before Assembling
Code:
nasm -i path/to/include_dir/ source.asm
Motivation:
In complex projects, separating common routines or constants into include files improves maintainability and reusability. However, NASM must know where to look for these included files. Specifying additional directories expands NASM’s search path, ensuring all pertinent files are located during assembly.
Explanation:
-i path/to/include_dir/
: This argument tells NASM to addpath/to/include_dir/
to the list of directories it will search when looking for files included in the source code. It must end with a slash to be recognized properly by NASM.source.asm
: The main assembly file to assemble, which may containinclude
directives pointing to files within the specified directory.
Example Output:
After executing this command, NASM will include files located within path/to/include_dir/
when assembling source.asm
, allowing shared code and resources to be easily incorporated and compiled along with the main program.
Conclusion:
NASM proves to be a robust tool in the realm of low-level programming, catering to various needs through its flexible command-line interface. Whether you need a simple raw binary, a format-specific output, or comprehensive insight through listing files, NASM provides the functionality required for efficient and effective assembly programming. Understanding these use cases unlocks the full potential of NASM, empowering developers to undertake a wide range of projects with confidence and precision.