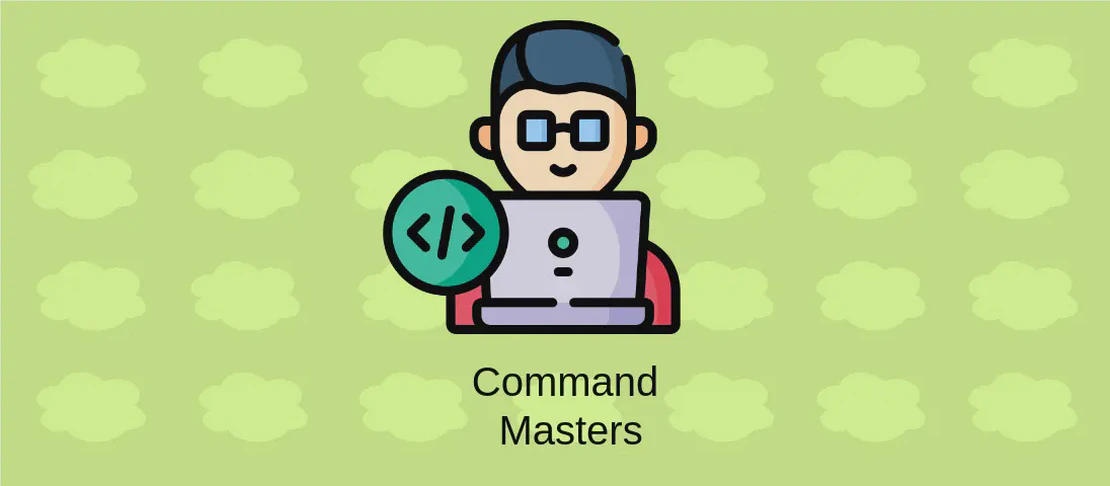
How to use the command "ncc" (with examples)
“ncc” is a command-line tool that allows you to compile a Node.js application into a single file. It supports TypeScript, binary addons, and dynamic requires, making it a powerful tool for bundling your Node.js applications.
Use case 1: Bundle a Node.js application
Code:
ncc build path/to/file.js
Motivation: In this use case, you can use the “ncc” command to bundle a Node.js application located at a specific path into a single file. This can be useful when you want to distribute your application as a single file, making it easier to deploy and share with others.
Explanation:
build
: This argument tells the “ncc” command to bundle the Node.js application.path/to/file.js
: This specifies the path to the Node.js application file you want to bundle.
Example output: The “ncc” command will bundle the Node.js application located at “path/to/file.js” into a single file.
Use case 2: Bundle and minify a Node.js application
Code:
ncc build --minify path/to/file.js
Motivation: In certain cases, you may want to minimize the size of your bundled Node.js application to improve performance and reduce bandwidth usage. By using the “–minify” option with the “ncc” command, you can achieve this.
Explanation:
--minify
: This flag tells the “ncc” command to minify the bundled Node.js application.
Example output: The “ncc” command will bundle and minify the Node.js application located at “path/to/file.js”, resulting in a smaller-sized output file.
Use case 3: Bundle and minify a Node.js application and generate source maps
Code:
ncc build --source-map path/to/file.js
Motivation: When developing a Node.js application, having source maps can greatly simplify the debugging process. By using the “–source-map” option with the “ncc” command, you can generate source maps alongside the bundled and minified application.
Explanation:
--source-map
: This flag tells the “ncc” command to generate source maps for the bundled Node.js application.
Example output: The “ncc” command will bundle and minify the Node.js application located at “path/to/file.js” and generate corresponding source maps in the output.
Use case 4: Automatically recompile on changes to source files
Code:
ncc build --watch path/to/file.js
Motivation: When actively developing a Node.js application, it can be time-consuming to manually recompile the code each time you make changes. By using the “–watch” option with the “ncc” command, it will automatically recompile the application whenever changes are detected in the source files.
Explanation:
--watch
: This flag tells the “ncc” command to monitor the source files for changes and recompile the application when modifications occur.
Example output: The “ncc” command will continuously monitor the source files of the Node.js application located at “path/to/file.js” and automatically recompile it whenever changes are made.
Use case 5: Bundle a Node.js application into a temporary directory and run it for testing
Code:
ncc run path/to/file.js
Motivation: During the development process, it is common to run and test a Node.js application multiple times. By using the “ncc” command with the “run” subcommand, you can bundle the application into a temporary directory and directly run it for testing purposes.
Explanation:
run
: This subcommand of the “ncc” command tells it to bundle the Node.js application into a temporary directory and run it.path/to/file.js
: This specifies the path to the Node.js application file.
Example output: The “ncc” command will bundle the Node.js application located at “path/to/file.js” into a temporary directory and execute it for testing purposes.
Use case 6: Clean the “ncc” cache
Code:
ncc clean cache
Motivation: Over time, the “ncc” command may cache certain files to speed up subsequent builds. However, there might be cases where you want to clear this cache to ensure a clean build. By running the command with the “clean” subcommand and “cache” argument, you can delete the “ncc” cache.
Explanation:
clean
: This subcommand of the “ncc” command tells it to clean a specific aspect of its functionality.cache
: This argument specifies that the cache should be cleaned.
Example output: The “ncc” command will delete the cache stored by “ncc”, ensuring a fresh build on the next run.
Conclusion:
In this article, we explored various use cases of the “ncc” command. We learned how to bundle Node.js applications, minimize their size, generate source maps, automatically recompile on file changes, run applications for testing, and clean the “ncc” cache. By utilizing these examples, you can efficiently manage and optimize your Node.js applications.