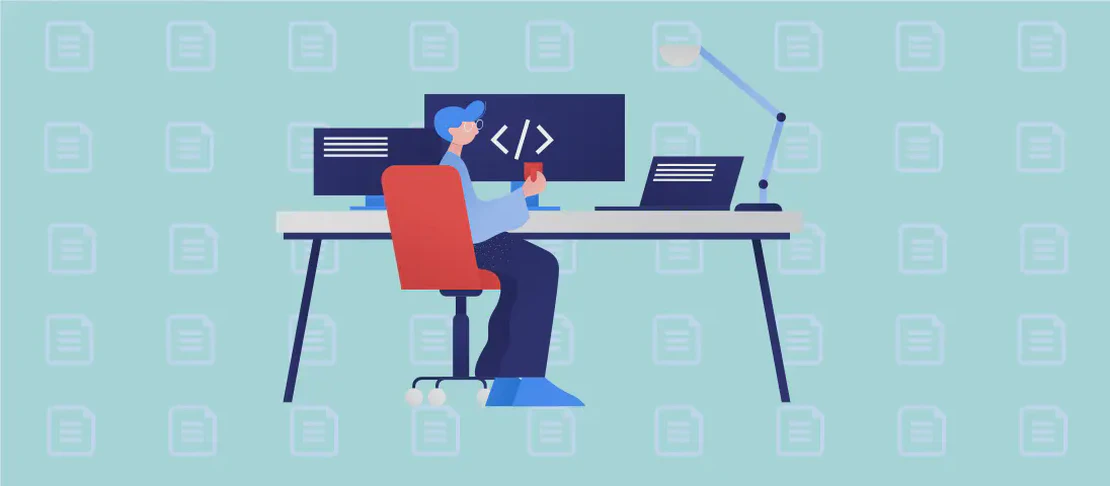
Understanding the 'ncu' Command for Managing npm Packages (with examples)
The ncu
command, short for “npm-check-updates,” is a powerful tool for developers who want to manage their npm package dependencies effectively. It simplifies the process of keeping your Node.js projects up-to-date by finding newer versions of package dependencies and determining outdated npm packages, both locally and globally. While ncu
is adept at checking for updates, it does not install them directly; it merely updates version numbers in package.json
. To apply these updates, a subsequent run of npm install
is necessary. This command is essential for developers aiming to ensure their projects are using the latest packages, which often lead to improved performance, security, and new features.
Use case 1: List outdated dependencies in the current directory
Code:
ncu
Motivation:
Running ncu
in the current directory allows developers to quickly audit which packages in their project have newer versions available. Keeping dependencies updated is crucial for leveraging the latest improvements and security patches offered by package maintainers. By listing outdated packages, developers can maintain high standards for code quality and security without manually checking each package.
Explanation:
- The command
ncu
with no additional arguments scopes the operation to the current project directory. It inspects thepackage.json
file for outdated dependencies and compares them to the latest available versions in the npm registry.
Example output:
Checking /path/to/project/package.json
[====================] 7/7 100%
express 4.17.1 → 4.18.0
lodash 4.17.15 → 4.17.21
react 16.13.0 → 17.0.2
Run `ncu -u` to upgrade package.json
Use case 2: List outdated global npm
packages
Code:
ncu --global
Motivation:
Over time, developers accumulate global npm packages that might become outdated. These packages might be CLI tools or utilities required globally across various projects. Keeping them updated ensures that new features and bug fixes are available, enhancing the development environment.
Explanation:
- The
--global
argument alters the scope from the local project directory to globally installed npm packages. This way,ncu
inspects and reports on any outdated global dependencies.
Example output:
Checking global packages
[====================] 5/5 100%
npm 6.14.8 → 7.5.3
nodemon 2.0.2 → 2.0.7
Run `npm install -g [package]` to update globally installed packages
Use case 3: Upgrade all dependencies in the current directory
Code:
ncu --upgrade
Motivation:
Automatically upgrading all packages in one go can save time for developers who need to quickly move their project forward with the latest updates. This can be particularly beneficial in the early stages of a new project or when preparing to refactor a project to use the latest technologies.
Explanation:
- The
--upgrade
flag instructsncu
to not only list but also update thepackage.json
file with the latest version numbers of all outdated dependencies. Note that this does not install the packages; it merely updates the version numbers in the configuration file.
Example output:
Upgrading /path/to/project/package.json
[====================] 7/7 100%
express 4.17.1 → 4.18.0
lodash 4.17.15 → 4.17.21
react 16.13.0 → 17.0.2
Run `npm install` to update your local packages
Use case 4: Interactively upgrade dependencies in the current directory
Code:
ncu --interactive
Motivation:
For larger projects with many dependencies, a developer might want to selectively upgrade packages that are most pertinent or beneficial to them at the moment rather than upgrading everything all at once. An interactive mode provides a hands-on approach to managing your updates, allowing for informed decisions.
Explanation:
- The
--interactive
flag initiates an interactive mode where the developer is prompted to decide for each package whether or not to update it. This interactive selection process gives more control over the upgrade process.
Example output:
Checking /path/to/project/package.json
[====================] 7/7 100%
express 4.17.1 → 4.18.0
Update this package? (Use arrow keys)
> Yes
No
Next
Use case 5: List outdated dependencies up to the highest minor version
Code:
ncu --target minor
Motivation:
When developing projects that rely on semantic versioning, it is often sufficient to upgrade to the latest minor version rather than the latest major version. This helps maintain backward compatibility while receiving minor improvements and bug fixes.
Explanation:
- The
--target minor
flag tellsncu
to limit the search to the latest minor versions for each package. This means it will find updates that do not introduce potential breaking changes associated with major version changes.
Example output:
Checking /path/to/project/package.json
[====================] 7/7 100%
express 4.17.1 → 4.17.2
lodash 4.17.15 → 4.17.21
No major version upgrades found
Use case 6: List outdated dependencies that match a keyword or regular expression
Code:
ncu --filter keyword|/regex/
Motivation:
In projects where only certain packages (by name or pattern) need to be checked for updates, filtering can focus the update check on only those dependencies. This is particularly useful for large codebases where updating specific dependencies might be planned or required for current tasks.
Explanation:
- The
--filter
flag allows specifying a keyword or a regular expression pattern to filter the dependencies. Only those matching the pattern will be considered for updates, providing a precise scope for the upgrade check.
Example output:
Checking /path/to/project/package.json
[====================] 7/7 100%
lodash 4.17.15 → 4.17.21
No other matches for "lodash"
Use case 7: List only a specific section of outdated dependencies
Code:
ncu --dep dev|optional|peer|prod|packageManager
Motivation:
Different types of dependencies serve varying roles in a project. A developer might only wish to update certain types, such as development or production dependencies, depending on the current phase of their work. This option allows for updates that are more context-sensitive.
Explanation:
- The
--dep
flag specifies which section of dependencies (development, optional, peer, production, or package manager) are to be checked for updates. This granularity provides more control over dependency management processes.
Example output:
Checking devDependencies of /path/to/project/package.json
[====================] 3/3 100%
mocha 8.0.1 → 8.2.1
eslint 7.0.0 → 7.5.0
Use case 8: Display help
Code:
ncu --help
Motivation:
As with any complex command-line tool, ncu
has a variety of flags and options that can be difficult to remember. Displaying the help information provides a quick reference for correct usage and available arguments, ensuring the command is used to its fullest potential.
Explanation:
- The
--help
argument summons the help documentation for thencu
command, detailing syntax, options, flags, and examples that assist the user in mastering its functionality.
Example output:
Usage: ncu [options]
Options:
-h, --help output usage information
-g, --global check global packages instead of in the current project
-f, --filter <pattern> filter packages by name or regular expression
--upgrade upgrade package definitions in package.json to latest versions
--interactive enable interactive prompts for each dependency
--dep <section> update only specified section (dev, optional, peer, prod, or packageManager)
...
Conclusion:
The ncu
command stands out as an invaluable tool for developers managing npm packages. Its versatility is showcased through its multiple options, enabling users to efficiently keep project dependencies updated. By offering functionalities from simply listing outdated dependencies to interactively upgrading them, ncu
greatly enhances a developer’s ability to maintain and improve their project setups with ease.