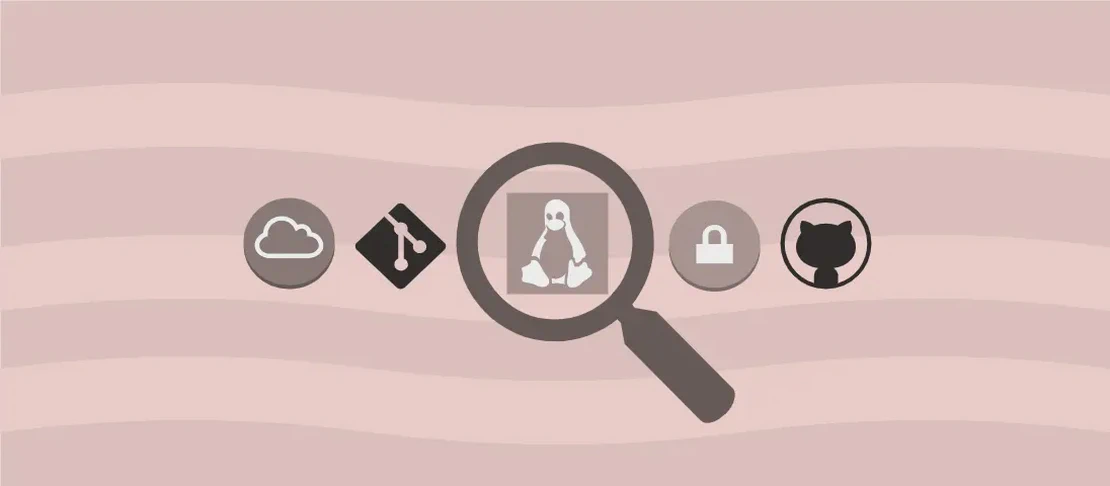
How to Use the Command 'New-Item' in PowerShell (with examples)
- Windows
- December 17, 2024
The New-Item
command in PowerShell is a versatile tool used to create new files, directories, symbolic links, hard links, junctions, or registry entries. It offers a straightforward way to manage file systems and the registry in a Windows environment. By utilizing New-Item
, users can automate tasks related to directory management, file creation, and registry manipulation. Here, we explore various use cases of the New-Item
command, each illustrated with examples.
Use Case 1: Create a New Blank File
Code:
New-Item path\to\file
Motivation:
Creating a blank file is a common task during software development, scripting, or for organizational purposes. A new blank file often serves as a placeholder or a configuration file that will later be populated with necessary data.
Explanation:
New-Item
: This is the command used to create a new item.path\to\file
: Specifies the path where the new file will be created. This includes the full path and the filename with extension, if applicable.
Example Output:
When executed, the command creates a file at the specified path. If “path\to\file” resolves correctly, you will see a PowerShell object representing the new file.
Use Case 2: Create a New Directory
Code:
New-Item -ItemType Directory path\to\directory
Motivation:
Organizing files into directories is crucial for maintaining an efficient and navigable filesystem. New directories are often needed when setting up new projects or when reorganizing existing files.
Explanation:
New-Item
: Invokes the command to create a new item in the filesystem.-ItemType Directory
: Specifies that the item to be created is a directory.path\to\directory
: Indicates the path where the new directory will be created.
Example Output:
Upon execution, a new directory is created at the specified location, and its path is outputted as confirmation.
Use Case 3: Write a New Text File with Specified Content
Code:
New-Item path\to\file -Value content
Motivation:
Automatically generating text files with specific content is useful for configuration files, scripts, or documentation that need an initial set of data or instructions.
Explanation:
New-Item
: Command for creating a new item.path\to\file
: Location where the new file will be created.-Value content
: Directly provides the content that will be written into the file.
Example Output:
The command results in a new file at the designated path containing the specified content.
Use Case 4: Write the Same Text File in Multiple Locations
Code:
New-Item path\to\file1 , path\to\file2 , ... -Value content
Motivation:
When deploying multiple instances of a file across various directories, this method efficiently copies specified content into numerous locations simultaneously.
Explanation:
New-Item
: Initiates the creation of new items.path\to\file1 , path\to\file2 , ...
: Lists the file paths where the new files will be created. Each specified path will receive a new file with identical content.-Value content
: Assigns the content to be written into all specified files.
Example Output:
Multiple files are created at the designated paths, each containing the specified content. Confirmation is provided via PowerShell output for each file creation.
Use Case 5: Create a Symbolic Link, Hard Link, or Junction
Code:
New-Item -ItemType SymbolicLink|HardLink|Junction -Path path\to\link_file -Target path\to\source_file_or_directory
Motivation:
Links are powerful tools for referencing or integrating multiple files or directories without duplication. They enable seamless file access and system resource management.
Explanation:
New-Item
: Command to create a new item.-ItemType SymbolicLink|HardLink|Junction
: Defines the type of link to be created—symbolic link, hard link, or junction.-Path path\to\link_file
: Specifies where the link will be created.-Target path\to\source_file_or_directory
: Indicates the source item to which the link will point.
Example Output:
The command generates a link at the specified path, referencing the target file or directory. A PowerShell object confirming the link creation is outputted.
Use Case 6: Create a New Blank Registry Entry
Code:
New-Item path\to\registry_key
Motivation:
Modifying the registry is essential for system customization and software configuration on Windows systems. Creating a blank registry entry allows for additional configuration and settings setup.
Explanation:
New-Item
: Command used to create the new registry key.path\to\registry_key
: The path within the registry where the new entry will be created.
Example Output:
A new registry entry is created at the specified path, with confirmation provided in the form of PowerShell output.
Use Case 7: Create a New Blank Registry Entry with Specified Value
Code:
New-Item path\to\registry_key -Value value
Motivation:
Setting initial values within a registry key saves time and enhances precision during configurations, customizing applications, and managing system resources.
Explanation:
New-Item
: Initiates the creation of a new registry entry.path\to\registry_key
: Specifies where in the registry the entry will be created.-Value value
: Indicates the value to be assigned to the new registry entry upon creation.
Example Output:
The command accomplishes the creation of a registry entry with an assigned value, providing operational confirmation through PowerShell output.
Conclusion
The New-Item
command is a powerful tool within PowerShell, offering flexibility in managing files, directories, links, and registry entries. By understanding its use cases and syntax, users can efficiently automate tasks and manage systems within a Windows environment. Each example underscores the command’s versatility, making it a critical component of everyday administration tasks.