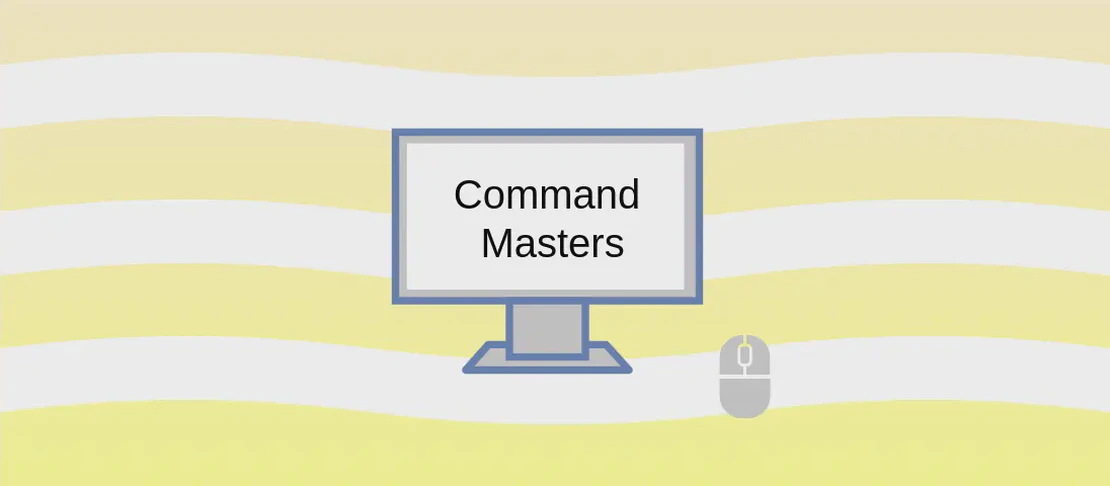
How to Use the Command 'ng' to Manage Angular Applications (with Examples)
The Angular CLI, triggered by the command ng
, is a powerful tool designed to streamline the development of Angular applications. It provides developers with commands to create, manage, build, and test Angular applications with minimal configuration. Used directly from the command line, ng
simplifies several tasks that are usually prone to manual repetition, making Angular development more efficient and less error-prone.
Use Case 1: Creating a New Angular Application
Code:
ng new project_name
Motivation:
Creating a new Angular application from scratch can be quite a complex task, involving setting up the project’s structure, installing necessary dependencies, and configuring the development environment. The ng new
command automates this entire process. It ensures that developers get a complete, ready-to-use boilerplate application with all required files and settings optimally configured. This is perfect for starting new projects quickly, allowing developers to focus more on writing code than setting up the initial structure.
Explanation:
new
: This specific command creates a new Angular project.project_name
: This argument specifies the name of the generated folder and application. It should be a valid DNS name.
Example Output:
Upon executing this command, a directory named project_name
is created in your current working directory. It contains a fully structured Angular application along with configuration files, an initial component, and styling defined by Angular standards.
Use Case 2: Adding a New Component
Code:
ng generate component component_name
Motivation:
Components are the basic building blocks of Angular applications. They encapsulate the view (HTML), style (CSS), and logic (TypeScript) of a section of the user interface. Rather than creating these files manually, the ng generate component
command automates the entire process. This not only saves time but ensures consistent structure and boilerplate code following Angular best practices.
Explanation:
generate
: This sub-command is used to create or alter any part of the Angular application.component
: Specifies the type of feature you want to generate.component_name
: The name of the component. This should usually be in kebab-case.
Example Output:
The command generates a new directory within the src/app
directory with the component name. Inside, it creates four files: a TypeScript file for logic, an HTML file for layout, a CSS file for styling, and a spec file for tests, all of which are auto-wired together.
Use Case 3: Adding a New Class
Code:
ng generate class class_name
Motivation:
Classes in Angular can be utilized for various purposes such as models or services that encapsulate logic and data representation. The ng generate class
command simplifies the creation of these classes, reducing the chance of human error and maintaining a consistent class structure throughout the codebase.
Explanation:
generate
: Indicates that you want to create something new.class
: Specifies the creation of a TypeScript class.class_name
: The name of the class, usually in PascalCase.
Example Output:
This command creates a new TypeScript file in the current project directory with the class name. The file contains a basic class declaration that can be immediately utilized for implementing logic or data modeling.
Use Case 4: Adding a New Directive
Code:
ng generate directive directive_name
Motivation:
Directives are used to attach behavior to elements in the DOM. Creating directives manually requires setting up several files and configurations, which can be prone to error. Using ng generate directive
streamlines this process, ensuring that the new directive is correctly scaffolded and integrated into the Angular project structure.
Explanation:
generate
: Indicates creation.directive
: Specifies the creation of an Angular directive.directive_name
: The desired name for the directive, usually in camelCase.
Example Output:
Executing this command leads to the creation of a new directory containing files for the directive coding, testing, and CSS styling. The generated files are automatically configured to ensure a swift setup.
Use Case 5: Running the Application
Code:
ng serve
Motivation:
Running an Angular application is essential for testing and development purposes. The ng serve
command compiles the application, watches for file changes, and serves the application on a local server. It provides immediate feedback on code changes, making it efficient for development iterations.
Explanation:
serve
: The command to start the local development server.
Example Output:
Upon running ng serve
, the console displays a compilation process. When complete, the application is available in the browser at http://localhost:4200/
, allowing for direct interaction and testing.
Use Case 6: Building the Application
Code:
ng build
Motivation:
Building an application prepares it for deployment. It compiles TypeScript into JavaScript, minifies files, and prepares assets for production. ng build
ensures the application is optimized, reducing load time and enhancing performance.
Explanation:
build
: The command to compile the application into an optimized, production-ready version.
Example Output:
This command generates a dist/
directory in the project folder, populated with static files ready for deployment onto a web server.
Use Case 7: Running Unit Tests
Code:
ng test
Motivation:
Testing is crucial for maintaining robust applications. The ng test
command runs unit tests using Karma, the default Angular testing framework. Automating tests helps detect and fix bugs early, ensuring the stability of the application across changes.
Explanation:
test
: Initiates the testing suite.
Example Output:
Running the command starts the test runner. It provides a report on the success or failure of each unit test, with details on failures for debugging and corrections.
Use Case 8: Displaying Angular Version
Code:
ng version
Motivation:
It is often essential to know the exact version of Angular and its dependencies for compatibility and support purposes. The ng version
command reports the version information of the Angular CLI and the Angular package used in an app, helping developers manage updates and avoid version conflicts.
Explanation:
version
: Displays the Angular CLI version and other project dependencies.
Example Output:
The output lists the version numbers for Angular CLI, Angular Framework, and other associated packages.
Conclusion:
The ng
command is a quintessential tool for Angular developers, providing a comprehensive suite of commands to enhance productivity and maintain consistency across the development lifecycle. These commands simplify the complexities of application creation, component management, building, testing, and version control, ensuring efficient and error-free Angular development.