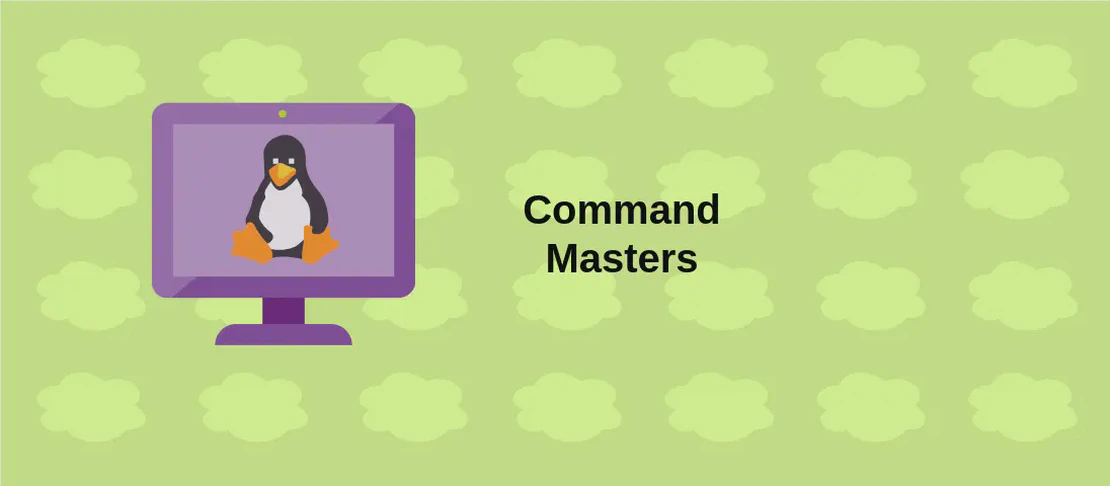
How to use the command 'nim' (with examples)
The Nim compiler provides developers with a comprehensive tool for processing, compiling, and linking source files written in the Nim programming language. Nim is known for its efficiency, expressiveness, and versatility, making it a popular choice for a variety of programming tasks. The compiler not only turns your Nim code into executable programs but also allows for performance optimizations, code analysis, and documentation generation among other capabilities.
Use case 1: Compile a source file
Code:
nim compile path/to/file.nim
Motivation:
When developing in Nim, the fundamental step of compiling your source code is essential for transforming human-readable code into machine-executable binaries. This use case illustrates the straightforward compilation of a Nim program, enabling you to verify the correctness of your code’s syntax and link it with the necessary libraries before execution.
Explanation:
nim
: This is the command-line interface for the Nim compiler, initiating the compilation process.compile
: This argument specifies the operation to be performed, instructing Nim to compile the specified source file.path/to/file.nim
: This is a placeholder for the actual path to your Nim source file, which should be replaced with your specific file path.
Example output:
Hint: used config file '/path/to/nim/config/nim.cfg' [Conf]
Hint: system [Processing]
Hint: yourfile [Processing]
CC: yourfile
CC: stdlib_system
Hint: operation successful: yourfile.c nimcache/stdlib_system.c
Use case 2: Compile and run a source file
Code:
nim compile -r path/to/file.nim
Motivation:
The ability to compile and instantly execute your Nim program is invaluable for iterative development and testing. This use case is perfect for when you need to see immediate results or outputs from your code after writing or modifying it. It streamlines your workflow by combining the compile-and-run steps into one command.
Explanation:
nim
: Invokes the Nim compiler.compile
: Indicates the intention to compile the file.-r
: This flag tells Nim to run the program immediately after it has been compiled successfully.path/to/file.nim
: This specifies the file path to your Nim source code.
Example output:
Hint: used config file '/path/to/nim/config/nim.cfg' [Conf]
Hint: system [Processing]
Hint: yourfile [Processing]
.....
CC: yourfile
CC: stdlib_system
Hint: operation successful: yourfile [Exec]
Running: ./yourfile
Hello, Nim World!
Use case 3: Compile a source file with release optimizations enabled
Code:
nim compile -d:release path/to/file.nim
Motivation:
When you are ready to deploy your Nim application, optimizing it for performance is crucial. Release optimizations include various compiler tricks and techniques that make your executable run faster and more efficiently. Use this command to ensure that your application utilizes the best possible performance optimizations provided by the Nim compiler.
Explanation:
nim
: Launches the Nim compiler.compile
: Specifies that the file needs to be compiled.-d:release
: This flag enables the compiler to apply an array of optimizations that enhance performance and efficiency in the compiled binary.path/to/file.nim
: The path to the Nim source file that you wish to compile with optimizations.
Example output:
Hint: used config file '/path/to/nim/config/nim.cfg' [Conf]
Hint: system [Processing]
Hint: yourfile [Processing]
.....
CC: yourfile [Release]
CC: stdlib_system [Release]
Hint: operation successful: yourfile.c nimcache/stdlib_system.c [Release]
Use case 4: Build a release binary optimized for low file size
Code:
nim compile -d:release --opt:size path/to/file.nim
Motivation:
In applications where binary size is a constraint, such as embedded systems or low-bandwidth environments, minimizing the file size without sacrificing functionality is vital. This command reduces the size of the generated release binary to ensure it fits constraints like limited storage or faster network transmission.
Explanation:
nim
: Executes the Nim compiler.compile
: Indicates compilation of the source code.-d:release
: Activates optimizations for a release build.--opt:size
: Directs the compiler to optimize specifically for a smaller file size.path/to/file.nim
: Indicates the path to the Nim file to be compiled.
Example output:
Hint: used config file '/path/to/nim/config/nim.cfg' [Conf]
Hint: system [Processing]
Hint: yourfile [Processing]
.....
CC: yourfile [Release Size]
CC: stdlib_system [Release Size]
Hint: operation successful: yourfile.c nimcache/stdlib_system.c [Release Size]
Use case 5: Generate HTML documentation for a module
Code:
nim doc path/to/file.nim
Motivation:
Comprehensive documentation is integral to large projects and team collaboration. Generating HTML documentation provides an easy-to-read format for understanding code structure, usage, and functionality. This is especially useful for open-source projects where newcomers need clear and accessible documentation.
Explanation:
nim
: Initiates the Nim compiler.doc
: Instructs Nim to generate documentation rather than compile the code.path/to/file.nim
: Specifies the path to the Nim source file from which HTML documentation will be generated.
Example output:
Generating documentation for: /path/to/file.nim [Doc]
Created file: ./file.html [Success]
Use case 6: Check a file for syntax and semantics
Code:
nim check path/to/file.nim
Motivation:
During development, ensuring your code follows correct syntax and semantics before proceeding to more resource-intensive compilation is a good practice. This command allows you to perform a quick check on your Nim source file, helping identify errors early and improve code quality.
Explanation:
nim
: Starts the Nim compiler.check
: Requests a syntax and semantic check rather than full compilation.path/to/file.nim
: Designates the path to the Nim source file to be checked.
Example output:
Hint: used config file '/path/to/nim/config/nim.cfg' [Conf]
Hint: system [Processing]
Hint: yourfile [Checking]
No errors detected in yourfile.nim
Conclusion:
The Nim compiler is a powerful toolset for processing, optimizing, and managing your Nim code. Whether you are developing simple scripts or complex applications, understanding and utilizing these commands maximize your efficiency and enhance your development process. Each use case here highlights the Nim compiler’s versatility - from basic compilation to advanced optimization, documentation generation, and error checking.