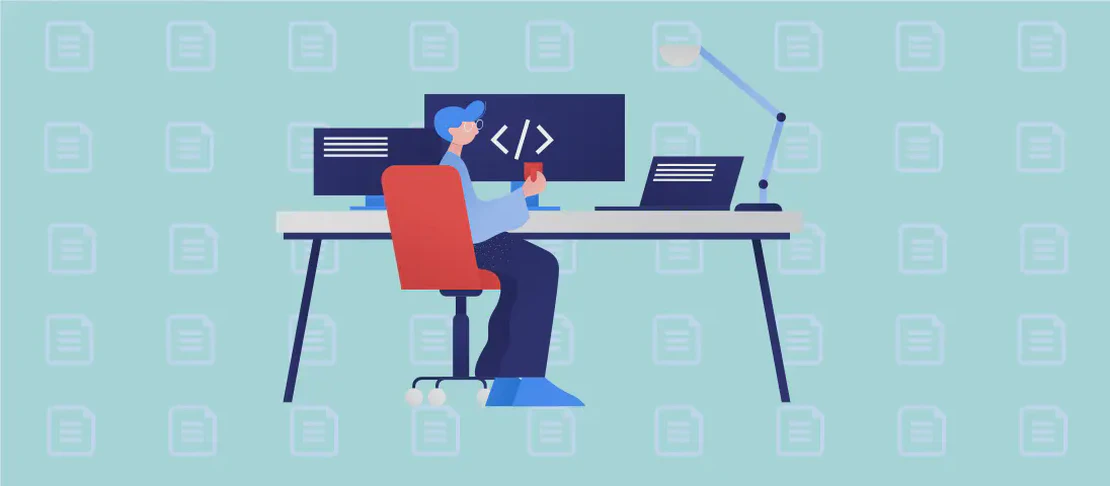
How to use the command 'ninja' (with examples)
The ’ninja’ command is a build system that is designed to be especially fast, generating outputs efficiently by minimizing unnecessary work. It is particularly useful in large software projects where extensive codebases require frequent rebuilding. Unlike other build systems, Ninja is minimalistic and focuses on speed by operating with lower latency. More information about Ninja can be found at Ninja Build Manual .
Use case 1: Build in the current directory
Code:
ninja
Motivation:
The primary motivation for using this specific command is to compile and build a project within the current directory. This straightforward usage is essential when you are working on a project, and your source code, along with the Ninja scripts, resides within the directory you are currently in. Executing ninja
without any options allows Ninja to automatically locate its build configuration file in the current directory and proceed with building all targets defined in that configuration.
Explanation:
ninja
: When executed without additional arguments, Ninja reads thebuild.ninja
file in the current directory and builds the default targets specified within that file. This approach is fundamental for developers looking for a straightforward and rapid way to initiate a build process.
Example output:
[1/2] Compiling source.c
[2/2] Linking output.exe
Use case 2: Build in the current directory, executing 4 jobs at a time in parallel
Code:
ninja -j 4
Motivation:
This command is beneficial when dealing with large projects and seeks to take advantage of multi-core processors by executing multiple jobs simultaneously. Specifying multiple jobs can significantly decrease build time, as it allows tasks to be handled concurrently. This is particularly useful for large-scale projects or when you have tasks that can be parallelized, such as compiling separate source files independently.
Explanation:
ninja
: Initiates the build process by reading from thebuild.ninja
file in the current directory.-j 4
: The-j
option specifies the number of jobs to run in parallel. Here, it tells Ninja to execute up to 4 jobs simultaneously, efficiently utilizing system resources and speeding up the build process.
Example output:
[1/8] Compiling source_1.c
[2/8] Compiling source_2.c
...
[8/8] Linking output.exe
Use case 3: Build a program in a given directory
Code:
ninja -C path/to/directory
Motivation:
This usage is ideal when your project or its build configuration is not located in your current working directory. By specifying the directory where your build.ninja
file is located, you can initiate the build process from anywhere in the filesystem, allowing for more flexibility in managing projects stored across various directories on your machine.
Explanation:
ninja
: Initiates the build process.-C path/to/directory
: This option changes the directory topath/to/directory
before running. It’s useful for executing Ninja builds in directories other than the current one. This is especially handy in environments with multiple or nested build directories.
Example output:
ninja: Entering directory `path/to/directory'
[1/1] Rebuilding target
Use case 4: Show targets (e.g., install
and uninstall
)
Code:
ninja -t targets
Motivation:
Knowing what targets are available is crucial for developers to understand what tasks are automatable within the build system. This command is particularly useful when you are not fully aware of all the tasks or outputs configured within your build.ninja
file. By listing all targets, developers can navigate complex build systems more effectively and execute desired tasks without unnecessary trial and error.
Explanation:
ninja
: Calls the Ninja build system to access its tool functionalities.-t targets
: The-t
flag stands for “tool,” andtargets
is a built-in tool that lists the targets defined in the build file. This command helps identify all potential build targets and actions.
Example output:
default: all files defined to be built by default
clean: script to remove intermediate files
install: script to install built resources
uninstall: script to uninstall resources
Use case 5: Display help
Code:
ninja -h
Motivation:
Access to command-line options and their explanations is valuable for both new users and experienced developers who might need a refresher or quick guidance on less frequently used features. This command serves as an entry point for understanding the broad functionalities provided by Ninja, helping in the effective utilization of its build capabilities.
Explanation:
ninja
: Starts the Ninja command-line interface.-h
: This option stands for “help” and provides a detailed explanation of usage, available commands, and options. It is vital for understanding how to properly execute Ninja commands and leverage its full potential.
Example output:
usage: ninja [options] [targets...]
options:
-w, --warn FILE emit warning if FILE is missing
-t TOOL [ARGS...] run a Ninja tool.
-v, --verbose show executed commands
...
Conclusion:
The ’ninja’ command stands out as a powerful and efficient build system, especially suitable for large projects that require rapid build processes. By leveraging its various options, such as parallel building and directory-specific building, Ninja allows developers to optimize their builds, as evident in these use cases. Whether listing available tasks or seeking help, mastering the ’ninja’ command can significantly streamline development workflows.