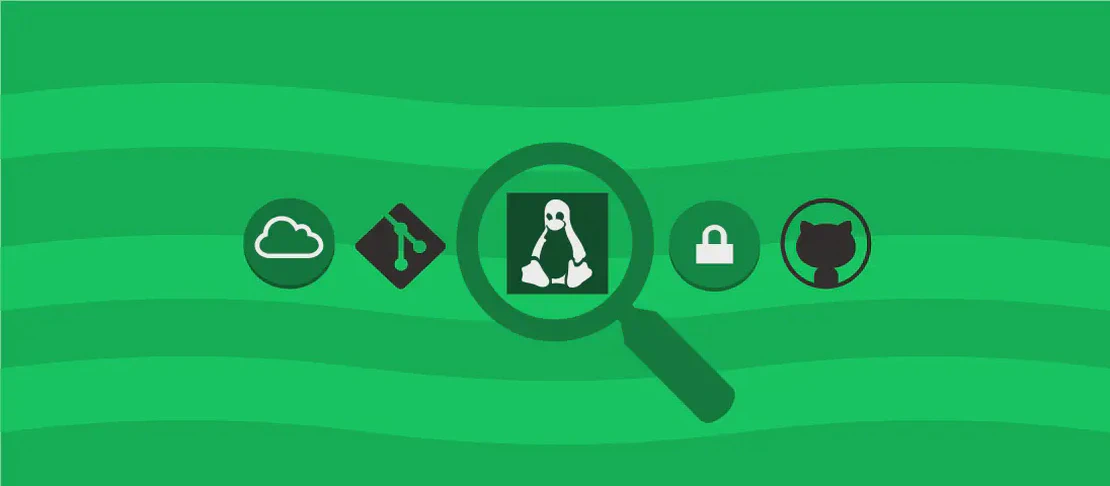
Node.js Command Examples (with examples)
Running a JavaScript File
To run a JavaScript file using the node
command, simply provide the file path as an argument:
node path/to/file.js
Motivation: Running a JavaScript file allows you to execute standalone scripts or build and run server-side applications written in JavaScript. By using Node.js, you can leverage the power of JavaScript on the server.
Explanation:
node
: Thenode
command starts the Node.js runtime environment.path/to/file.js
: This is the file path pointing to the JavaScript file you want to run.
Example Output:
If the JavaScript file contains console output, such as console.log("Hello, World!")
, running node path/to/file.js
would output Hello, World!
.
Starting a REPL (Interactive Shell)
To start a REPL (Read-Evaluate-Print Loop), use the node
command without any arguments:
node
Motivation: A REPL provides an interactive environment for testing and experimenting with JavaScript code. It is useful for quickly trying out small code snippets, exploring APIs, and debugging.
Explanation:
Running node
without any arguments starts an interactive shell where you can directly enter and execute JavaScript code. Each line of code entered is immediately evaluated, and the result (if any) is printed to the console.
Example Output:
When starting the REPL, you will see a prompt >
. You can then enter JavaScript code and see the immediate result. For example, entering 2 + 2
would output 4
.
Executing a File with Auto-Restart
To execute a JavaScript file and automatically restart the process when an imported file is changed, use the --watch
flag:
node --watch path/to/file.js
Motivation:
Using the --watch
flag is helpful during development when you want the Node.js process to automatically restart whenever a file it depends on is modified. This allows for faster iteration and more efficient debugging.
Explanation:
--watch
: This flag tells Node.js to monitor the specified file for changes.path/to/file.js
: This is the file path pointing to the JavaScript file you want to execute.
Example Output:
After running the node --watch path/to/file.js
command, the Node.js process will start and execute the specified file. If any imported file (e.g., a module or dependency) changes, the process will be automatically restarted.
Evaluating JavaScript Code from Command Line
To evaluate JavaScript code by passing it as an argument, use the -e
flag followed by the code:
node -e "console.log('Hello, World!')"
Motivation: Evaluating JavaScript code from the command line is useful when you want to quickly test a code snippet or perform one-off calculations or transformations without the need for an external file.
Explanation:
-e
: This flag specifies that the following argument should be treated as JavaScript code."console.log('Hello, World!')"
: This is an example JavaScript code that will be evaluated.
Example Output:
Running node -e "console.log('Hello, World!')"
would immediately execute the provided JavaScript code and output Hello, World!
to the console.
Evaluating and Printing the Result
To evaluate JavaScript code and print the result, use the -p
flag followed by the code:
node -p "Math.random()"
Motivation: Evaluating JavaScript code and printing the result is useful when you want to quickly obtain a computed value, fetch information from APIs, or examine the properties of built-in Node.js objects.
Explanation:
-p
: This flag specifies that the following argument should be treated as JavaScript code, and the result should be printed to the console."Math.random()"
: This is an example JavaScript code that generates a random number from 0 to 1.
Example Output:
Running node -p "Math.random()"
would immediately execute the provided JavaScript code and output a random number to the console, such as 0.456123789
.
Activating the Inspector
To activate the Inspector and pause execution until a debugger is connected, use the --inspect-brk
flag:
node --no-lazy --inspect-brk path/to/file.js
Motivation: The Inspector is a powerful tool for debugging Node.js applications. By activating it, you can pause the execution of your code, set breakpoints, and inspect variables, helping to identify and fix issues more effectively.
Explanation:
--no-lazy
: This flag disables lazy compilation, ensuring that breakpoints are set correctly.--inspect-brk
: This flag activates the Inspector and breaks the execution at the start of the specified file.
Example Output:
After running the node --no-lazy --inspect-brk path/to/file.js
command, the Node.js process will start and pause execution at the first line of the specified file. It will then wait for a debugger to connect before continuing execution.