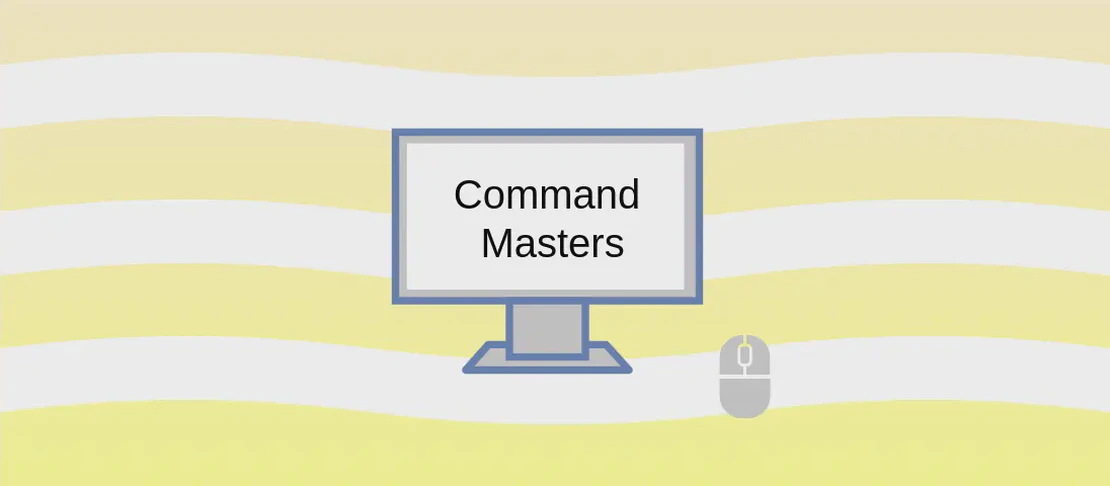
How to Effectively Use the Command 'nodemon' (with Examples)
Nodemon is a powerful utility specifically designed for node.js-based development environments. It monitors and watches the files within your node application. Whenever a change is detected in the files, nodemon will automatically restart your node application to reflect those modifications seamlessly, thus streamlining the development process. More information about nodemon can be found at nodemon.io .
Use case 1: Execute the specified file and watch a specific file for changes
Code:
nodemon path/to/file.js
Motivation:
When you’re performing active development on a JavaScript application, you often make frequent changes to your source code. The need to manually restart your node server after each change can be tedious and error-prone. By using nodemon in this manner, you eliminate this repetitive task as nodemon automatically handles restarting the server whenever it detects changes in the file.js
. This enhances productivity and helps ensure that your application behaves as expected immediately after any alterations.
Explanation:
nodemon
: This is the command to invoke the nodemon utility.path/to/file.js
: This specifies the file that nodemon will monitor. You need to replace this with the actual path to your JavaScript file.
Example output:
[nodemon] 2.0.15
[nodemon] to restart at any time, enter `rs`
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js,mjs,json
[nodemon] starting `node path/to/file.js`
Use case 2: Manually restart nodemon
Code:
rs
Motivation:
There are instances where, after making multiple changes or to apply certain manual updates, you might require restarting the application manually without changing files again. While nodemon is running, typing rs
allows for this immediate restart. This is particularly useful when dealing with non-file system changes like environment variable updates or when testing specific application states that can be triggered only at the application start.
Explanation:
rs
: This is a command issued in the terminal where nodemon is already active. It stands for “restart” and only functions while nodemon is running, providing a manual way to restart the node application.
Example output:
[nodemon] restarting due to manual restart
[nodemon] starting `node path/to/file.js`
Use case 3: Ignore specific files
Code:
nodemon --ignore path/to/file_or_directory
Motivation:
In some projects, there may be files or directories whose changes do not need to trigger a restart of your node application. Examples include log files, generated documentation, or other static asset files. By instructing nodemon to ignore certain files or directories, you can avoid unnecessary restarts, leading to a more efficient monitoring process.
Explanation:
nodemon
: Initiates the nodemon utility.--ignore path/to/file_or_directory
: This flag allows you to specify which files or directories nodemon should disregard. This path should be replaced with the specific file or directory path you want nodemon to ignore.
Example output:
[nodemon] 2.0.15
[nodemon] to restart at any time, enter `rs`
[nodemon] ignoring: path/to/file_or_directory
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js,mjs,json
[nodemon] starting `node path/to/file.js`
Use case 4: Pass arguments to the node application
Code:
nodemon path/to/file.js arguments
Motivation:
Frequently, node applications are designed to receive command line arguments to alter their behavior based on runtime conditions, such as specifying configurations, modes of execution, or data inputs. By using nodemon with additional arguments directed towards your application, you can ensure that it starts with the correct parameters, especially useful for testing differing scenarios or configurations from the command line.
Explanation:
nodemon
: Calls the nodemon utility.path/to/file.js
: The path to your JavaScript file to execute.arguments
: These are the arguments for your node application. Substitute “arguments” with the actual runtime arguments needed for your application.
Example output:
[nodemon] 2.0.15
[nodemon] to restart at any time, enter `rs`
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js,mjs,json
[nodemon] starting `node path/to/file.js arguments`
Use case 5: Pass arguments to node itself
Code:
nodemon arguments path/to/file.js
Motivation:
There may be occasions where you need to provide specific node options, like --inspect
for debugging purposes. By placing node arguments before your file path, you can customize the execution of the node runtime itself. This flexibility is crucial when debugging applications or managing memory allocation, performance tuning, or activating certain experimental features.
Explanation:
nodemon
: Executes the nodemon utility.arguments
: Node-specific arguments like--inspect
, which allows debugging.path/to/file.js
: The file you want to execute, this path should be the last in the command structure.
Example output:
[nodemon] 2.0.15
[nodemon] to restart at any time, enter `rs`
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js,mjs,json
[nodemon] starting `node --inspect path/to/file.js`
Debugger listening on ws://127.0.0.1:9229/...
Use case 6: Run an arbitrary non-node script
Code:
nodemon --exec "command_to_run_script options" path/to/script
Motivation:
You may have scenarios where you need to monitor non-Javascript files or scripts, such as bash scripts, as part of your process automation or testing routine. This capability allows nodemon to be more versatile, as you can use it to execute any command or script upon file changes, thereby serving a wider range of workflows beyond just node applications.
Explanation:
nodemon
: Starts the nodemon utility.--exec "command_to_run_script options"
: Defines the command or script to execute when a change is detected. Replacecommand_to_run_script options
with the proper command-line invocation.path/to/script
: The path to the script file you want to execute, which nodemon will monitor for changes.
Example output:
[nodemon] 2.0.15
[nodemon] to restart at any time, enter `rs`
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js
[nodemon] starting `command_to_run_script options path/to/script`
Use case 7: Run a Python script
Code:
nodemon --exec "python options" path/to/file.py
Motivation:
If your development workflow includes Python scripts or testing environments and you wish to monitor them for changes, nodemon can adapt to those needs. This can be invaluable when working on projects that involve both JavaScript and Python components, ensuring an integrated and efficient process for testing and development.
Explanation:
nodemon
: Initiates the nodemon utility.--exec "python options"
: Specifies Python to run the script, along with any Python-related options.path/to/file.py
: The specific Python script to execute, which nodemon will monitor for any detected changes.
Example output:
[nodemon] 2.0.15
[nodemon] to restart at any time, enter `rs`
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js
[nodemon] starting `python path/to/file.py`
Conclusion:
Nodemon proves to be a robust and versatile tool for enhancing the efficiency and convenience of development cycles across various scripting languages. From automating node application restarts to executing a variety of scripts, it offers an indispensable resource for developers. Each use case outlined above demonstrates how nodemon can be adjusted to fit specific development needs, ultimately fostering a more streamlined workflow.