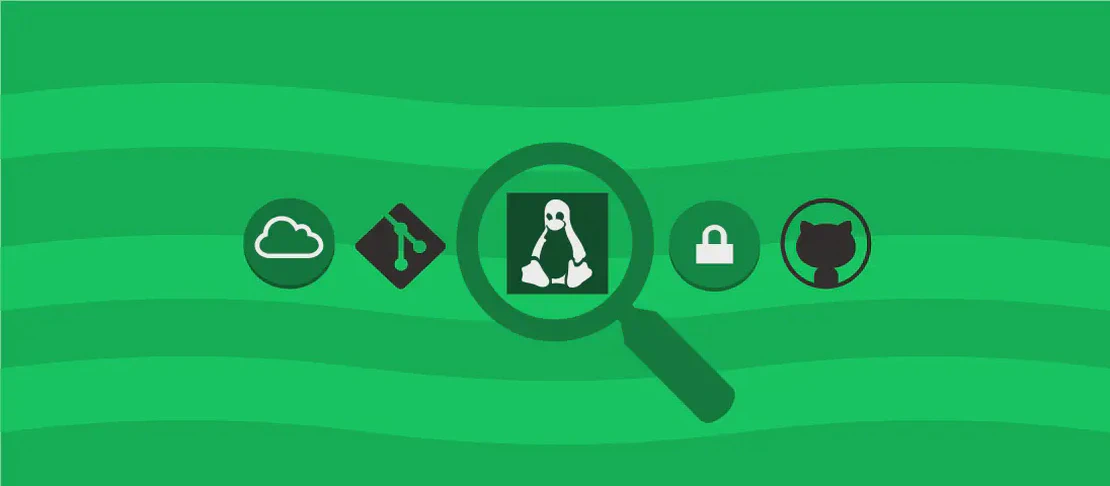
Managing Node.js Versions with nodenv (with examples)
Nodenv is a powerful tool that allows developers to manage different versions of Node.js effortlessly. Whether you are working on multiple projects that require different Node versions or you want to experiment with new changes in Node.js without affecting your current setup, nodenv provides a seamless way to install, switch, and manage Node.js versions. The flexibility of nodenv makes it an indispensable tool in a developer’s toolkit.
Install a Specific Version of Node.js
Code:
nodenv install version
Motivation:
Using different projects that require different Node.js versions is common in a developer’s work environment. For instance, one project might require Node.js 12 while another depends on the latest Node.js 16 features. Installing specific versions allows developers to align their environment with project requirements without conflicts.
Explanation:
nodenv
: This is the command-line interface used to manage Node.js versions.install
: This argument specifies that you wish to download and set up a particular Node.js version.version
: Replace this placeholder with the version number you want to install, such as14.17.0
.
Example Output:
Downloading Node.js source code...
-> http://nodejs.org/dist/v14.17.0/node-v14.17.0.tar.gz
Node.js 14.17.0 has been installed.
Display a List of Available Versions
Code:
nodenv install --list
Motivation:
Before installing a Node.js version, it’s valuable to see what versions are available. This helps in identifying which stable or experimental versions are ready for installation, ensuring compatibility and planned upgrades.
Explanation:
nodenv
: Utilized to manage various versioning tasks for Node.js.install
: Here, it initiates the process to list the versions.--list
: This flag ensures that instead of performing an installation, nodenv returns a list of all available Node.js versions that can be installed.
Example Output:
12.22.1
14.17.0
16.2.0
17.0.0
Use a Specific Version of Node.js Across the Whole System
Code:
nodenv global version
Motivation:
Some developers or development teams prefer a uniform Node.js version across all projects. This consistency is often necessary in a production environment where stability needs to be maintained, thus requiring a particular Node.js version for all processes running on the system.
Explanation:
nodenv
: This keeps managing Node.js versions seamless across different demands.global
: This sets the Node.js version as the default for the entire system.version
: It’s where you specify which version should be applied globally, like14.17.0
.
Example Output:
Global Node.js version set to 14.17.0
Use a Specific Version of Node.js with a Directory
Code:
nodenv local version
Motivation:
Different projects often have different Node.js version requirements. Using a local Node.js version allows a project to run within its specific configuration, independent of the system-wide setting, preventing version mismatches and facilitating smoother project development and deployment.
Explanation:
nodenv
: Command-line tool for version management in Node.js.local
: This specifies that the version should be used within the current directory, affecting only local operations.version
: Enter the desired Node.js version here, for example,12.22.1
.
Example Output:
Local Node.js version set to 12.22.1
Display the Node.js Version for the Current Directory
Code:
nodenv version
Motivation:
Knowing the active Node.js version in the current working directory is crucial for understanding the environment in which your application will run. This knowledge helps in ensuring that the development and production setups are consistent.
Explanation:
nodenv
: Useful for its ability to query the current Node.js version.version
: Directly queries the active Node.js version for the current directory.
Example Output:
14.17.0 (set by /Users/username/.nodenv/version)
Display the Location of a Node.js Installed Command (e.g., npm
)
Code:
nodenv which command
Motivation:
Tracking the source of executables like npm
ensures developers know exactly which version of tools are being used. This can prevent unexpected behaviors or bugs due to version inconsistencies, hence making debugging more straightforward.
Explanation:
nodenv
: Manages the finding of Node.js related executables.which
: This parameter helps to identify the path associated with Node.js commands.command
: Represents the executable for which you want to determine the path, oftennpm
.
Example Output:
/Users/username/.nodenv/versions/14.17.0/bin/npm
Conclusion:
Nodenv is a crucial utility for developers requiring flexible version control of Node.js in their environments. Its comprehensive set of commands empowers users to maintain precise Node.js setups, ensuring compatibility across various development and production scenarios. By strategically leveraging nodenv, developers can enhance their project’s stability and adaptability to different Node.js releases.