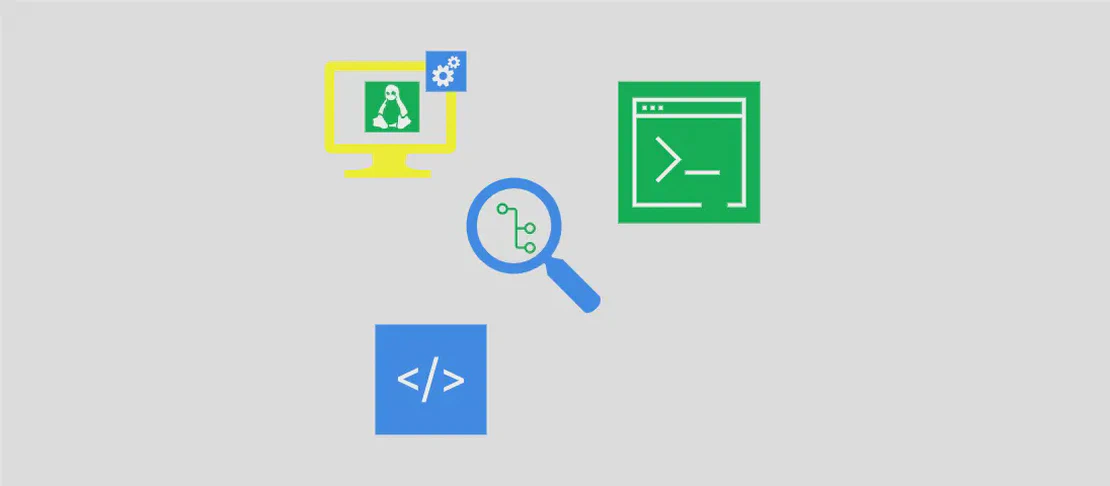
How to Use the Command 'nokogiri' (with Examples)
Nokogiri is a powerful parsing tool that handles the complexities of reading and manipulating HTML and XML documents. It is widely used in web scraping, data extraction, and even when working with digital document libraries. With its wide range of capabilities, Nokogiri simplifies working with structured data formats by providing easy-to-use methods that can be integrated into Ruby applications. Below are practical examples illustrating the various use cases of the nokogiri
command.
Parse the Contents of a URL or File
Code:
nokogiri https://www.example.com
Motivation:
Parsing web pages or files to extract specific information is a common task in data analysis and web development. By using Nokogiri, developers can read and manipulate document structures with ease. This use case is particularly common in web scraping where the dynamic content of a web page needs to be extracted for further analysis or storage.
Explanation:
In this command, nokogiri
is directed to parse the content found at https://www.example.com
. The tool can be pointed at either a URL or a file path, making it versatile for both online and local data sources.
Example Output:
Upon parsing, Nokogiri outputs the structured content of the webpage or file into a traversable format, displaying hierarchical tags and content.
Parse as a Specific Type
Code:
nokogiri path/to/file --type xml
Motivation:
HTML and XML are two different markup languages used for structuring data. When dealing with mixed content or needing specific processing guidelines, it can be essential to specify the type of document being handled. Parsing as a particular type ensures that Nokogiri applies the correct set of rules and methods for the given format, preserving the intended document structure.
Explanation:
The --type
option allows the tool to know whether it should treat the input as XML or HTML. Here, path/to/file
represents the file to be parsed, whereas --type xml
explicitly states that the content should be treated as XML, which is crucial for triggering the appropriate parsing and validation mechanisms.
Example Output:
This command would parse the XML file, respecting its hierarchical and nested structure, suitable for further XML processing or validation tasks.
Load a Specific Initialization File Before Parsing
Code:
nokogiri path/to/file -C path/to/config_file
Motivation:
Configuration files often contain initialization settings, data transformation rules, or custom scripts intended to modify how parsing should proceed. This feature is particularly useful when dealing with complex files that require customized, repeatable parsing logic without modifying the base data repeatedly.
Explanation:
Here, the -C
argument specifies the path to a configuration file that should be loaded before parsing. This file can contain settings or scripts that the parsing process should utilize, thus enabling customization according to specific needs or user preferences.
Example Output:
Nokogiri applies any transformations or settings specified in the config file, which might adjust how elements within path/to/file
are read or modified during parsing.
Parse Using a Specific Encoding
Code:
nokogiri path/to/file --encoding UTF-8
Motivation:
Different documents and web pages can use varying character encodings, from UTF-8, ASCII, to others like ISO-8859. Correctly interpreting a file’s encoding is crucial when extracting text data, ensuring that characters are read accurately and do not result in malformed output or errors due to encoding mismatches.
Explanation:
The --encoding
option lets you specify the character encoding that should be used while parsing. In the example, UTF-8
is identified as the desired encoding, which is a common and versatile choice capable of representing many characters found across global languages.
Example Output:
When parsing with this command, Nokogiri respects the specified UTF-8 encoding, ensuring all characters are accurately read and any output showcases the correct textual data as intended.
Validate Using a RELAX NG File
Code:
nokogiri path/to/file --rng path/to/schema.rng
Motivation:
Validating documents against a predefined schema is essential in both development and production environments, ensuring data integrity and consistency. Using a RELAX NG schema can help identify deviations, errors, or unexpected structures within an XML document, promoting reliability in data manipulation or storage tasks.
Explanation:
The option --rng
specifies a Relax NG schema file (path/to/schema.rng
) for validation against the input document (path/to/file
). This validation step ensures that the data meets expected structural conventions and constraints defined within the schema.
Example Output:
Upon execution, Nokogiri evaluates the XML file against the RELAX NG schema and outputs validation messages. These could confirm successful validation or highlight discrepancies between the document and schema.
Conclusion
Nokogiri is an extremely versatile command-line tool that facilitates the handling of HTML and XML documents in a streamlined manner. By parsing different types of input, specifying document types, utilizing encoding standards, and validating structures against external schemas, Nokogiri remains a go-to solution for developers and data analysts requiring robust and precise data processing capabilities.