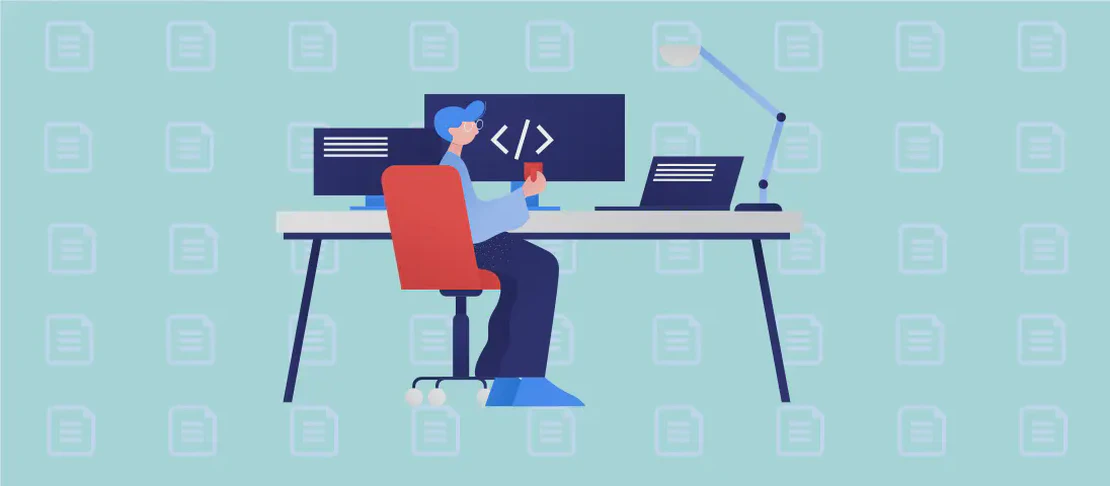
How to Manage Package Access Using 'npm access' (with examples)
The npm access
command is a powerful tool for managing access to npm packages. As a package owner, it’s crucial to control who can view, edit, or publish your packages. With npm access
, you can set access levels, list collaborators, manage package status, and configure access settings. This command ensures that only authorized users can modify or interact with your published packages, thereby maintaining the security and integrity of your code.
Use case 1: List packages for a user or scope
Code:
npm access list packages user|scope|scope:team package_name
Motivation:
You might be working in a team where multiple people contribute to various npm packages. Listing all packages for a specific user or a team scope helps you track package ownership and collaboration responsibilities. It provides clarity on who is responsible for which package, making package management more organized.
Explanation:
user|scope|scope:team
: Replace this with either a specific user, a scope, or a team within a scope. This parameter identifies whose package access you want to view.package_name
: Replace with the name of the package you’re interested in.
Example output:
[ 'package1', 'package2', 'package3' ]
This output lists all packages that a specific user, scope, or team has access to.
Use case 2: List collaborators on a package
Code:
npm access list collaborators package_name username
Motivation:
Knowing who has access to your npm package is crucial for maintaining control over who can contribute or alter your package. Listing collaborators ensures that you are aware of all individuals and roles with current access, allowing for better governance and security.
Explanation:
package_name
: The name of the npm package for which you want to list collaborators.username
: A specific user to see their role or access status on the package.
Example output:
{
"user1": "read-write",
"user2": "read-only"
}
The above output shows the access level (read-write or read-only) for each collaborator on the specified package.
Use case 3: Get status of a package
Code:
npm access get status package_name
Motivation:
Before sharing your package link or adding it to a project, you might want to check whether your npm package is public or private. Public packages can be accessed and installed by anyone, whereas private packages are restricted to authorized users only. Knowing the current status can dictate your distribution strategy.
Explanation:
package_name
: The name of the package whose status you wish to retrieve.
Example output:
status: public
This output confirms that the package is currently set to a public access level, meaning anyone can access it.
Use case 4: Set package status (public or private)
Code:
npm access set status=public|private package_name
Motivation:
Switching a package between public and private can be necessary when transitioning between development phases. For instance, a private package might be made public after thorough testing and review, or a public package might be switched to private for security reasons or exclusive access.
Explanation:
status=public|private
: Choose eitherpublic
to make the package accessible to everyone orprivate
to restrict access.package_name
: The name of the package you want to change the status for.
Example output:
status updated: package_name is now private
The package’s access setting has been successfully updated to private.
Use case 5: Grant access to a package
Code:
npm access grant read-only|read-write scope:team package_name
Motivation:
When working within collaborative environments or specific teams, you may need to share packages with varying degrees of permissions. Granting access allows teams to either view or contribute to the package, enabling more collaborative development workflows.
Explanation:
read-only|read-write
: Specify whether the scope or team’s access level should beread-only
(view without editing) orread-write
(view and edit permissions).scope:team
: The scope and team to which you allow package access.package_name
: The package name you wish to grant access to a specific team or scope.
Example output:
team scope:team now has read-write access to package_name
The specified team now has the granted level of access, promoting teamwork and coordination.
Use case 6: Revoke access to a package
Code:
npm access revoke scope:team package_name
Motivation:
There might be situations where the access to a package should be restricted or removed due to changes in project requirements or team composition. Revoking access from a specific team ensures that only the right people have access to sensitive package data.
Explanation:
scope:team
: Designates which team or scope will lose access to the package.package_name
: The name of the package from which you are revoking access.
Example output:
access for scope:team has been revoked on package_name
The specified team no longer has access, maintaining secure control over package information.
Use case 7: Configure two-factor authentication requirement
Code:
npm access set mfa=none|publish|automation package_name
Motivation:
Increased security measures like two-factor authentication (2FA) reduce the risk of unauthorized access and potential malicious activities. Configuring 2FA requirements for a package ensures that only verified and authorized users can proceed with actions such as publishing or automation.
Explanation:
mfa=none|publish|automation
: Determines the kind of operations that require 2FA.none
disables 2FA,publish
enforces 2FA for publishing actions, andautomation
enforces 2FA for automation scripts.package_name
: The package name you want to apply two-factor authentication settings for.
Example output:
mfa configured for package_name, requires mfa for publish actions
The package now requires 2FA for the specified actions, enhancing its security.
Conclusion:
npm access
is an essential tool in the arsenal of npm package management. By understanding and utilizing its rich set of commands, package owners can precisely control who accesses their packages and how. From listing collaborators to configuring two-factor authentication, npm access
offers flexibility and security, making npm package management both efficient and secure.