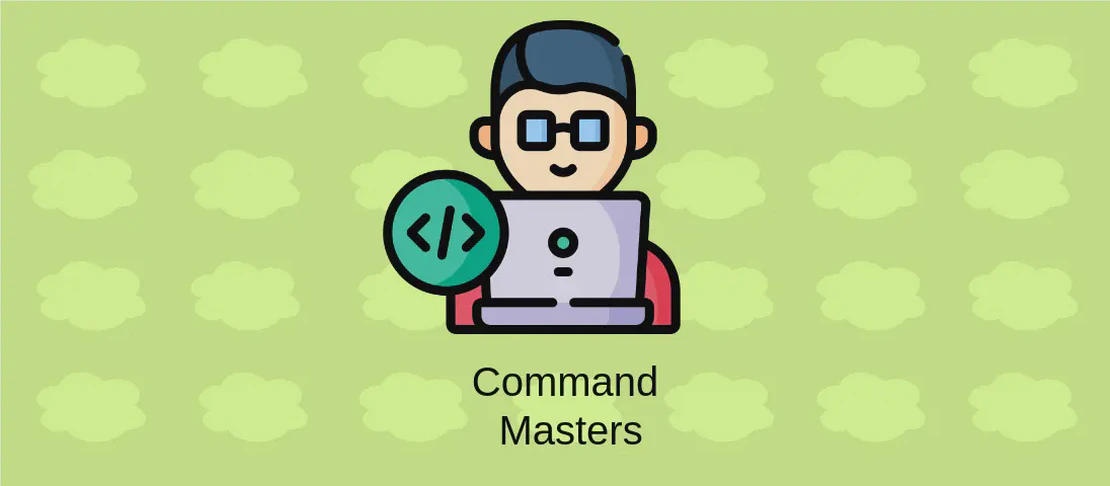
Mastering `npm audit` for Dependency Security (with examples)
In the world of Node.js, package management and dealing with dependencies are an integral aspect of developing robust applications. The npm audit
command is a powerful tool provided by npm (Node Package Manager) to help developers maintain the security of their applications by scanning for vulnerabilities within their project dependencies. By analyzing the project’s package.json
and package-lock.json
, npm audit
identifies and reports vulnerabilities, offers remediation suggestions, and can even automatically fix them. This article will explore each use case of the npm audit
command, illustrating its practical applications with examples.
Scan the project’s dependencies for known vulnerabilities
Code:
npm audit
Motivation:
Regular vulnerability scanning is crucial for maintaining the security health of your application. By routinely executing npm audit
, developers can quickly identify and address security flaws that could potentially be exploited by attackers. This baseline check helps ensure that your project’s dependencies are as secure as possible.
Explanation:
npm audit
: This command on its own initiates a security scan of all the dependencies listed in your project’spackage.json
file and contrasts them with known vulnerabilities in npm’s security database. It produces a summary report, indicating any vulnerabilities along with their severity.
Example Output:
# npm audit report
# Run npm install --global npm@latest to upgrade npm to 7.0.0
# │
# ├── Underscore CVE-2019-10744
# │ For more information, visit https://npmjs.com/advisories/1679
#
# found 2 low severity vulnerabilities in 1111 scanned packages
Automatically fix vulnerabilities in the project’s dependencies
Code:
npm audit fix
Motivation:
Automating the remediation of vulnerabilities is a time-saver and ensures that dependencies are promptly updated. npm audit fix
is instrumental for addressing vulnerabilities with minimal manual intervention, helping maintain a cleaner and safer dependency tree without manual adjustments.
Explanation:
npm audit fix
: This command assesses the vulnerabilities detected and attempts to apply patches or updated versions of dependencies wherever available, automatically modifying yourpackage-lock.json
andnode_modules
to incorporate these changes.
Example Output:
fixed 1 of 2 vulnerabilities in 1111 scanned packages
1 vulnerability required manual review and could not be updated
Force an automatic fix to dependencies with vulnerabilities
Code:
npm audit fix --force
Motivation:
Sometimes, standard fixes may leave certain vulnerabilities unresolved due to potential significant changes in dependencies. Utilizing the --force
option overrides these reservations, aligning all dependencies to their latest versions irrespective of breaking changes. This is powerful but potentially risky in a production setting.
Explanation:
npm audit fix
:--force
: This flag instructs npm to apply updates even if it means updating dependencies to major (breaking) versions. This can significantly alter the project’s behavior but is necessary when vulnerabilities need urgent remediation without the time for careful version management.
Example Output:
fixed 2 of 2 vulnerabilities in 1111 scanned packages
2 packages were updated, 10 packages were updated with breaking changes
Update the lock file without modifying the node_modules
directory
Code:
npm audit fix --package-lock-only
Motivation:
There are scenarios where developers may need to update only the package-lock.json
without physically altering the installed modules. This can serve as a preparatory step before applying a more thorough update or during codebase audits where system consistency needs to be maintained.
Explanation:
npm audit fix
:--package-lock-only
: The flag directs the audit to perform fixes only in thepackage-lock.json
, leaving actual installed modules innode_modules
unchanged. This option is particularly useful for preparing updates or testing for conflicts without changing the active code.
Example Output:
fixed 0 of 2 vulnerabilities in 1111 scanned packages
2 vulnerabilities require manual review
package-lock.json was updated
Perform a dry run. Simulate the fix process without making any changes
Code:
npm audit fix --dry-run
Motivation: Before executing a series of changes, it’s beneficial to simulate those changes to understand their impact. Running a dry run gives users foresight into how fixes would be applied, allowing for evaluation and decision-making before committing any actual transformation in their dependency management.
Explanation:
npm audit fix
:--dry-run
: This option performs a simulated audit fix, presenting all fixes that would occur without making any changes to thenode_modules
directory or thepackage-lock.json
file, providing insightful foresight while preserving the current project state.
Example Output:
found 2 vulnerabilities (1 low, 1 high) in 1111 scanned packages
fixed 1 vulnerability with a potential update
the dry run leaves code unmodified
Output audit results in JSON format
Code:
npm audit --json
Motivation: Advanced users or automated systems often require audit outcomes in structured formats like JSON for further processing or integration into continuous integration pipelines. JSON-formatted output facilitates programmatic parsing and integration with tooling for enhanced security measures.
Explanation:
npm audit
:--json
: By appending this flag, the audit output is converted into a JSON format, which is especially useful when results are needs parsing or redirection into other software modules or systems for automated processing.
Example Output:
{
"actions": [],
"advisories": {},
"muted": [],
"metadata": {
"vulnerabilities": {
"info": 0,
"low": 1,
"moderate": 0,
"high": 1,
"critical": 0
},
"dependencies": 1111,
"devDependencies": 0,
"optionalDependencies": 0,
"totalDependencies": 1111
}
}
Configure the audit to only fail on vulnerabilities above a specified severity
Code:
npm audit --audit-level=high
Motivation: In environments where rigorous security standards must be adhered to, focusing on crucial vulnerabilities above a certain severity becomes essential. Configuring the audit process to flag only significant threats streamlines focus on immediate risk management and aligns with organizations’ security protocols.
Explanation:
npm audit
:--audit-level=high
: This argument sets a threshold for vulnerability severity, ensuring that the audit process flags only those issues classified at or above the chosen severity level (info, low, moderate, high, critical), allowing tailored oversight that meets the project’s security requirements and organizational practices.
Example Output:
found 1 high severity vulnerability in 1111 scanned packages
run `npm audit` for details
Conclusion:
Harnessing the various capabilities of npm audit
empowers developers to confront their project’s dependencies with a robust security-centric approach. From basic checks to automated fixes and conditional vulnerability flagging, npm audit
tools provide a comprehensive framework for ensuring that Node.js applications remain secure against known risks, allowing developers to prioritize coding with confidence in their project’s security posture.