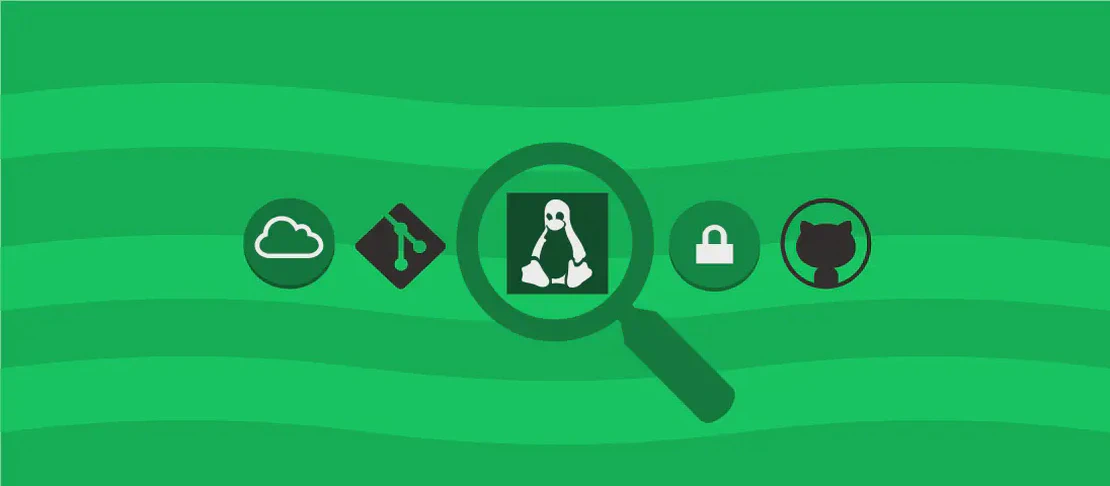
Mastering the npm Cache Command (with examples)
The npm cache command is an essential tool for managing the cache of npm packages. It’s part of npm (Node Package Manager), which is widely used for handling various packages within Node.js projects. The cache is an important aspect as it helps in speeding up the installation process by storing data that npm fetches during installs. Managing this cache effectively can lead to better performance and can resolve potential issues related to corrupted data.
Use case 1: Add a specific package to the cache
Code:
npm cache add package_name
Motivation:
Adding a specific package to the cache is useful when preparing an environment for offline installation or when you want to ensure that a package that you rely on frequently is readily available. This can be particularly beneficial in a development environment where network bandwidth is limited or unreliable.
Explanation:
npm cache
- This invokes the cache management command within npm.add
- This sub-command indicates that you want to add something to the cache.package_name
- This is a placeholder for the actual name of the package you want to cache.
Example Output:
Added package_name to the cache
Use case 2: Remove a specific package from the cache
Code:
npm cache remove package_name
Motivation:
Removing a specific package from the cache is necessary when you want to free up disk space or when you suspect that the cached package might be corrupted or outdated. This ensures that the next time you need this package, it will be downloaded fresh from the registry.
Explanation:
npm cache
- Invokes the cache command.remove
- Indicates removal action within the cache.package_name
- Stands for the package you wish to remove from the cache.
Example Output:
Removed package_name from the cache
Use case 3: Clear a specific cached item by key
Code:
npm cache clean key
Motivation:
Clearing a specific cached item by key allows for precise cache management. This is helpful when you have a specific cache artifact identified by a key that you no longer need or want to refresh.
Explanation:
npm cache
- Calls the cache management tool.clean
- Specifies the cleaning action.key
- Represents the specific cache item to be cleared, identified by its key.
Example Output:
Cleaned cache item corresponding to key
Use case 4: Clear the entire npm cache
Code:
npm cache clean --force
Motivation:
Clearing the entire npm cache is a drastic but occasionally necessary action, especially in cases where the cache has become entirely corrupt or if storage space is critically low. This ensures that all old data is removed and can be a clean slate for future package installations.
Explanation:
npm cache
- Opens up the cache command suite.clean
- Initiates the cleaning process.--force
- This flag is essential as it forces the complete clearing of the cache, given that this is a potentially destructive action.
Example Output:
Cache cleared
Use case 5: List the contents of the npm cache
Code:
npm cache ls
Motivation:
Listing the contents of the cache is an informative action that helps you see what is currently stored in the cache. This can be used for auditing purposes or to verify that a particular package is indeed cached.
Explanation:
npm cache
- Engages the cache module.ls
- Abbreviation for ’list’, this command will list all items currently in the cache.
Example Output (truncated):
package1@1.0.0
package2@2.3.1
Use case 6: Verify the integrity of the npm cache
Code:
npm cache verify
Motivation:
Verifying the integrity of the npm cache is critical to ensuring that your cached data is not corrupted. By running this command, you can prevent issues related to corrupted package installations which can lead to faulty application behavior.
Explanation:
npm cache
- Access the cache functionality.verify
- This command checks the integrity and validity of the items stored within the cache.
Example Output:
Cache verified: 345 files, 29.1MB
Integrity: 100% of files verified
Use case 7: Show information about the npm cache location and configuration
Code:
npm cache dir
Motivation:
Knowing where the npm cache is stored and how it is configured is useful for system administration tasks or when you need to manually inspect, back up, or move your cache to another location.
Explanation:
npm cache
- Summons the cache command.dir
- This will output the directory path where the npm cache is currently stored.
Example Output:
/Users/username/.npm/_cacache
Use case 8: Change the cache path
Code:
npm config set cache path/to/directory
Motivation:
Sometimes it might be necessary to change the default location of the npm cache. This can be due to disk space constraints or organizational policies that dictate storing cache data on certain volumes or devices.
Explanation:
npm config
- Accesses the configuration aspect of npm.set cache
- The subcommands here are for setting the cache configuration.path/to/directory
- This is a placeholder for the actual path to the new cache location you wish to set.
Example Output:
cache = "path/to/directory"
Conclusion:
The npm cache command is a versatile and powerful tool for managing package cache in Node.js environments. Whether you’re troubleshooting potential issues, optimizing performance, or adapting to system constraints, understanding how to use these commands enhances your control over project dependencies. Such capabilities can significantly streamline package management tasks, improve application reliability, and boost overall development efficiency.