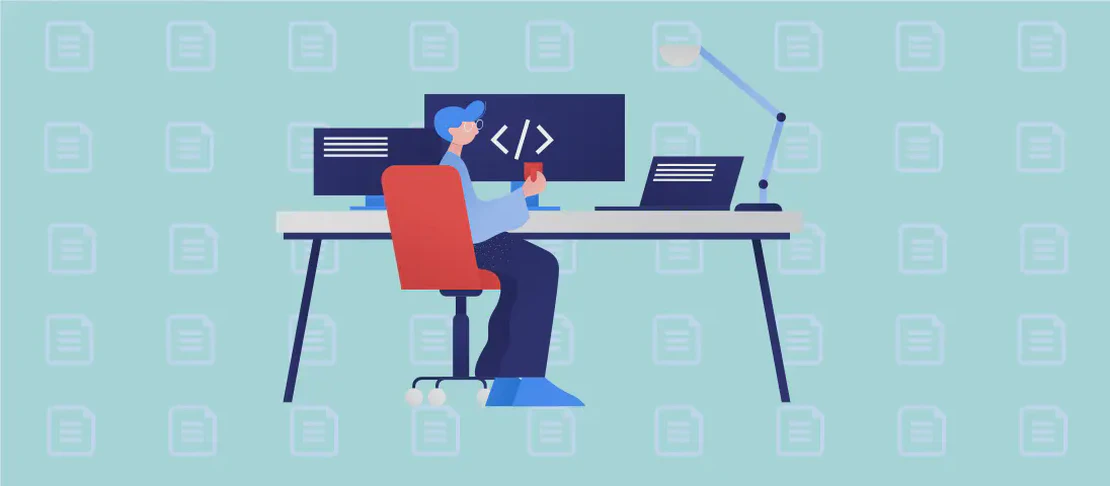
How to Use the Command 'npm' (with examples)
NPM (Node Package Manager) is an essential tool for JavaScript developers, helping in managing the various dependencies involved in a Node.js project. It enables easy installation, version management, and uninstallation of packages. Whether you are just starting out on a small project or managing a large scale application, understanding how to effectively use npm commands can significantly enhance your workflow and productivity.
Use case 1: Create a package.json
file with default values
Code:
npm init -y
Motivation:
Creating a package.json
file is the first step in setting up a Node.js project. It serves as the project’s manifest file, listing metadata relevant to the project and includes the list of packages your project depends on. By using the command with the -y
flag, you can bypass the interactive setup, automatically setting default values for all fields in package.json
. This is particularly useful when you want to quickly scaffold a project without being interrupted by setup prompts.
Explanation:
npm init
: This initiates the creation of apackage.json
file.-y
or--yes
: This flag automatically accepts the default options for all fields inpackage.json
, bypassing the interactive setup questions.
Example output:
{
"name": "your-project-name",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Use case 2: Download all the packages listed as dependencies in package.json
Code:
npm install
Motivation:
When collaborating on a project or shifting work between environments, it’s crucial to ensure consistency in the project’s dependencies. Running npm install
will automatically read through the package.json
file, download, and set up all necessary dependencies listed within. This is the simplest way to recreate the exact development environment specified by your package.json
, ensuring all team members are working with the same libraries and versions.
Explanation:
npm install
: This command reads thepackage.json
file and installs all dependencies and devDependencies listed in it.
Example output:
added 50 packages in 2s
Use case 3: Download a specific version of a package and add it to the list of dependencies in package.json
Code:
npm install lodash@4.17.21
Motivation:
Sometimes, maintaining compatibility with existing code necessitates installing a specific version of a package. The ability to specify a particular version of a library resolves compatibility issues that may arise due to breaking changes or regressions in newer package releases. Through this command, you ensure the continuity and stability of your application by adhering to known and tested versions.
Explanation:
npm install
: Initiates the installation process.lodash@4.17.21
: Specifies the package namelodash
and the desired version4.17.21
. This package will be installed at this exact version and added topackage.json
.
Example output:
+ lodash@4.17.21
added 1 package in 1s
Use case 4: Download the latest version of a package and add it to the list of dev dependencies in package.json
Code:
npm install jasmine -D
Motivation:
Development dependencies include tools needed for development, testing, and building processes rather than the final production output. Installing a package as a dev dependency is perfect for including tests, linters, or transpilers, such as Jasmine, Mocha, or Babel. This command ensures these tools are easily accessible during development but are not included in the production bundle.
Explanation:
npm install
: Initiates the installation process.jasmine
: The name of the package to install.-D
or--save-dev
: This flag specifies that the package is to be included in thedevDependencies
section ofpackage.json
, which designates packages necessary only during development.
Example output:
+ jasmine@3.6.4
added 23 packages in 1.5s
Use case 5: Download the latest version of a package and install it globally
Code:
npm install -g nodemon
Motivation:
Global installation of a package is ideal for utilities or tools that are meant to be available from the command line, across multiple projects. For instance, nodemon
is a tool that automatically restarts a Node application when file changes are detected, and having it accessible globally allows it to be used across any project without needing to install it each time.
Explanation:
npm install
: Initiates the installation process.-g
or--global
: This flag ensures the package is installed globally, making it available system-wide.nodemon
: The name of the package to install.
Example output:
+ nodemon@2.0.14
added 118 packages in 8s
Use case 6: Uninstall a package and remove it from the list of dependencies in package.json
Code:
npm uninstall lodash
Motivation:
As projects evolve, certain packages may become redundant or unnecessary. Uninstalling a package is essential to keep your project lightweight and free from obsolete or unused dependencies. This command is vital in maintaining an efficient codebase and ensuring systematic dependency management by promptly removing unused packages and updating the package.json
file accordingly.
Explanation:
npm uninstall
: Initiates the uninstallation process.lodash
: The name of the package to uninstall, which will remove it from the node_modules directory and the dependencies section of thepackage.json
file.
Example output:
removed 1 package and audited 49 packages in 1s
Use case 7: List all locally installed dependencies
Code:
npm list
Motivation:
To swiftly get an overview of the packages your project relies on, listing all locally installed dependencies provides tremendous value. This command is particularly useful when auditing your project’s packages, ensuring correct versions are installed, and checking for redundant or conflicting dependencies. A comprehensive dependency overview helps maintain system integrity during development stages.
Explanation:
npm list
: Displays the dependency tree of the current project, listing all locally installed packages found in the node_modules directory.
Example output:
project-name@1.0.0 /path/to/project
├── lodash@4.17.21
└── express@4.17.1
Use case 8: List all top-level globally installed packages
Code:
npm list -g --depth 0
Motivation:
Managing globally installed packages can become cumbersome, especially if many utility tools are installed over time. Having a clear understanding of what is installed globally on your system is crucial for system-wide package management. This command allows developers to maintain a clear inventory of all top-level globally installed packages, essential for a clean and efficient development environment.
Explanation:
npm list
: Displays the dependency tree.-g
or--global
: This flag specifies to list globally installed packages.--depth 0
: Limits the displayed tree depth to zero, ensuring only top-level packages are shown.
Example output:
/usr/local/lib
├── nodemon@2.0.14
└── npm@6.14.11
Conclusion:
Mastering npm commands is an essential skill for JavaScript developers, as it greatly enhances the efficiency and manageability of Node.js project development. By understanding and effectively using commands like npm init
, npm install
, and others, developers can set up, maintain, and optimize their projects with ease. With a structured approach to dependency management, npm ensures projects remain stable, secure, and up-to-date, thus fortifying the backbone of modern software development.