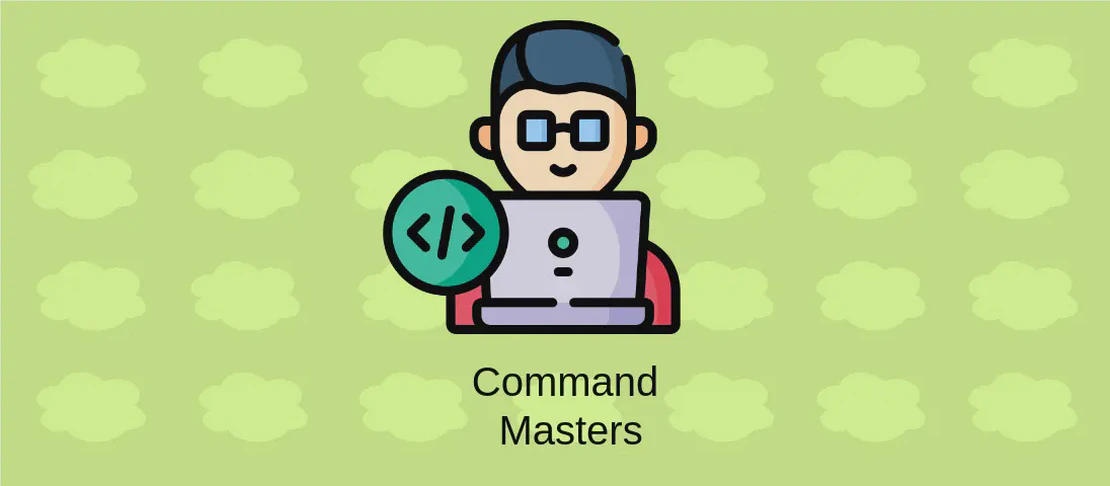
How to Use the Command `npm find-dupes` (with Examples)
The npm find-dupes
command is a useful utility provided by npm that helps developers identify duplicate dependencies within their node_modules
directory. These duplicates often result from different versions of the same package being installed as dependencies of various modules. Identifying and resolving these duplicates can optimize your project’s size and performance, as well as prevent potential conflicts or issues arising from mismatched versions.
Use case 1: List all duplicate packages within node_modules
Code:
npm find-dupes
Motivation:
When working on a JavaScript project, especially in a large-scale application, you may quickly accumulate numerous dependencies. Over time, different parts of your application could rely on different versions of the same package, leading to redundancy. This command helps in identifying these duplicates so you can streamline your project by consolidating dependency versions where possible.
Explanation:
The command npm find-dupes
is executed without additional arguments. It scans the node_modules
directory for duplicate dependencies, focusing solely on the required packages used in the project. It’s a straightforward command aiming to provide a comprehensive list of redundant packages that exist due to version variations.
Example output:
Duplicate packages found:
- lodash
- versions: 4.17.20, 4.17.21
- react
- versions: 16.13.1, 16.14.0
Use case 2: Include devDependencies
in duplicate detection
Code:
npm find-dupes --include=dev
Motivation:
While managing project dependencies, developers often overlook devDependencies
during optimization because they are not bundled with the production build. However, having multiple versions of these in development can increase dependency bloat and cause unnecessary confusion and complexity during the development process. By including devDependencies
, the command offers a more thorough examination of the entire project’s dependency tree.
Explanation:
The --include=dev
flag instructs npm to include devDependencies
in the duplicate detection process. This means the command will not only look at the dependencies necessary for your production-ready application but also those used for development and testing, thus providing a holistic view of duplicates across all types of dependencies.
Example output:
Duplicate packages found:
- mocha
- versions: 8.2.1, 8.3.0
- eslint
- versions: 7.14.0, 7.15.0
Use case 3: List all duplicate instances of a specific package in node-modules
Code:
npm find-dupes package_name
Motivation:
This use case is handy when you suspect a particular package is causing conflicts or when you want to verify the versions of a specific dependency used across your application. Specifying a package name allows for a focused scan, reducing the time and computational resources required for a complete audit of the node_modules
directory.
Explanation:
By replacing package_name
with the actual name of the package you’re interested in, the command will narrow its search to entries related to that package. This targeted search helps pinpoint problems linked directly to a specific dependency, which might be causing compatibility or functionality issues.
Example output:
Duplicate instances of lodash found:
- versions: 4.17.19, 4.17.20, 4.17.21
Use case 4: Exclude optional dependencies from duplicate detection
Code:
npm find-dupes --omit=optional
Motivation:
Optional dependencies might not be crucial for your application’s core functionality; they may provide additional features or enhancements that aren’t strictly necessary. As such, developers might choose to exclude these from duplicate checks to focus on more critical components, especially if they suspect that optional dependencies are less likely to impact application performance significantly.
Explanation:
The --omit=optional
flag tells the command to ignore optional dependencies during its search for duplicates. This results in a more streamlined output, focusing only on dependencies essential to the application’s main operations.
Example output:
Duplicate packages found excluding optional dependencies:
- chalk
- versions: 4.0.0, 4.1.0
- axios
- versions: 0.21.0, 0.21.1
Use case 5: Set the logging level for output
Code:
npm find-dupes --loglevel=silent|error|warn|info|verbose
Motivation:
Depending on the context in which duplication analysis is being conducted, users might require more or less verbosity in the command output. For example, during a routine check, concise information about duplicates may be sufficient, while a detailed log is more appropriate during debugging or integration into a continuous integration pipeline. Adjusting the log level allows for tailored output to fit these contrasting scenarios.
Explanation:
The --loglevel
option provides control over how much detail is included in the command’s output, where:
silent
: Suppresses all output.error
: Displays only error messages.warn
: Shows warnings.info
: Provides general information (default level).verbose
: Offers detailed information about the process and findings.
Example output:
Duplicate packages found (info level):
- express
- versions: 4.17.1, 4.17.2
- mongoose
- versions: 5.10.9, 5.11.0
Use case 6: Output duplicate information in JSON format
Code:
npm find-dupes --json
Motivation:
Incorporating automation or scripting tools to analyze or take action based on duplicate dependencies often requires structured data inputs. A JSON format output from npm find-dupes
can easily be consumed by such tools, simplifying processes inside build scripts, CI/CD pipelines, or other automated workflows.
Explanation:
The --json
flag converts the output to a JSON format. This structured output is intended for machine parsing, allowing for easy integration into other software systems or scripts that process dependency information programmatically.
Example output:
{
"duplicates": [
{
"package": "react",
"versions": ["16.13.1", "16.14.0"]
},
{
"package": "react-dom",
"versions": ["16.13.1", "16.14.0"]
}
]
}
Use case 7: Limit duplicate search to specific scopes
Code:
npm find-dupes --scope=@scope1,@scope2
Motivation:
Large projects often utilize scoped packages to segregate dependencies into namespaces, particularly when using private npm registries. Limiting a duplicate search to specific scopes can be useful when maintaining or investigating specific subsystems or modules, ensuring focused attention to critical areas without being overwhelmed by unrelated information.
Explanation:
The --scope=@scope1,@scope2
argument restricts the duplication search to specified scopes. This approach allows users to effectively manage and analyze duplicates within a well-defined subset of their package ecosystem, reducing noise from unrelated components.
Example output:
Duplicate packages found in specified scopes:
- @scope1/util
- versions: 1.0.0, 1.1.0
- @scope2/helper
- versions: 2.3.0, 2.3.5
Use case 8: Exclude specific scopes from duplicate detection
Code:
npm find-dupes --omit-scope=@scope1,@scope2
Motivation:
Excluding certain scopes may be necessary if those packages are managed separately or if you trust their versions perfectly align across dependencies. This can also reduce the complexity and size of the search output when specific scopes are irrelevant to current optimizations or audits.
Explanation:
The --omit-scope=@scope1,@scope2
flag instructs the command to ignore specified scopes during the search for duplicates. It is beneficial when you are confident in the versioning consistency or relevance of these scopes to the matter at hand.
Example output:
Duplicate packages found excluding specified scopes:
- lodash
- versions: 4.17.20, 4.17.21
- axios
- versions: 0.19.0, 0.21.1
Conclusion:
By utilizing the npm find-dupes
command with the various options and parameters explained above, developers can efficiently manage and reduce unnecessary redundancy within their project’s dependencies. Through focused or comprehensive analysis approaches, the command assists in optimizing applications, maintaining consistent versioning, and supporting a more reliable and streamlined development environment.