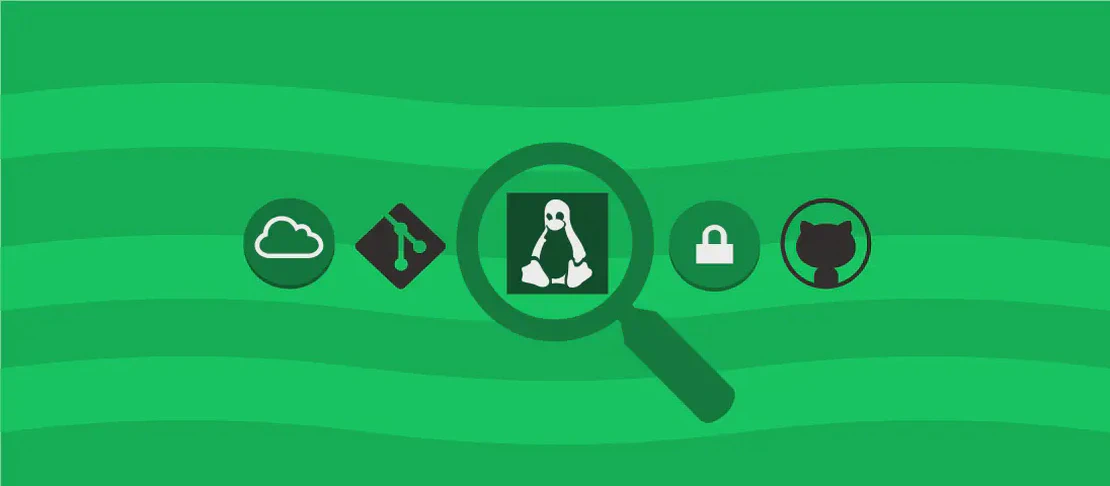
How to use the command 'npm init' (with examples)
The npm init
command is a crucial part of any Node.js development workflow. It is used to create a package.json
file, which serves as the manifest for your project. This file contains important metadata about the project, including the name, version, description, main file, scripts, license, and more. The initialization process can be tailored to prompt you for details or set default values automatically, easing the initial setup of a project.
Use case 1: Initialize a new package with prompts
Code:
npm init
Motivation:
Using the npm init
command without any flags is the most flexible and informative way to initialize a new Node.js project. This interactive approach renders a series of prompts guiding you through setting up the package.json
file according to your specific requirements. Whether you’re a beginner needing guidance or an experienced developer needing precision for a complex project, this approach ensures that you consciously set each parameter rather than relying on defaults that may not be appropriate for every project.
Explanation:
npm
stands for Node Package Manager, which is a tool used to manage dependencies for a Node.js project.init
is a command that initiates the setup to create a newpackage.json
file interactively.
Example Output:
This utility will walk you through creating a package.json file.
It only covers the most common items, and tries to guess sensible defaults.
See `npm help init` for definitive documentation on these fields
and exactly what they do.
Press ^C at any time to quit.
package name: (your-project)
version: (1.0.0)
description: A brief description of your project
entry point: (index.js)
test command:
git repository:
keywords:
author:
license: (ISC)
About to write to /Users/you/your-project/package.json:
{…}
Is this OK? (yes)
Use case 2: Initialize a new package with default values
Code:
npm init -y
Motivation:
For many developers, especially those prototyping or creating simple projects, spending time entering each field manually isn’t necessary. When you want to skip the interactive questionnaire and establish a project quickly, using the -y
flag initializes a new package.json
file with default settings. This setup is ideal for when time is of the essence, and finer details can be refined later.
Explanation:
-y
or--yes
automatically responds ‘yes’ to all the prompts innpm init
. This flag bypasses the questionnaire and generates thepackage.json
file using default values.
Example Output:
{
"name": "default-project",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Use case 3: Initialize a new package using a specific initializer
Code:
npm init create-react-app my-app
Motivation:
When building specific kinds of projects, like React applications, using initializers helps streamline setup with tailored configurations and dependencies suited for that type of project. The command npm init create-react-app my-app
serves as a shortcut for creating a new React application set up according to best practices. This approach not only saves developers from manual configurations but also ensures that all necessary tools and boilerplate code are correctly implemented from the start.
Explanation:
create-react-app
is a specific initializer provided by the React community, which sets up a new React app with zero configuration required.my-app
specifies the directory name where the React app will reside. If the directory does not exist, it will be created.
Example Output:
Creating a new React app in /path/to/my-app.
Installing packages. This might take a couple of minutes.
Installing react, react-dom, and react-scripts with cra-template...
...
Success! Created my-app at /path/to/my-app
Inside that directory, you can run several commands:
npm start
Starts the development server.
npm run build
Bundles the app into static files for production.
npm test
Starts the test runner.
npm run eject
Removes this tool and copies build dependencies, configuration files
and scripts into the app directory. If you do this, you can’t go back!
We suggest that you begin by typing:
cd my-app
npm start
Happy hacking!
Conclusion:
The npm init
command offers versatile options to set up a new Node.js project, catering to both detailed, interactive users and those seeking quick, default setups. Whether generating a basic package.json
file, initializing with predefined defaults, or leveraging specific initializers for frameworks like React, npm init
provides a comprehensive, streamlined approach to kickstart development efficiently. Understanding these use cases and their implementations empowers developers to manage project metadata effectively and crucially aids in efficient project lifecycle management from the outset.