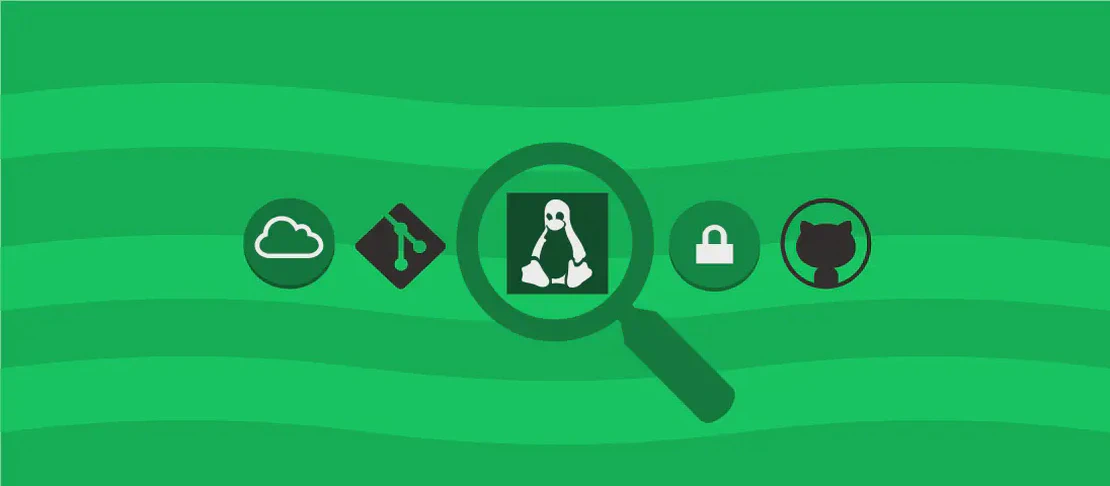
How to use the command 'npm ls' (with examples)
The npm ls
command is a versatile tool that belongs to the npm (Node Package Manager) ecosystem. It is primarily used to print installed packages and their dependencies to standard output (stdout). By using different flags, developers can customize the output to include additional information or change the format of the data. This flexibility can be quite useful when trying to understand the structure of dependencies in a Node.js project.
Use case 1: Print all versions of direct dependencies to stdout
Code:
npm ls
Motivation:
Understanding which versions of direct dependencies are currently installed can be crucial for debugging or ensuring compatibility in a project. By listing these versions, a developer can quickly verify that the right dependencies are in use without delving into the detailed hierarchy of nested dependencies.
Explanation:
In this instance, the command npm ls
is executed without any additional flags, which results in a simple tree structure being displayed. This output shows the direct dependencies of the project along with their versions. It does not descend into the sub-dependencies, making it useful for a high-level overview.
Example output:
my-project@1.0.0 /path/to/my/project
├── express@4.17.1
├── mongoose@5.9.10
└── react@16.13.1
Use case 2: Print all installed packages including peer dependencies
Code:
npm ls --all
Motivation:
In some cases, it’s essential to see not only the direct dependencies but all the dependencies, including peer dependencies and their hierarchy. This complete picture is indispensable when resolving complex dependency-related issues that might involve deep nesting or mismatches.
Explanation:
The --all
flag is an addition to the npm ls
command that forces npm to display all dependencies of all modules at every level. It provides a comprehensive view into everything that gets installed with your package, thus making it an invaluable debugging tool.
Example output:
my-project@1.0.0 /path/to/my/project
├─┬ express@4.17.1
│ ├── accepts@1.3.7
│ ├── array-flatten@1.1.1
│ └── body-parser@1.19.0
├─┬ mongoose@5.9.10
│ ├── async@3.1.0
│ ├── bson@1.1.4
│ └── kareem@2.3.1
├─┬ react@16.13.1
│ ├── loose-envify@1.4.0
│ └── scheduler@0.19.1
Use case 3: Print dependencies with extended information
Code:
npm ls --long
Motivation:
Sometimes, more detailed information about each dependency is necessary, such as its path or the package’s author. This level of detail can assist developers in understanding more about the dependencies, which can be helpful in an audit or review process.
Explanation:
By including the --long
flag, each package listed by the npm ls
command will provide extended information, typically including package description, repository URL, author, and more. This detailed knowledge can be critical for deeply understanding the context and origin of each package.
Example output:
my-project@1.0.0 /path/to/my/project
└── express@4.17.1
Package: express
Version: 4.17.1
Repository: https://github.com/expressjs/express
Author: TJ Holowaychuk <tj@vision-media.ca>
Use case 4: Print dependencies in parseable format
Code:
npm ls --parseable
Motivation:
When the output of the list command is to be consumed by a script or further processed by a software tool, having a parse-friendly format is crucial. This use case is designed for such situations where integration or automation requires a simplified text output that scripts can easily handle.
Explanation:
The --parseable
flag switches the output into a format that’s easier to parse programmatically. It removes any tree formatting and provides paths to each package in the dependency tree, which is useful for input into other scripts or tools.
Example output:
/path/to/my/project
/path/to/my/project/node_modules/express
/path/to/my/project/node_modules/mongoose
/path/to/my/project/node_modules/react
Use case 5: Print dependencies in JSON format
Code:
npm ls --json
Motivation:
JSON format is ubiquitous in data interchange within the programming world today, especially with web services and APIs. Using npm ls
to output dependency data in JSON format makes it easy to manipulate and analyze using tools or languages that support JSON.
Explanation:
The --json
flag is an essential option for users who want the output in a structured JSON format. This format provides a hierarchical object structure of dependencies, suitable for further processing, storage, or analysis with JSON-capable tools.
Example output:
{
"name": "my-project",
"version": "1.0.0",
"dependencies": {
"express": {
"version": "4.17.1"
},
"mongoose": {
"version": "5.9.10"
},
"react": {
"version": "16.13.1"
}
}
}
Conclusion:
The npm ls
command is a powerful tool for Node.js developers working with the npm package ecosystem. Its versatility, demonstrated through various use cases and options, allows developers to dive deeply into dependency management, enhancing project health and reliability through better understanding and clarity.