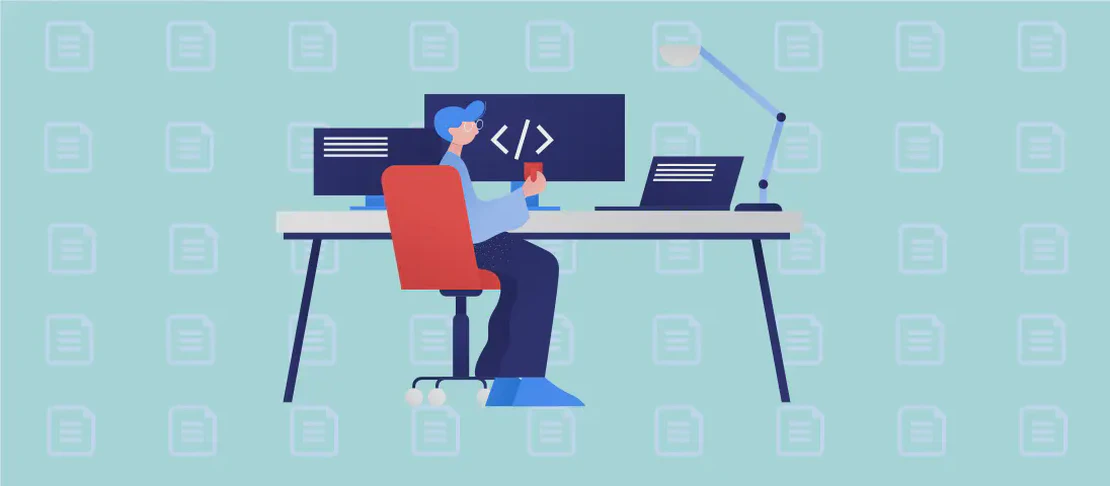
How to Use the Command 'npm run' (with examples)
The npm run
command is a feature offered by Node Package Manager (NPM) which empowers developers to execute scripts directly from the npm command line interface. This functionality is essential for automating tasks in JavaScript projects, helping in build processes, tests, or even starting server applications. By using npm run
, developers can easily standardize procedures across their projects, enhancing workflow efficiency and collaboration. Each script defined in a project’s package.json
file can be run with specific commands, ensuring flexibility and precision.
Use Case 1: Run a Script
Code:
npm run script_name
Motivation:
This use case arises when you have a defined set of tasks that you want to automate. For instance, suppose you’re working on a project where you need to transpile your code from a modern JavaScript version to one that’s more widely supported. By defining a script called, say, build
, you can streamline this process into a single command. Running this script saves time and ensures that the correct commands are executed in the right sequence every time.
Explanation:
npm
: Invokes the Node Package Manager, which is an essential tool in any JavaScript development environment.run
: Directs npm to run one of the custom scripts defined in thepackage.json
file.script_name
: Placeholder for the actual script name, specified in your project’spackage.json
under the “scripts” section. For instance, it could bebuild
if you’re compiling your code.
Example output:
> my-project@1.0.0 build
> webpack
Hash: 3ad98269fdf9b3
Version: webpack 4.39.3
Time: 2354ms
Built at: 09/28/2019 10:42:51 AM
Use Case 2: Pass Arguments to a Script
Code:
npm run script_name -- argument --option
Motivation:
There are scenarios where scripts require dynamic input to alter their behavior, such as passing environment-specific configurations. This usage is invaluable when your script needs to react to different environments (development, production) or special cases without altering the main script code. It expands the flexibility of your npm scripts by tailoring their execution to current needs.
Explanation:
npm
: Similar invocation as before.run
: Specifies the command to execute the given script.script_name
: The custom script identifier from thepackage.json
.--
: Signals to npm that what follows are arguments and options meant for the script.argument
: Represents positional arguments specific to the script’s execution.--option
: Denotes options that modify script behavior.
Example output:
> my-project@1.0.0 build
> webpack --mode development
Hash: 783b52df76a
Version: webpack 4.39.3
Time: 2420ms
Built at: 09/28/2019 10:47:21 AM
Use Case 3: Run a Script Named start
Code:
npm start
Motivation:
The start
script is a convention used in npm projects to define how to start the main application. It is often used to initiate servers, making this a critical use case for backend developers or anyone running long-lived services. Since npm start
is a special case that doesn’t require the run
keyword, it simplifies the command, assuming the project’s primary start-up process.
Explanation:
npm
: Standard Node Package Manager invocation.start
: This predefined script name is treated specially by npm, executing normally without the explicitrun
prefix.
Example output:
> my-project@1.0.0 start
> node server.js
Server running at http://localhost:3000/
Use Case 4: Run a Script Named stop
Code:
npm stop
Motivation:
The ability to stop a process that was started, especially in development, helps developers manage resources effectively. Whether you’re stopping a server or ending a task, this script ensures processes can be halted cleanly and consistently.
Explanation:
npm
: As with all the commands, this starts the Node Package Manager.stop
: This script name potentially stops running services or processes related to the application in its defined manner.
Example output:
> my-project@1.0.0 stop
> kill -9 $(lsof -t -i:3000)
Server stopped successfully.
Use Case 5: Run a Script Named restart
Code:
npm restart
Motivation:
Restarting is crucial for applying code changes without initiating every component separately. This use case is beneficial in development environments where applications are frequently modified, allowing work to proceed with minimal downtime.
Explanation:
npm
: Utilizes npm to manage node project scripts.restart
: Combinesstop
andstart
to halt and relaunch the application scripts, ensuring all new code changes are in effect.
Example output:
> my-project@1.0.0 restart
> npm stop && npm start
> my-project@1.0.0 stop
> kill -9 $(lsof -t -i:3000)
Server stopped successfully.
> my-project@1.0.0 start
> node server.js
Server running at http://localhost:3000/
Use Case 6: Run a Script Named test
Code:
npm test
Motivation:
Ensuring code quality through tests is a staple of modern software development practices. This script is commonly employed to automate the running of units and integration tests, often occurring frequently enough to warrant this simple, efficient command. Regular testing reinforces confidence in code reliability and correctness.
Explanation:
npm
: Again, the node environment’s package manager.test
: As a default, whennpm test
is executed, it automatically looks for and runs tests as per thetest
script entry in yourpackage.json
.
Example output:
> my-project@1.0.0 test
> jest
PASS ./sum.test.js
✓ adds 1 + 2 to equal 3 (5ms)
Conclusion:
Understanding and leveraging the npm run
command unlocks a potent layer of operational efficiency within JavaScript projects. Whether launching applications, testing code, or handling dynamic scripts with arguments, npm run
provides a unified interface to manage and automate various development tasks effectively, fostering both increased productivity and streamlined workflow.