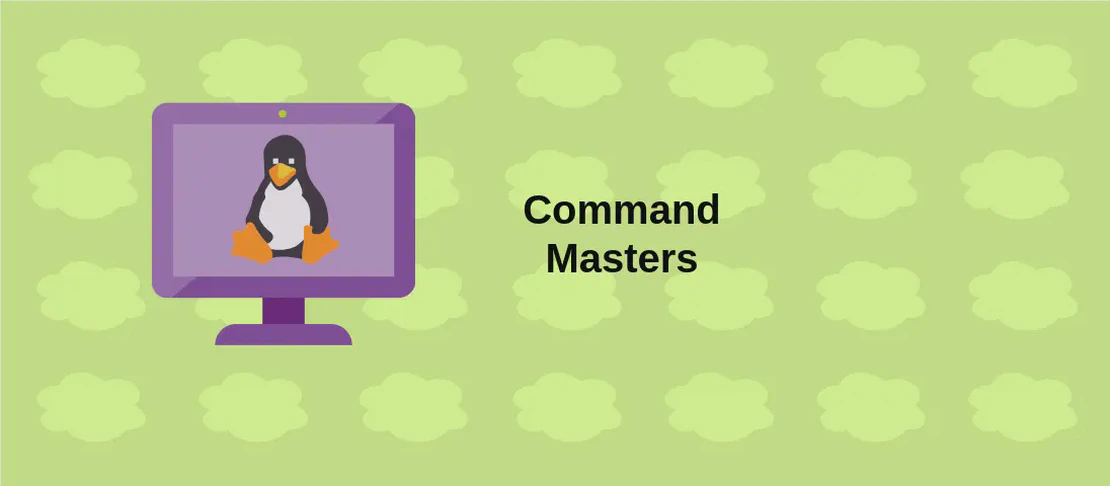
How to use the command 'npm star' (with examples)
The npm star
command is part of the Node Package Manager (npm) CLI toolset. It allows developers to mark packages as favorites in the npm registry. By starring a package, developers can express their appreciation for a package, making it easier to find and keep track of packages they find especially useful or noteworthy. This command is particularly beneficial for those who regularly work with JavaScript and Node.js projects, as it can streamline the process of managing and curating preferred packages.
Use case 1: Star a public package from the default registry
Code:
npm star package_name
Motivation: When using npm frequently, it is helpful to mark packages you find essential or plan to use continually. By starring a public package from the default registry, you can quickly access it later without needing to remember its exact name among the thousands available.
Explanation:
npm
: This is the command-line interface to interact with Node Package Manager, a crucial tool for JavaScript development.star
: This subcommand is used to mark a package as a favorite.package_name
: Replace this with the actual name of the package you wish to star.
Example output:
⭐ Marked package_name as a favorite!
Use case 2: Star a package within a specific scope
Code:
npm star @scope/package_name
Motivation: Scoped packages are typically used by organizations or developers to namespace their packages, ensuring no overlap with publicly available names. Starring a package within a specific scope is useful for keeping track of packages from a particular organization or project.
Explanation:
npm
: The Node Package Manager command-line tool.star
: Command to mark a package as a favorite.@scope/package_name
: This syntax specifies a package within a specific scope, wherescope
is the namespace andpackage_name
is the package you want to star.
Example output:
⭐ Marked @scope/package_name as a favorite!
Use case 3: Star a package from a specific registry
Code:
npm star package_name --registry=registry_url
Motivation: Different organizations might host their own npm registries, especially for private packages. This command is beneficial for maintaining a curated list of favorite packages from specific registries that are not part of the default npm ecosystem.
Explanation:
npm
: The Node Package Manager’s command interface.star
: Used here to favorite a package.package_name
: The name of the package to be starred.--registry=registry_url
: This flag specifies a particular registry URL from which the package should be starred, directing npm to look beyond the official npm repository.
Example output:
⭐ Marked package_name from registry registry_url as a favorite!
Use case 4: Star a private package that requires authentication
Code:
npm star package_name --auth-type=legacy|oauth|web|saml
Motivation: Many companies maintain private npm packages that require authentication to access. This command example is crucial for those instances, ensuring that the user is appropriately authenticated while starring a private package.
Explanation:
npm
: Command-line utility for interacting with packages.star
: Command used for marking the desired package as a favorite.package_name
: The specific private package to star.--auth-type=legacy|oauth|web|saml
: This option specifies the method for authentication. Different methods (legacy, oauth, web, saml) cater to various security and authentication setups, allowing flexibility based on organizational requirements.
Example output:
⭐ Authenticated and marked package_name as a favorite!
Use case 5: Star a package by providing an OTP for two-factor authentication
Code:
npm star package_name --otp=otp
Motivation: Security is enhanced in many systems through two-factor authentication (2FA), and the npm ecosystem supports this for protecting user accounts. When a package requires 2FA, one can star it by supplying the one-time password (OTP), thereby ensuring that only authenticated users can perform actions.
Explanation:
npm
: Command-line tool for managing npm packages.star
: Used to mark the specified package as favored.package_name
: Name of the package you wish to star.--otp=otp
: This flag is used to pass the one-time password necessary for completing actions that require higher security measures, which guards against unauthorized access.
Example output:
⭐ Marked package_name as a favorite using OTP!
Use case 6: Star a package with detailed logging
Code:
npm star package_name --loglevel=verbose
Motivation: When performing actions in npm, sometimes detailed feedback is necessary to ensure that everything is proceeding correctly or to debug issues. Using a verbose log level offers comprehensive output about the process, useful for developers who need to closely monitor operations or troubleshoot problems.
Explanation:
npm
: Node’s package manager CLI tool.star
: The command to highlight a package as a favorite.package_name
: Name of the package you aim to star.--loglevel=verbose
: This option enables detailed logging, increasing the verbosity of output messages to include more information about the process undertaken by thenpm star
command.
Example output:
npm info it worked if it ends with ok
npm verb cli [<full CLI command>]
...
npm verbose star package_name
⭐ Marked package_name as a favorite!
...
npm info ok
Use case 7: List all your starred packages
Code:
npm star --list
Motivation: As you use npm over time, the list of packages you star can grow significantly. Listing all your starred packages is an efficient way to review which packages you have marked as favorites, potentially re-evaluating and re-organizing them as needed.
Explanation:
npm
: Command-line interface to the npm package ecosystem.star
: Subcommand for managing favorite packages.--list
: This option directs the command to list all packages you have previously starred, offering a comprehensive view of your favorites.
Example output:
List of your starred packages:
- express
- lodash
- react
Use case 8: List your starred packages from a specific registry
Code:
npm star --list --registry=registry_url
Motivation: When working with multiple registries, especially private ones, you might want to separately list packages starred from each specific registry. This capability is crucial when dealing with different environments, projects, or organizational spaces.
Explanation:
npm
: The CLI tool for Node’s package manager.star
: Used for performing actions related to favorite packages.--list
: Instructs the command to display packages you’ve marked.--registry=registry_url
: Specifies the registry from which you want to list your starred packages, valuable for isolating lists from various registries.
Example output:
List of your starred packages from registry registry_url:
- private-package-1
- custom-utils-package
Conclusion:
The npm star command is a versatile tool that provides a simple way to mark packages as favorites, enabling users to manage and review their preferred packages across public and private registries. By utilizing options for scope, authentication, OTP, verbosity, and registry specification, developers can customize the operation to fit their specific needs and security requirements. This functionality plays a vital role in organizing and accessing critical software components in the Node.js ecosystem.