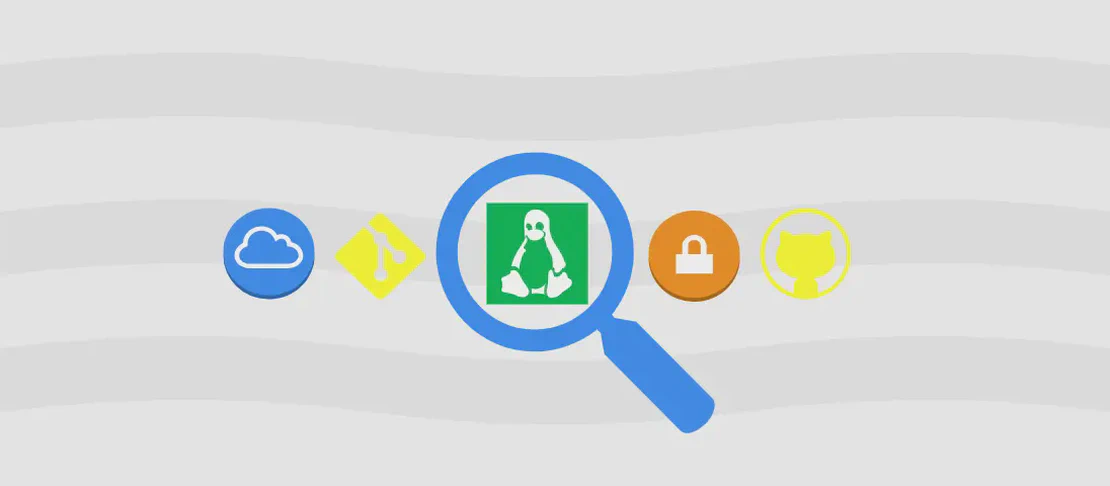
How to Use the Command 'npm version' (with examples)
The npm version
command is an essential tool for managing version numbers within Node.js package development. When developing Node.js packages, updating the version is a critical step to signal new updates, improvements, or bug fixes. This command not only updates the package.json
file with a new version but also optionally creates a Git tag to keep track of different versions. Here’s how to effectively use npm version
for various tasks.
Use Case 1: Check Current Version
Code:
npm version
Motivation:
Before making any changes to a package, it’s essential to know the current version. By running this command, you can quickly verify the existing version of your package as registered in your package.json
file. This ensures you have a baseline understanding before making any version increments or changes.
Explanation:
npm version
: This command, without any additional parameters, fetches the current version of your package as specified in thepackage.json
file. It’s a straightforward way to confirm your starting point before making modifications.
Example Output:
1.5.3
Use Case 2: Bump the Minor Version
Code:
npm version minor
Motivation:
Incrementing the minor version is a common practice when you’ve added backward-compatible functionalities to your package. Using this command ensures that users are informed of new features that won’t break existing functionality.
Explanation:
minor
: This argument tellsnpm
to increase the minor version number in yourpackage.json
file. Versioning conventions usually indicate that a minor version is associated with new features or improvements that are backward compatible.
Example Output:
v1.6.0
Use Case 3: Set a Specific Version
Code:
npm version 2.3.1
Motivation:
There might be occasions when you need to set an exact version for consistency or compatibility reasons. This could arise if you’re aligning with another part of a system, or adhering to an internal versioning policy.
Explanation:
2.3.1
: This argument specifies the exact version you wish to set in yourpackage.json
. It overrides the current version, allowing for precision control over versioning which can be critical in managing dependencies and maintaining compatibility.
Example Output:
v2.3.1
Use Case 4: Bump the Patch Version Without Creating a Git Tag
Code:
npm version patch --no-git-tag-version
Motivation:
There are instances where you want to release a bug fix or a minor patch without creating an accompanying Git tag. This might be necessary during a rapid series of fixes or a development sprint where Git tagging would be burdensome.
Explanation:
patch
: This tellsnpm
to increase the patch version in thepackage.json
, which typically involves bug fixes or small maintenance updates.--no-git-tag-version
: This flag suppresses the creation of a Git tag, focusing solely on the version bump in your project files without changing your Git history or creating unnecessary tags.
Example Output:
v1.5.4
Use Case 5: Bump the Major Version with a Custom Commit Message
Code:
npm version major -m "Upgrade to %s for reasons"
Motivation:
When making significant, potentially breaking changes, a major version increment is needed. It’s professionally prudent to include a descriptive commit message explaining the reason behind such changes. This practice is crucial for clarity in collaborative environments or projects with multiple contributors.
Explanation:
major
: This argument bumps the major version, indicating substantial changes that are likely not backward-compatible with previous versions.-m "Upgrade to %s for reasons"
: The-m
flag allows you to specify a custom commit message. The%s
placeholder is replaced by the new version number, providing clear documentation within the commit history about why the major version increment occurred.
Example Output:
v2.0.0
Conclusion:
The npm version
command is a versatile tool for managing the lifecycle of Node.js projects. By understanding how to use it for various scenarios—from checking current versions to making specific version changes—you can maintain a clean, coherent versioning history. This approach helps facilitate clearer communication and version control, contributing significantly to project stability and reliability.