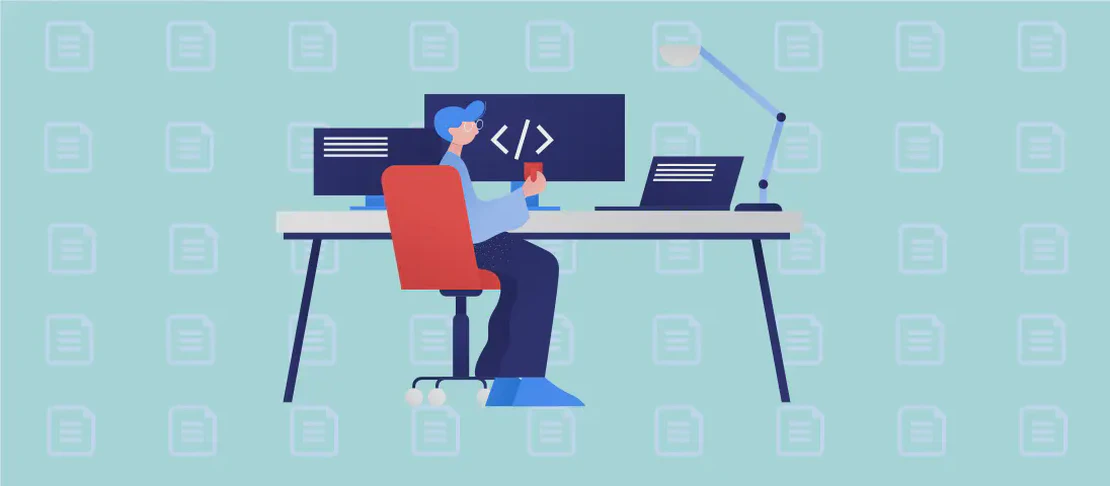
Utilizing the 'npx' Command (with examples)
npx
is a tool that comes with npm
(Node Package Manager). It’s designed to facilitate the ease of executing binary scripts directly from packages without having to globally install them. This allows developers to quickly and efficiently run scripts from packages, manage versions, and execute code across various environments. By leveraging npx
, you can reduce the clutter of globally installed packages and maintain a cleaner and more manageable development setup.
Use case 1: Execute the command from a local or remote npm
package
Code:
npx create-react-app my-app
Motivation:
Using npx
to execute a package command directly is extremely convenient, especially when you want to use a package one time or try it before deciding to install it permanently. In this example, we want to create a new React application quickly without having to install create-react-app
globally.
Explanation:
npx
: The command-line tool that executes the requested binary files.create-react-app
: The name of the package or application we want to run.my-app
: This is an argument passed tocreate-react-app
, specifying the name of the directory where the new React application will be set up.
Example output:
Creating a new React app in /your-path/my-app.
Installing packages. This might take a couple of minutes.
Installing react, react-dom, and react-scripts...
...
Use case 2: Specify the package explicitly when multiple commands have the same name
Code:
npx --package cowsay cowsay Hello, world!
Motivation:
This use case is particularly helpful when you have multiple packages that may contain executables with the same name. By explicitly stating which package your command should come from, you avoid ambiguity and ensure the correct command is executed, which can prevent errors and unexpected behavior.
Explanation:
npx
: Invokes the npx tool.--package cowsay
: Specifies that the command to execute,cowsay
, should come from thecowsay
package.cowsay Hello, world!
: The actual command, withHello, world!
being the string we wantcowsay
to display in its output.
Example output:
______________
< Hello, world! >
--------------
\ ^__^
\ (oo)\_______
(__)\ )\/\
||----w |
|| ||
Use case 3: Run a command if it exists in the current path or in node_modules/.bin
Code:
npx --no-install serve
Motivation:
When you want to run a command quickly without installing it globally or fetching it from a remote registry, using --no-install
ensures that npx
only looks for the command locally within existing paths or in node_modules/.bin
. This is efficient for frequently used local commands and helps speed up development by avoiding unnecessary network requests.
Explanation:
npx
: The base tool being utilized.--no-install
: A flag to preventnpx
from attempting to fetch or install packages from the npm registry.serve
: The command to execute, if it can be found locally.
Example output:
INFO: Accepting connections at http://localhost:3000
Use case 4: Suppress output from npx
itself when executing a command
Code:
npx --quiet eslint myfile.js
Motivation:
Sometimes, you might want to streamline the command-line output to be less verbose, especially in scripts or automation processes. With --quiet
, you suppress any non-essential output from npx
, making the console display only the necessary information, such as errors or warnings related to the command being run.
Explanation:
npx
: Initiates the npx execution process.--quiet
: Tellsnpx
to suppress its default verbosity.eslint
: The command being executed to analyze your JavaScript file.myfile.js
: The specific file being linted byeslint
.
Example output:
/path/to/myfile.js
1:1 error 'myVar' is assigned a value but never used no-unused-vars
✖ 1 problem (1 error, 0 warnings)
Use case 5: Display help
Code:
npx --help
Motivation:
Accessing detailed information about npx
and its various options and syntaxes is crucial for both new users and experienced developers. The --help
command is an invaluable resource for learning about all capabilities and parameters that npx
offers, fostering a deeper understanding and adept use in more complex scenarios.
Explanation:
npx
: The command tool you’re seeking help about.--help
: This flag signals that you want to access the help documentation ofnpx
.
Example output:
Usage:
npx [options] <command>[args...]
Options:
--package <pkg> Package name to resolve
--quiet Suppress output from npx itself
--no-install Use local if present, else abort
--help Show help for npx
...
Conclusion:
The npx
command serves as a highly flexible tool for developers, streamlining the execution of npm package commands without the weight of global installations. Whether configuring new projects, navigating multiple executable scenarios, or optimizing command execution locally, npx
plays a valuable role in simplifying modern JavaScript development workflows. Embracing these use cases can substantially enhance productivity and reduce complications in managing JavaScript projects of varying scales.