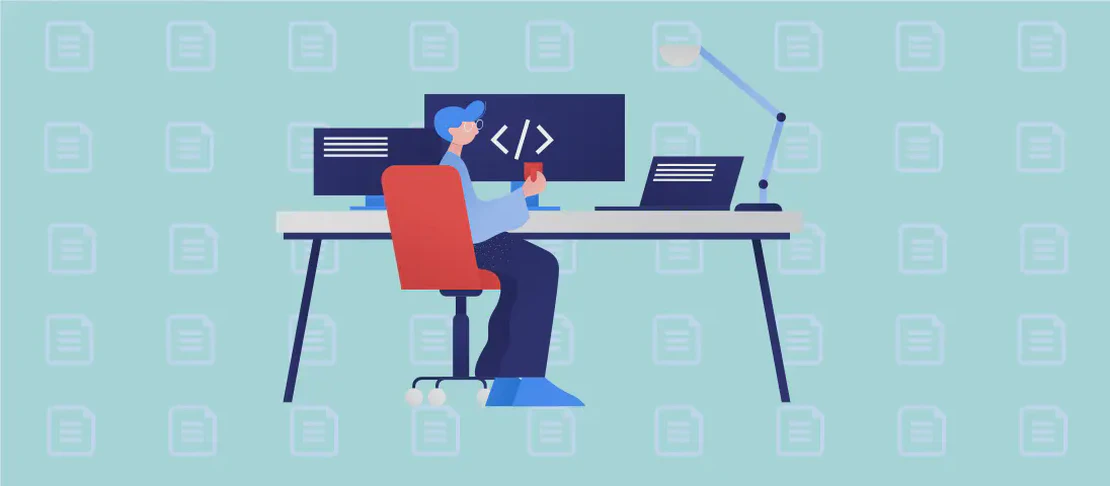
How to use the NVIDIA CUDA Compiler Driver `nvcc` (with examples)
The NVIDIA CUDA Compiler Driver, commonly referred to as nvcc
, is a core component for programmers working with NVIDIA’s CUDA platform. CUDA is a parallel computing architecture that utilizes the extraordinary computing power of NVIDIA’s GPUs to deliver incredibly high performance for computationally intensive applications. The nvcc
command is crucial as it transforms CUDA code into executable binaries that can run on NVIDIA GPUs. This article explores various use cases of the nvcc
command, each demonstrating its versatility and functionality in different contexts.
Compile a CUDA program
Code:
nvcc path/to/source.cu -o path/to/executable
Motivation:
This is the fundamental use case where a CUDA program written in a source file (with a .cu
extension) is compiled into an executable. This process is essential for converting human-readable code into a format that machines can execute. The ease with which nvcc
handles this task makes it the backbone of development in CUDA-driven environments, enabling developers to test, implement, and refine their algorithms on NVIDIA GPUs.
Explanation:
nvcc
: Invokes the NVIDIA CUDA Compiler Driver to begin the compilation process.path/to/source.cu
: The path to the CUDA source file that contains the program logic.-o path/to/executable
: Specifies the output path and name for the compiled executable file.
Example Output:
After running the command, an executable binary file will be created at the specified output location. This file can be executed to run the CUDA program using an NVIDIA GPU.
Generate debug information
Code:
nvcc path/to/source.cu -o path/to/executable --debug --device-debug
Motivation:
Debugging is an integral part of software development, allowing developers to identify and fix errors within their code. In CUDA programming, where errors can also involve the complex interaction of both the GPU and CPU, comprehensive debugging information is critical. This example showcases how nvcc
can generate detailed debug details that assist in troubleshooting and optimizing CUDA programs.
Explanation:
nvcc
: Starts the compilation process.path/to/source.cu
: Path to the CUDA program that is to be compiled.-o path/to/executable
: Designates the output file for the executable.--debug
: Directsnvcc
to include debugging information in the output.--device-debug
: Extends debugging details to cover the CUDA devices, showing information relevant to GPU executed portions of the program.
Example Output:
The output will be a debuggable executable, with enhanced data that can be used by CUDA-compatible debuggers to analyze and step through the application.
Include libraries from a different path
Code:
nvcc path/to/source.cu -o path/to/executable -Ipath/to/includes -Lpath/to/library -llibrary_name
Motivation:
Complex CUDA programs often rely on external libraries to access pre-written functions and tools that save time and effort. Including libraries from varied locations is necessary when these resources are not situated in standard paths. This command helps manage dependencies effectively by including specific library paths and names, streamlining development processes.
Explanation:
nvcc
: Launches the compilation process.path/to/source.cu
: Specifies the CUDA source file to be compiled.-o path/to/executable
: Determines where the final executable will reside and its associated name.-Ipath/to/includes
: Adds a directory to the list of paths to search for header files during compilation.-Lpath/to/library
: Adds a directory to the library search path for resolving external dependencies.-llibrary_name
: Links against a specific library that provides needed functionalities.
Example Output:
The output is an executable that correctly links with external libraries, enabling full access to the required features and functions defined in the included paths.
Specify the compute capability for a specific GPU architecture
Code:
nvcc path/to/source.cu -o path/to/executable --generate-code arch=arch_name,code=gpu_code_name
Motivation:
Different GPUs have diverse capabilities, a factor known as compute capability. By specifying the compute capability, developers ensure that their applications are optimized and fully functional on targeted GPU architectures. This is especially important for achieving optimal performance and compatibility when running applications on varying types of NVIDIA GPUs.
Explanation:
nvcc
: Initiates the compiling sequence.path/to/source.cu
: Path to the source code file requiring compilation.-o path/to/executable
: Location and name of the executable result.--generate-code arch=arch_name,code=gpu_code_name
: Specifies the architecture and the GPU code generation options.arch_name
refers to the architecture target, whilegpu_code_name
specifies the instruction set for a specific GPU family.
Example Output:
The compiled application will be tailor-made for the specified GPU architecture, ensuring it operates efficiently on the intended hardware.
Conclusion:
The nvcc
command is indispensable for CUDA developers working with NVIDIA GPUs, offering extensive capabilities beyond simple compilation. From debugging to linking libraries and targeting specific hardware architectures, nvcc
provides a comprehensive toolkit for developers seeking to harness the power of parallel processing with CUDA. Through these examples, the versatility and critical role of nvcc
in CUDA development are clearly highlighted, equipping developers with the knowledge needed to effectively build and optimize GPU-accelerated applications.