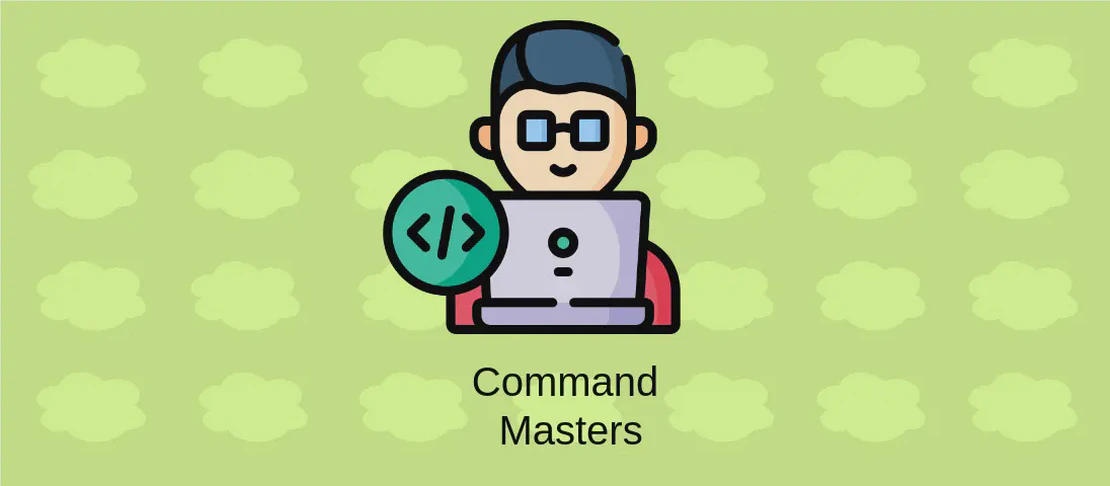
How to use the command `nvm` (with examples)
The nvm
command is a tool that allows you to easily manage different versions of Node.js on your system. It provides a simple way to install, uninstall, and switch between different versions of Node.js. With nvm
, you can install specific versions of Node.js, set a default version, and run scripts or REPLs with specific versions.
Use case 1: Install a specific version of Node.js
Code:
nvm install node_version
Motivation: You may want to install a specific version of Node.js to ensure compatibility with a particular project or to try out new features available in a specific version.
Explanation: The nvm install
command is used to install a specific version of Node.js. You need to specify the version number or label you want to install. For example, nvm install 12.8
or nvm install v16.13.1
will install the specified Node.js version.
Example output:
...
Downloading and installing node v16.13.1...
...
Use case 2: Use a specific version of Node.js in the current shell
Code:
nvm use node_version
Motivation: You may have multiple versions of Node.js installed on your system and want to switch between them. This can be useful when working on different projects that require different versions.
Explanation: The nvm use
command is used to switch to a specific version of Node.js in the current shell. You need to specify the version number or label you want to use. For example, nvm use 12.8
or nvm use v16.13.1
will switch to the specified Node.js version.
Example output:
Now using node v16.13.1 (npm v8.1.0)
Use case 3: Set the default Node.js version
Code:
nvm alias default node_version
Motivation: You may want to set a default Node.js version that will be used whenever a new shell is opened. This can be useful if you have a preferred version or if you want to ensure consistency across different projects.
Explanation: The nvm alias
command is used to set an alias for a specific Node.js version. By setting an alias named “default” to a specific version, that version will be used whenever a new shell is opened without explicitly specifying a version.
Example output:
default -> 12.8 (-> v12.8.1)
Use case 4: List all available Node.js versions and highlight the default one
Code:
nvm list
Motivation: You may want to see a list of all the Node.js versions installed on your system. This can be useful to verify the versions available and to check which version is currently set as the default.
Explanation: The nvm list
command lists all available Node.js versions installed on your system. It also highlights the version that is currently set as the default.
Example output:
...
10.24.1
-> 12.8 (-> v12.8.1)
14.17.6
16.13.1
system
stable
...
Use case 5: Uninstall a given Node.js version
Code:
nvm uninstall node_version
Motivation: You may want to remove a specific version of Node.js from your system. This can be useful if you no longer need that version or if you want to free up disk space.
Explanation: The nvm uninstall
command is used to uninstall a specific version of Node.js. You need to specify the version number or label you want to uninstall. For example, nvm uninstall 12.8
or nvm uninstall v16.13.1
will uninstall the specified Node.js version.
Example output:
...
Uninstalled node v16.13.1
...
Use case 6: Launch the REPL of a specific version of Node.js
Code:
nvm run node_version --version
Motivation: You may want to quickly launch a specific version of the Node.js REPL (Read-Eval-Print Loop) to test out code or explore features available in that version.
Explanation: The nvm run
command is used to launch a specific version of the Node.js REPL. You need to specify the version number or label you want to launch, followed by the --version
flag to display the version information.
Example output:
...
v12.8.1
...
Use case 7: Execute a script in a specific version of Node.js
Code:
nvm exec node_version node app.js
Motivation: You may want to run a script using a specific version of Node.js. This can be useful when working on projects that require specific dependencies or need to be tested with different versions of Node.js.
Explanation: The nvm exec
command is used to execute a script in a specific version of Node.js. You need to specify the version number or label you want to use, followed by the command and script you want to execute. For example, nvm exec 12.8 node app.js
or nvm exec v16.13.1 node app.js
will execute the app.js
script using the specified Node.js version.
Example output:
...
Running app.js using node v12.8.1
...
Conclusion:
The nvm
command is a powerful tool for managing different versions of Node.js. It allows you to easily install, uninstall, and switch between versions, set defaults, and execute scripts or REPLs with specific versions. By using these examples, you can effectively manage your Node.js environment and ensure compatibility with different projects.