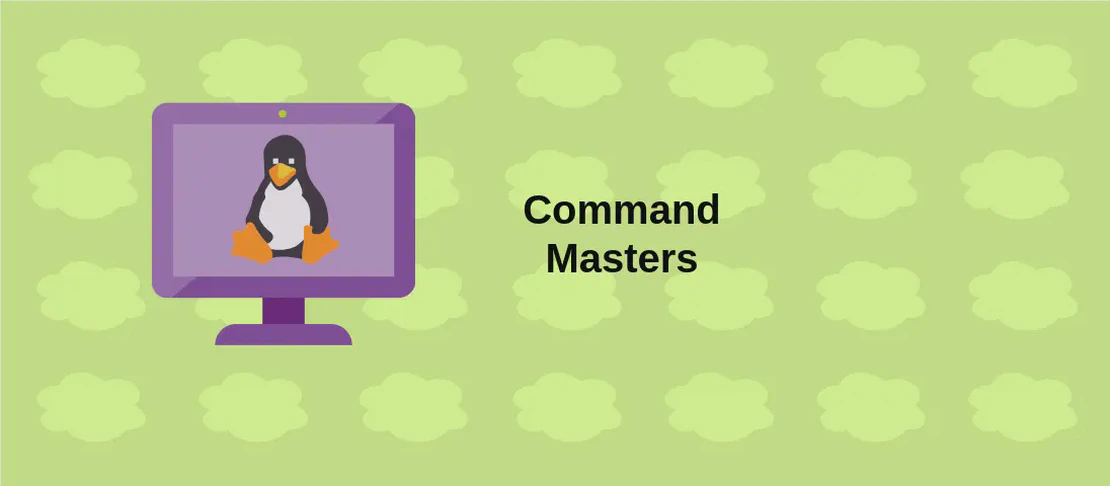
Exploring OCaml Command-Line Use Cases (with examples)
The OCaml command-line tool offers a versatile way to interact with OCaml, a functional programming language known for its expressive syntax and automatic memory management features. The “ocaml” command provides an interactive environment for learning and development in OCaml, and can also execute scripts directly. Below, we’ll delve into some common use cases of the OCaml command, offering a comprehensive exploration of how it can be utilized effectively.
Use case 1: Read OCaml commands from the user and execute them
Code:
ocaml
Motivation:
Using the OCaml Read-Eval-Print Loop (REPL) is an excellent way to test snippets of OCaml code, practice OCaml syntax, and rapidly experiment with new ideas without the need for compiling an entire program. The REPL provides immediate feedback, which makes it an invaluable tool for both learning and debugging.
Explanation:
ocaml
: This command alone enters the interactive session where users can input OCaml commands directly. It launches the OCaml REPL, which stands for Read-Evaluate-Print Loop. In this mode, users can enter OCaml expressions one by one. Each expression is read, evaluated, and the result is printed to the console.
Example Output:
OCaml version 4.12.0
# let x = 5 + 3;;
val x : int = 8
# print_string "Hello, OCaml!";;
Hello, OCaml!- : unit = ()
The output shows the result of evaluating OCaml expressions entered by the user, showcasing the environment’s ability to provide real-time computation results.
Use case 2: Read OCaml commands from a file and execute them
Code:
ocaml path/to/file.ml
Motivation:
Executing OCaml commands from a file is beneficial when managing larger codebases or scripts where typing the code interactively into the REPL would be inefficient. It offers a streamlined way to run complex OCaml programs stored in files, allowing for easier collaboration, version control, and repeated testing.
Explanation:
ocaml
: Calls the OCaml interpreter to start executing commands.path/to/file.ml
: Specifies the path to an OCaml script file, typically ending with the.ml
extension, signifying a source file. The interpreter reads this file and executes its contents sequentially as if they were entered directly in the REPL.
Example Output:
Assuming the file example.ml
contains the following OCaml code:
let greet name = "Hello, " ^ name ^ "!"
let () = print_endline (greet "World")
Executing ocaml example.ml
results in:
Hello, World!
This shows that the interpreter has successfully read the file, executed the script, and printed the greeting to the standard output.
Use case 3: Run OCaml script with modules
Code:
ocaml module1 module2 path/to/file.ml
Motivation:
Using external modules with an OCaml script allows the user to incorporate predefined functionalities and libraries, enhancing the flexibility and capability of simple scripts. This practice supports code reuse, modularity, and an organized approach to coding, making complex program construction more manageable.
Explanation:
ocaml
: Initiates the OCaml interpreter.module1
andmodule2
: Represents optional module names that can be included when executing the script. These might be compiled module interfaces or implementations that need to be referenced while running the file.path/to/file.ml
: Denotes the script file to execute. When specified with modules, it allows access to functionalities defined in these modules.
Example Output:
Consider having utils.ml
and main.ml
with the following contents:
utils.ml
:
let add x y = x + y
main.ml
:
let sum = Utils.add 10 20
let () = Printf.printf "The sum is: %d\n" sum
Running ocaml utils.cmo main.ml
should generate:
The sum is: 30
Here, utils.ml
needs to be compiled to a .cmo
file (OCaml object file) using ocamlc -c utils.ml
before being executed with the script. The command then uses the compiled .cmo
file (utils.cmo
) to resolve the Utils.add
call in main.ml
.
Conclusion:
The versatility of the OCaml command through its interpretive capabilities provides a robust mechanism for developing and testing programs. Whether through interacting directly with the REPL, executing scripts from files, or managing complex module-based projects, each use case enhances a developer’s proficiency in leveraging the OCaml language to its fullest potential. These tools cater to both beginners seeking to immerse themselves in a functional programming environment and seasoned developers managing intricate OCaml applications.