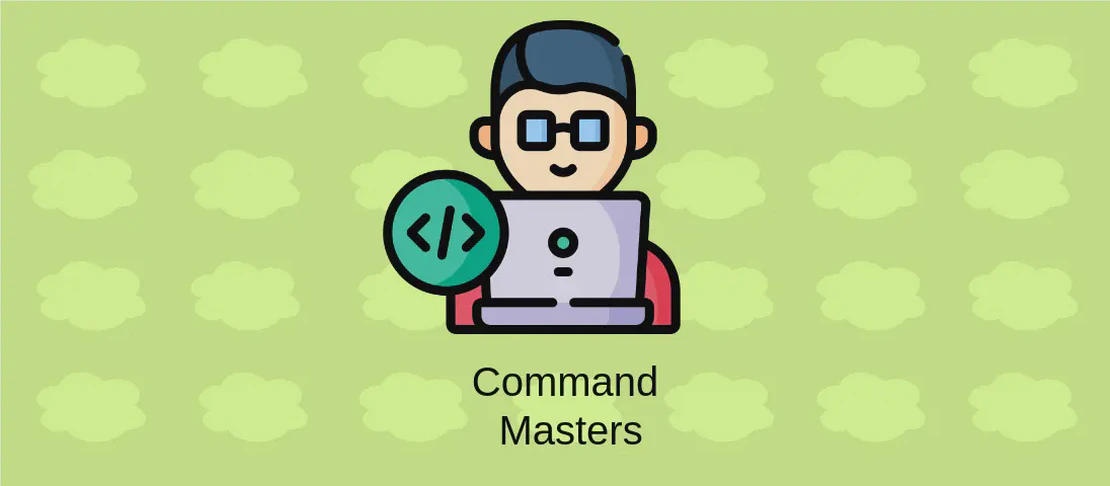
Using the OCaml Compiler 'ocamlc' (with examples)
The ocamlc
command is a crucial tool for OCaml programmers, serving as the bytecode compiler for the OCaml language. It is specifically designed to produce executables that can be run using the OCaml interpreter. By converting high-level OCaml code into bytecode, ocamlc
facilitates the development, testing, and execution of OCaml programs efficiently. More comprehensive details on OCaml and its compiler can be found at the official OCaml website.
Use case 1: Creating a Binary from a Source File
Code:
ocamlc path/to/source_file.ml
Motivation:
Generating a binary from an OCaml source file is a fundamental task for any OCaml developer who wants to transition from code to a runnable form. This use case illustrates the simplest scenario where the developer aims to compile a single OCaml source file, source_file.ml
, into an executable binary. By doing this, programmers can quickly test their application logic or share the executable with others for running without needing the source code or specific OCaml setup.
Explanation:
ocamlc
: This initializes the OCaml bytecode compiler, indicating that the command will involve compiling OCaml code into a binary.path/to/source_file.ml
: This specifies the file path to the OCaml source code that you want to compile. It tellsocamlc
which file to read, parse, and compile.
Example Output:
Upon execution, ocamlc
will compile the source_file.ml
and produce an output file, typically named a.out
by default. This output is an executable binary of the OCaml program. You can run this executable in the OCaml interactive environment using:
./a.out
Use case 2: Creating a Named Binary from a Source File
Code:
ocamlc -o path/to/binary path/to/source_file.ml
Motivation:
Often, developers prefer to have control over the name of the generated binary to enhance clarity, especially when dealing with multiple executables or different versions of a program. This use case is about specifying a custom name for the output binary file, rather than using the default. By naming output files in a meaningful way, project management becomes more streamlined and organized.
Explanation:
ocamlc
: As in the previous use case, this is the command that runs the OCaml bytecode compiler.-o path/to/binary
: The-o
flag stands for ‘output’. It allows the user to specify the desired path and name of the resulting binary file. This enhances flexibility and usability by allowing meaningful file names.path/to/source_file.ml
: Again, this represents the path to the source OCaml file that needs to be compiled into a binary.
Example Output:
This command will generate an executable binary named precisely as indicated in the path/to/binary
. For instance, if path/to/binary
is specified as my_program
, the output produces an executable file named my_program
, which can be run using:
./my_program
Use case 3: Automatically Generating a Module Signature (Interface) File
Code:
ocamlc -i path/to/source_file.ml
Motivation:
Generating a module signature file is particularly advantageous for developers who wish to define and clearly view the interfaces of their modules. OCaml modules play a significant role in encapsulating functionality and data types. This use case demonstrates how to automatically create a module signature, which outlines the public interface of a module. It is crucial for understanding and organizing code, especially in larger projects where modules must interact reliably.
Explanation:
ocamlc
: The command to engage the OCaml bytecode compiler, ready for operations on the OCaml code.-i
: This flag triggers the compiler to generate a module interface file, a.mli
file. It inspects the source files and outputs their signatures, showing the assumptions the module makes of itself and how it interacts externally.path/to/source_file.ml
: This signifies the source code file from which to generate the interface. The input file is parsed to determine the exposed and accessible parts of the code.
Example Output:
Running this command does not produce a binary. Instead, it returns a textual representation of the interface of the specified OCaml module directly in the terminal window. It lists the exposed functions, types, and values that other modules can interact with, helping developers grasp the module’s capabilities and constraints.
Conclusion:
The ocamlc
command is a versatile tool for compiling and interfacing with OCaml programs. Whether simply converting source files to executables, specifying custom names for output binaries, or eliciting the interface of a module, ocamlc
plays a vital role in the OCaml development workflow. Embracing these functionalities allows developers to write, organize, and share OCaml code more effectively.