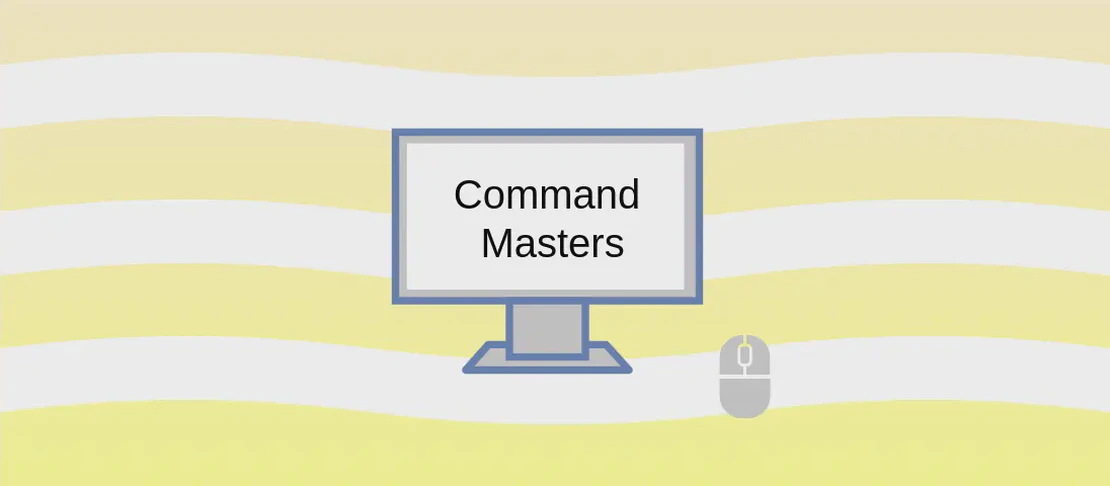
How to Effectively Use the 'ollama' Command (with examples)
The ‘ollama’ command is a powerful tool designed to facilitate interactions with large language models. By acting as a language model runner, it provides a systematic environment for deploying, managing, and customizing various models. This tool makes it significantly easier for users to access machine learning models for a range of applications, from basic conversation simulators to complex data analysis tasks. The following use cases illustrate how to utilize the ‘ollama’ command in various scenarios.
Use case 1: Start the daemon required to run other commands
Code:
ollama serve
Motivation:
The ‘ollama serve’ command is essential for setting up the necessary environment that allows other ‘ollama’ commands to function. By starting the daemon, you establish a groundwork server that can manage requests and processes related to language models. This is the first step to using ‘ollama’ effectively, ensuring that your system is prepared for deploying models without running into errors.
Explanation:
serve
: This command initiates the background process necessary for the ‘ollama’ utility to function properly, akin to initializing a service that awaits further commands or requests related to language models.
Example Output:
ollama daemon has been started and is running as a background process.
Use case 2: Run a model and chat with it
Code:
ollama run model
Motivation:
Running a model is integral when you want to engage directly with a language model, either for entertainment, educational purposes, or to support a business application. This command allows you to initiate a session where you can converse with the model in real time, leveraging its large corpus of learned information for various applications.
Explanation:
run
: This command signifies executing a specific model to make it active and ready for interaction.model
: This placeholder symbolizes the name of the specific language model you wish to operate on. It could be a general-purpose chat model or a specialized model for targeted tasks.
Example Output:
Model ready. Start your conversation now.
Use case 3: Run a model with a single prompt
Code:
ollama run model prompt
Motivation:
For cases where you need a quick response to a single query or when you want to automate a repetitive task, running a model with a single prompt is highly efficient. It allows for immediate utility from the model without entering into a prolonged interactive session. This is especially useful in scripting and batch processing contexts.
Explanation:
run
: Invokes the model to process information and produce an output.model
: Denotes the specific model being put to use.prompt
: The actual input or question you wish the model to respond to. It’s a direct message encapsulating your instructions for the model.
Example Output:
> User: What is the capital of France?
Response: The capital of France is Paris.
Use case 4: List downloaded models
Code:
ollama list
Motivation:
Listing downloaded models is a utility function that gives users an overview of what models are available locally. This is important for managing disk space and understanding which models can be readily utilized or updated. It’s particularly beneficial when dealing with many models because it helps in planning updates or removals.
Explanation:
list
: This command instructs ‘ollama’ to enumerate all the models that have been downloaded and are stored on your system.
Example Output:
Available models:
- model_A
- model_B
- model_C
Use case 5: Pull/Update a specific model
Code:
ollama pull model
Motivation:
The ‘ollama pull’ command is vital for ensuring that your models are up-to-date with the latest improvements and optimizations. This becomes crucial when fine-tuning performance, implementing new features, or ensuring that known bugs within a model have been resolved. Regular updates ensure interaction precision and the security of utilizing cutting-edge AI.
Explanation:
pull
: Fetches the latest version of a specified model from an external repository.model
: Specifies the name of the model you wish to update or download anew.
Example Output:
Pulling updates for model: model_A
Update complete.
Use case 6: List running models
Code:
ollama ps
Motivation:
In any multi-process environment, it is important to keep track of active processes to optimize performance and resource management. The ‘ps’ command allows users to see at a glance which models are currently running, aiding in debugging, workload management, and efficient resource utilization.
Explanation:
ps
: Stands for ‘process status’, this command lists all the models currently being run or interacting with the system.
Example Output:
Running models:
- model_B
Started at: 10:05 AM
Use case 7: Delete a model
Code:
ollama rm model
Motivation:
Over time, models that are no longer needed or due for replacement can clutter storage and leave systems vulnerable to inefficiencies. Deleting obsolete models is crucial for maintaining an organized system, freeing up storage, and avoiding redundancy. Managing models effectively can be a crucial part of ensuring that only the best and most necessary models are kept accessible.
Explanation:
rm
: Short for ‘remove’, this command deletes the specified model from local storage.model
: Represents the model targeted for deletion.
Example Output:
Model model_C has been removed.
Use case 8: Create a model from a Modelfile
Code:
ollama create new_model_name -f path/to/Modelfile
Motivation:
When there is a need to develop a customized model tailored to specific needs or datasets, you would use this command to create such a model from a predefined Modelfile
. This flexibility is invaluable for researchers, developers, or entities aiming to tailor language models for unique applications or experimenting with new model architectures.
Explanation:
create
: This action sets in motion the process of generating a new language model.new_model_name
: The intended name for the model being created, allowing for easy identification.-f
: Stands for ‘file’, indicating that what follows is the file path.path/to/Modelfile
: The specific filepath pointing to theModelfile
which contains definitions, instructions, and data necessary for creating the model.
Example Output:
Creating model: new_model_X from Modelfile
Model creation has been completed successfully.
Conclusion:
The ‘ollama’ command simplifies the process of interacting with and managing language models. Whether it’s starting a session, updating models, or customizing new ones, this suite of commands offers users robust control and flexibility for varied applications in machine learning and artificial intelligence. Familiarity with these commands empowers users to fully leverage the potential of language models, enhancing efficiency and the capabilities of their systems.