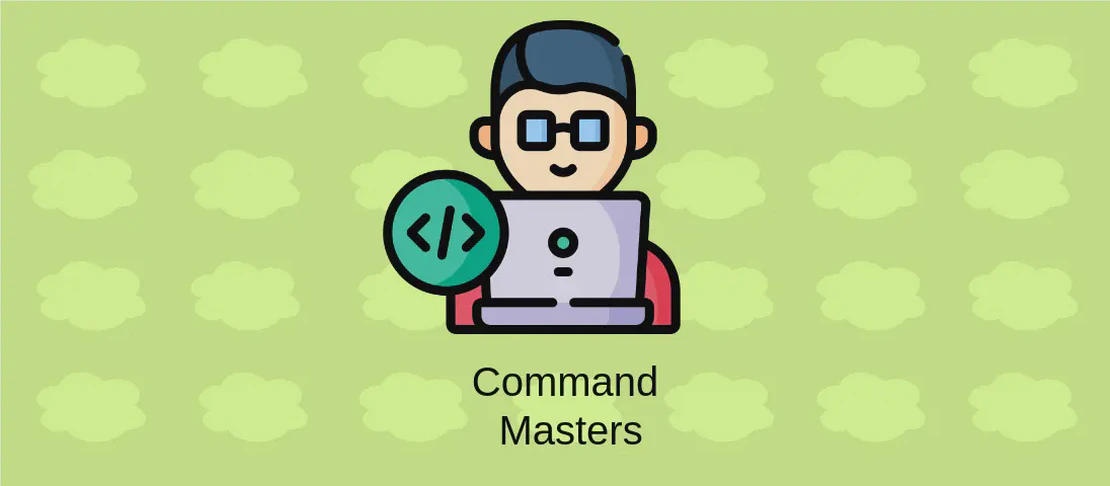
How to Optimize and Analyze LLVM Source Files with 'opt' (with examples)
The opt
command is a powerful tool used to run optimizations and analyses on LLVM Intermediate Representation (IR) bitcode files. It is part of the LLVM Compiler Infrastructure and allows developers to apply various transformations and inspect different analyses of their programs. This is especially useful for improving code performance, debugging, and understanding the internals of code execution by examining various representations and optimizations.
Use case 1: Run an Optimization or Analysis on a Bitcode File
Code:
opt -passname path/to/file.bc -S -o file_opt.bc
Motivation:
Using the opt
command to run an optimization or analysis is essential when working with compiled IR files in LLVM. By applying specific passes, developers can eliminate redundant code, optimize performance, or analyze various aspects of the program’s behavior. Each pass inspects or transforms the code to make it more efficient or provides insights into the program’s operations. This process is a crucial step in preparing high-performance applications and ensuring that the generated machine code is as optimal as possible.
Explanation:
-passname
: This argument specifies the particular optimization or analysis pass to be executed. Each pass corresponds to a specific transformation or analysis, such as dead code elimination or function inlining.path/to/file.bc
: This is the input file, containing the LLVM IR bitcode that will undergo the specified transformation or analysis.-S
: This flag tellsopt
to output the result in human-readable LLVM assembly language form, making it easier for developers to understand and verify the applied changes.-o file_opt.bc
: This argument specifies the output file where the optimized or analyzed bitcode will be saved.
Example Output:
A .bc file containing optimized or analyzed bitcode will be produced. This file can be further processed or compiled into machine code. If the -S
option is included, the output will be in an LLVM assembly form that can be opened and read with a text editor.
Use case 2: Output the Control Flow Graph of a Function to a .dot
File
Code:
opt -dot-cfg -S path/to/file.bc -disable-output
Motivation:
Generating a Control Flow Graph (CFG) is invaluable for developers seeking to visually analyze the flow of execution within a function. A CFG represents the order in which instructions are executed, which can assist in identifying bottlenecks, understanding complex code structures, and aiding with optimizations. By exporting the CFG to a .dot
file, it can then be used with graph visualization tools like Graphviz for visual inspection and analysis.
Explanation:
-dot-cfg
: This option generates a.dot
file for each function in the bitcode file, representing its Control Flow Graph.-S
: Again, this flag indicates that the output should be in human-readable form, even though.dot
generation is the primary concern here.path/to/file.bc
: The path to the input bitcode file that contains the functions for which the CFG will be generated.-disable-output
: This option prevents any output bitcode file from being generated since the primary goal is to create.dot
files for visualization, not to transform the bitcode itself.
Example Output:
For each function in the .bc
file, a corresponding .dot
file representing its CFG will be created. These files can be opened and visualized using tools like Graphviz to inspect the function’s control flow visually.
Use case 3: Optimize the Program at Level 2 and Output the Result to Another File
Code:
opt -O2 path/to/file.bc -S -o path/to/output_file.bc
Motivation:
Optimization levels in LLVM range from -O0
(no optimization) to -O3
(maximum optimization). Specifying -O2
offers a balanced approach between compilation time and optimization. This level typically includes aggressive optimizations such as code inlining, loop transformations, and dead code elimination. Using the opt
command with -O2
is often a standard procedure for improving the execution speed and reducing the size of the code without entering extremes of optimization.
Explanation:
-O2
: This is an optimization level that applies a series of complex optimizations aimed at improving runtime performance and reducing code size.path/to/file.bc
: This is the input bitcode file that will be optimized.-S
: Outputs the result in LLVM assembly language, which is human-readable.-o path/to/output_file.bc
: The name of the file where the optimized bitcode output will be stored.
Example Output:
The optimized bitcode file will contain more efficient code after the specified optimization level is applied. By examining the LLVM assembly output with -S
, developers can observe changes such as reduced redundant instructions and improved code flow leading to potentially faster execution times.
Conclusion
The opt
tool in LLVM enables developers and researchers to effectively optimize and analyze LLVM bitcode to generate efficient and insightful optimizations. Each use case demonstrates specific scenarios for applying transformations, generating graphs, or adjusting optimization levels, providing powerful capabilities for improving and understanding code performance at a low level.