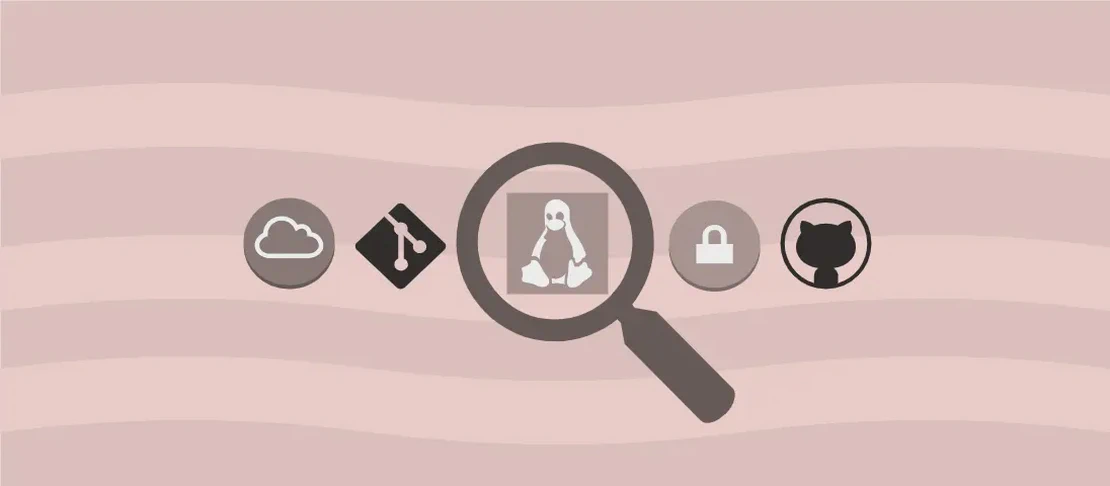
How to use the command 'pants' (with examples)
Pants is a fast, scalable, and user-friendly open-source tool designed to simplify build and developer workflows for large codebases. It offers a range of functionalities that enhance efficiency, improve code quality, and minimize manual tasks in software development. From listing targets to creating distributable packages, Pants streamlines many operations crucial to developers.
Use case 1: Listing all targets
Code:
pants list ::
Motivation:
In a large project, it’s often essential to have an overview of all the build targets, which could be libraries, binaries, or tests. Listing all targets helps developers understand the project’s structure and manage dependencies effectively.
Explanation:
pants
: Invokes the Pants command-line tool.list
: Specifies that the operation intends to list targets.::
: A wildcard symbol that matches all available targets in the project.
Example output:
path/to/first:target
path/to/second:target
path/to/third:target
This example output shows various targets within the project, offering a comprehensive view of what can be built and tested.
Use case 2: Running all tests
Code:
pants test ::
Motivation:
Running tests is crucial for ensuring that code changes do not introduce bugs. This command allows developers to execute all tests across the entire codebase, providing confidence that the software functions as expected after modifications.
Explanation:
pants
: Runs the Pants command-line tool.test
: Requests the execution of tests.::
: Ensures that all tests in the project are run.
Example output:
✓ path/to/test1.py: passed
✓ path/to/test2.py: passed
✗ path/to/test3.py: failed
The output lists test results, identifying which tests passed and which failed, thereby guiding developers toward areas that may need troubleshooting.
Use case 3: Fix, format, and lint only uncommitted files
Code:
pants --changed-since=HEAD fix fmt lint
Motivation:
Continuous code quality maintenance ensures that a project’s codebase remains clean and functional. This command focuses on uncommitted changes, helping developers keep new modifications up to standard without having to run formatting and linting on the entire codebase.
Explanation:
pants
: Initiates the Pants tool.--changed-since=HEAD
: Restricts operations to files changed since the last commit.fix
,fmt
,lint
: Sequentially fix issues, format code, and check for linting errors.
Example output:
path/to/changed_file.py: reformatted
path/to/other_changed_file.py: no issues found
The output shows the changes applied to the files, such as reformatting operations or confirmations of compliance with coding standards.
Use case 4: Typechecking only uncommitted files and their dependents
Code:
pants --changed-since=HEAD --changed-dependents=transitive check
Motivation:
Typechecking is a great practice to catch type-related errors early, which are often subtle and hard to debug. Running typechecks specifically on newly changed files and their transitive dependents ensures that recent changes do not introduce new type-related issues, thus enforcing type safety just before a commit.
Explanation:
pants
: Uses the Pants tool.--changed-since=HEAD
: Focuses typecheck on files changed since the last commit.--changed-dependents=transitive
: Expands the scope to include files that depend on the changed files, indirectly affected by changes.check
: Executes typechecking tasks.
Example output:
path/to/changed_file.py: passed
path/to/dependent_file.py: failed
This result helps developers quickly identify and resolve type errors in the affected code areas before they cause broader issues.
Use case 5: Creating a distributable package for a specified target
Code:
pants package path/to/directory:target-name
Motivation:
Building a distributable package is essential for deploying software or sharing it with others. This command simplifies the process of packaging a specified target, which could be any buildable component such as a library or application.
Explanation:
pants
: Activates the Pants tool.package
: Indicates the intent to create a package.path/to/directory:target-name
: Points to the specific directory and target in the project to package.
Example output:
Created dist/path/to/directory/target-name.tar.gz
The output confirms the successful creation of a distributable package, indicating the file location, making it ready for distribution.
Use case 6: Auto-generating BUILD file targets for new source files
Code:
pants tailor ::
Motivation:
When new source files are added to a project, updating BUILD configurations manually can be tedious and error-prone. Automatically generating BUILD file targets ensures that the new files are promptly integrated into the build system, saving time and reducing the risk of manual configuration errors.
Explanation:
pants
: Calls the Pants tool.tailor
: Auto-generates BUILD targets for the files.::
: Applies the operation to all new source files in the project.
Example output:
Created BUILD files with targets for new sources:
path/to/new_file.py:python_library
The output confirms newly created BUILD targets, specifying their types and locations, ensuring the project’s coherence.
Use case 7: Displaying help
Code:
pants help
Motivation:
Having access to a command’s documentation is crucial for users, especially when trying to learn new functionalities or recall seldom-used commands. This command provides immediate access to a brief usage overview, making it easier to understand how to best leverage the tool.
Explanation:
pants
: Engages the Pants tool.help
: Requests display of available commands and their descriptions.
Example output:
Usage: pants [options] [goals] [specs]
Options:
-h, --help Show this help message and exit
Available goals:
list List targets
test Run tests
package Create a distributable package
...
For more details, visit <https://www.pantsbuild.org/2.20/docs/using-pants/command-line-help>
The output presents a concise list of commands with descriptions, encouraging users to explore Pants’ capabilities confidently.
Conclusion:
Pants offers a suite of tools designed to simplify and enhance the development process for projects of any scale. From targeting specific elements in the codebase to ensuring type safety and code quality, Pants reduces manual overhead and promotes efficient development practices. By mastering its commands, developers can significantly streamline their workflows and maintain high code standards across their projects.