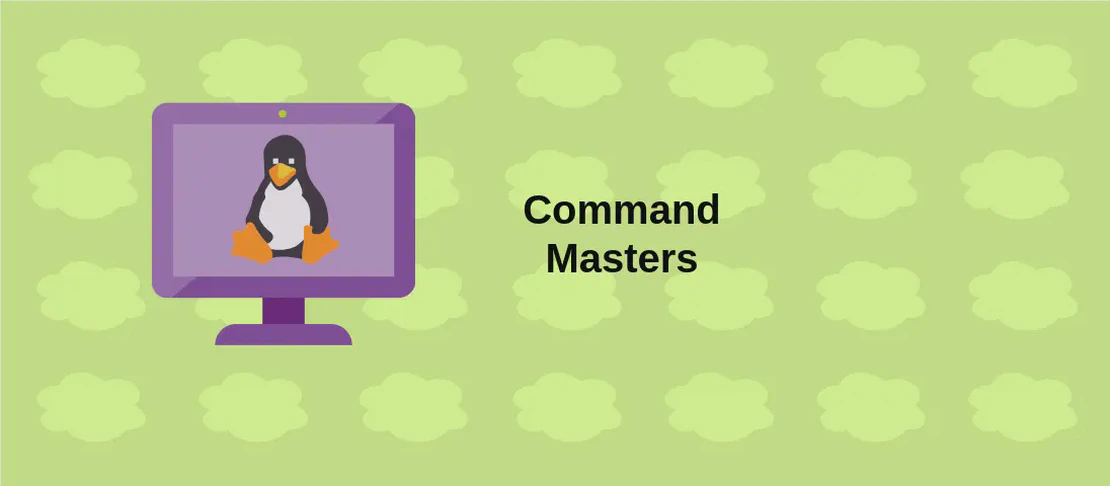
How to use the command `parallel-lint` (with examples)
parallel-lint
is a powerful command-line tool designed to help developers check the syntax of PHP files efficiently by utilizing parallel processing. Syntax errors in PHP scripts can be difficult to spot manually or during run-time, leading to bugs and unusual behavior in web applications. Parallel linting scans through code files to ensure there are no syntax issues, thus aiding in maintaining high code quality. This tool is especially useful in large projects with numerous PHP files. Let’s explore several use cases to see how parallel-lint
can be utilized.
Use case 1: Lint a specific directory
Code:
parallel-lint path/to/directory
Motivation:
For developers maintaining a PHP project or any codebase with numerous PHP scripts, ensuring that each file is free from syntax errors is critical. By using parallel-lint
, one can quickly scan an entire directory, saving time compared to checking each file individually.
Explanation:
path/to/directory
: Replace this with the actual path of the directory containing your PHP files. The command inspects all files within this directory and checks their syntax, providing a comprehensive overview of potential issues.
Example output:
PHP Parallel Lint 1.3.0
Checked path/to/directory in 10.5 seconds, found 0 errors
The result shows the time taken to lint the directory and the number of syntax errors found.
Use case 2: Lint a directory using the specified number of parallel processes
Code:
parallel-lint -j 4 path/to/directory
Motivation:
When dealing with large codebases, increasing the number of parallel processes can significantly reduce the time it takes to lint the files. This can be particularly beneficial in continuous integration or deployment pipelines where time is of the essence.
Explanation:
-j 4
: Specifies the number of parallel processes to use. Adjusting this number can optimize the processing time depending on the machine’s capability.path/to/directory
: The directory containing the PHP files you want to lint. The command will execute linting across multiple processes concurrently, as specified.
Example output:
PHP Parallel Lint 1.3.0
Using 4 parallel jobs
Checked path/to/directory in 5.2 seconds, found 0 errors
The output shows a reduction in processing time due to the increased number of parallel jobs.
Use case 3: Lint a directory, excluding the specified directory
Code:
parallel-lint --exclude path/to/excluded_directory path/to/directory
Motivation:
Sometimes, certain directories contain files that are not relevant or necessary to lint. For instance, third-party libraries or vendor directories in a project. Excluding these can improve linting speed and focus on the essential code.
Explanation:
--exclude path/to/excluded_directory
: Specifies the directory to be excluded from linting. Use this to ignore folders that do not need syntax checks.path/to/directory
: The directory where linting is to be performed, excluding the specified folder.
Example output:
PHP Parallel Lint 1.3.0
Excluded: path/to/excluded_directory
Checked path/to/directory in 8.3 seconds, found 0 errors
This indicates that the linting process ignored the specified directory successfully.
Use case 4: Lint a directory of files using a comma-separated list of extensions
Code:
parallel-lint -e php,html,phpt path/to/directory
Motivation:
Web projects often contain a mix of file types, and developers may want to run syntax checks on specific file types like PHP, HTML, and testing files. Customizing the extensions ensures only the relevant files are checked.
Explanation:
-e php,html,phpt
: This option specifies the list of file extensions to check. Separate multiple extensions with commas.path/to/directory
: Directory containing the files with the specified extensions.
Example output:
PHP Parallel Lint 1.3.0
Checked 150 files with extensions [php, html, phpt] in path/to/directory in 12.5 seconds, found 0 errors
Here, only the files with the listed extensions are linted.
Use case 5: Lint a directory and output the results as JSON
Code:
parallel-lint --json path/to/directory
Motivation:
Integrating lint results into other tools or systems often necessitates a structured output. JSON is a widely used format that can facilitate this, making the integration with applications easier for automated processes and enhancing visibility.
Explanation:
--json
: Instructsparallel-lint
to format the output in JSON, which can be parsed and utilized programmatically.path/to/directory
: The directory to lint, where the results will be captured in a JSON format.
Example output:
{
"files": 200,
"errors": 0,
"seconds": 6.2
}
The JSON output provides a clear, structured summary of the linting process.
Use case 6: Lint a directory and show Git Blame results for rows containing errors
Code:
parallel-lint --blame path/to/directory
Motivation:
Discovering errors in code is crucial, but understanding who last modified the erroneous lines can be equally important for troubleshooting. Git Blame
provides context around when the line was last changed, aiding in faster resolution.
Explanation:
--blame
: InvokesGit Blame
, attributing the lines with errors to the contributors who last modified them.path/to/directory
: The location where linting is conducted; any errors found will be annotated with Git Blame information.
Example output:
PHP Parallel Lint 1.3.0
Error found in file.php on line 34
Git Blame: John Doe, commit 45d3f21, 2022-10-01
Checked path/to/directory in 10.0 seconds, found 1 error
The output not only shows the error but identifies the contributor’s last modification, aiding collaborative debugging.
Conclusion:
Incorporating parallel-lint
into your workflow enhances the reliability and quality of PHP projects by ensuring syntax correctness efficiently. Its capability to harness parallel processing, exclude directories, tailor linting to specific file types, offer structured outputs, and integrate with Git for contextual insights makes it an indispensable tool for developers.