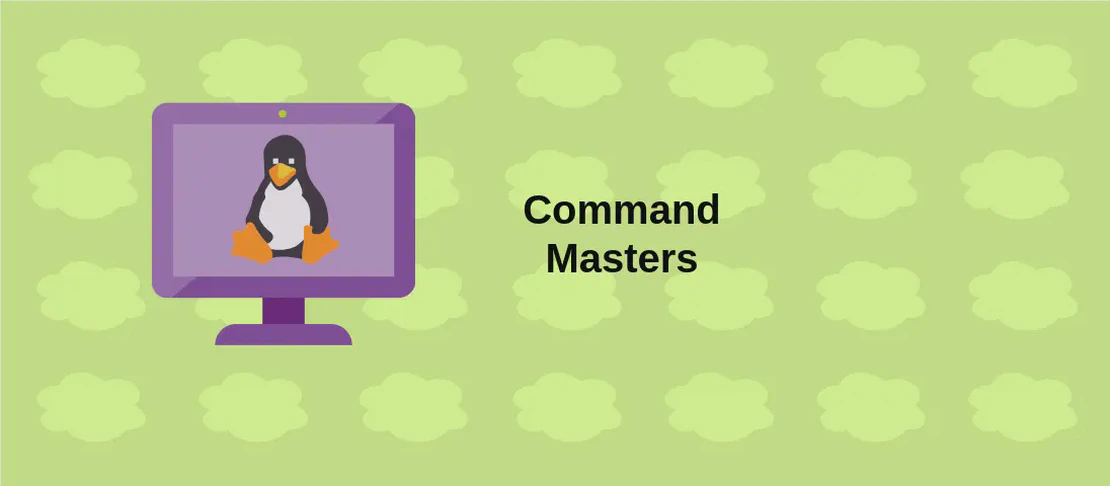
Leveraging Particle Command Line Interface (CLI) for Device Management (with examples)
The Particle Command Line Interface (CLI) is a powerful tool that allows developers to interact seamlessly with their Particle devices. With this tool, users can configure their devices, manage firmware, compile code, and execute functions directly from the command line. Its functionality is broad and tailored towards both individual developers and teams who are building connected projects with Particle’s IoT platforms.
Use case 1: Log in or Create an Account for the Particle CLI
Code:
particle setup
Motivation:
Before interacting with your Particle devices, it’s essential to establish a connection between the CLI and your Particle account. This command helps you set up your CLI by either logging into an existing Particle account or creating a new one. This step is vital for authentication and ensures that your commands are executed under the appropriate account.
Explanation:
particle
: This is the root command used to interact with the Particle CLI.setup
: This subcommand initiates the account setup process. It guides you through logging in or creating an account.
Example Output:
Welcome to the Particle Setup workflow!
> Please login or create a new account to start using the Particle CLI.
> Enter your email:
> Enter your password:
> Successfully logged in!
Use case 2: Display a List of Devices
Code:
particle list
Motivation:
Once you’re logged in, it’s often helpful to know which devices are connected to your Particle account. This command provides an overview of all devices, along with their statuses and identifying information. It’s useful for managing multiple devices and for quickly checking their connectivity status.
Explanation:
particle
: The CLI root command.list
: This subcommand fetches and displays a list of all devices associated with your Particle account, along with details such as device ID, name, and online status.
Example Output:
Device ID Name Status Type
20001a0018473434321abcd Light_Sensor online Photon
30001b0019576745234efgh Thermostat offline Argon
Use case 3: Create a New Particle Project Interactively
Code:
particle project create
Motivation:
Creating a new Particle project is a fundamental step for organizing your code and configuration for a specific application. This command helps to set up a new project directory with the necessary files and structure, providing a good starting point for any new IoT project.
Explanation:
particle
: The core command initiating the CLI interaction.project create
: This subcommand creates a new directory and initial configuration files, preparing an interactive setup environment for a new Particle project.
Example Output:
Enter the project name: MyNewProject
Project directory created at: /Users/username/MyNewProject
Initial files generated in the project folder.
Use case 4: Compile a Particle Project
Code:
particle compile photon path/to/source_code.ino
Motivation:
Compiling your source code is an essential step in ensuring that the code is error-free and ready to be uploaded to your device. The Particle CLI supports compiling code for various devices. This command is crucial for developers who modify their code frequently and require immediate feedback on the build status.
Explanation:
particle
: The main command for CLI interaction.compile
: This subcommand initiates the compilation process for your code.photon
: Specifies the type of device that you are targeting for compilation, in this case, a Photon.path/to/source_code.ino
: The path to your.ino
file, which contains the code you want to compile.
Example Output:
Compiling code for photon
Including:
path/to/source_code.ino
Home environment updated.
Project successfully compiled.
Use case 5: Update a Device to Use a Specific App Remotely
Code:
particle flash device_name path/to/program.bin
Motivation:
Flashing a new application to your device is often necessary when you update your code. This command allows you to remotely update a device with a new binary file, ensuring that your device runs the latest version of your application with minimal downtime.
Explanation:
particle
: The CLI command.flash
: This subcommand initiates the flashing process to update the firmware on a device.device_name
: The name of the target device you wish to flash.path/to/program.bin
: The path to the binary file of your compiled code.
Example Output:
Flashing code to device_name...
Flash successful! Your device_name is updating...
Use case 6: Update a Device to Use the Latest Firmware via Serial
Code:
particle flash --serial path/to/firmware.bin
Motivation:
Sometimes a direct USB connection is necessary or more reliable for updating firmware, especially in development or debugging contexts. This command allows for firmware updates via serial, which can be especially useful when working with local devices.
Explanation:
particle
: Root command for CLI operations.flash
: This triggers the firmware update process.--serial
: Specifies that the update should be done over a serial connection.path/to/firmware.bin
: The path to the binary file for the firmware you wish to install.
Example Output:
Put your device in DFU mode and click ENTER when ready.
Flashing firmware via serial...
Update successful!
Use case 7: Execute a Function on a Device
Code:
particle call device_name function_name function_arguments
Motivation:
Being able to invoke functions on your Particle device directly from the command line can drastically expedite testing and development processes. This can be especially useful for triggering actions or responses in real-time.
Explanation:
particle
: The command interface to the Particle cloud.call
: This subcommand indicates that you are calling a function on the device.device_name
: The name of the device on which the function will be executed.function_name
: The specific function you wish to execute on the device.function_arguments
: The arguments to be passed to the function. This can be empty if the function does not require arguments.
Example Output:
Calling function switchLight on device Light_Sensor
Successfully called function: response: {"return_value":1}
Conclusion:
The Particle CLI is an extensive toolkit for developers working within the Particle ecosystem. By offering commands for setup, device management, code compilation, firmware updates, and function execution, it streamlines the process of developing for and maintaining Particle IoT devices. Each use case exemplifies how specific commands provide critical functionality and ease the intricacies of working with connected devices. Whether you are experimenting with a single project or managing a fleet of devices, understanding and utilizing these commands can significantly enhance your development workflow.