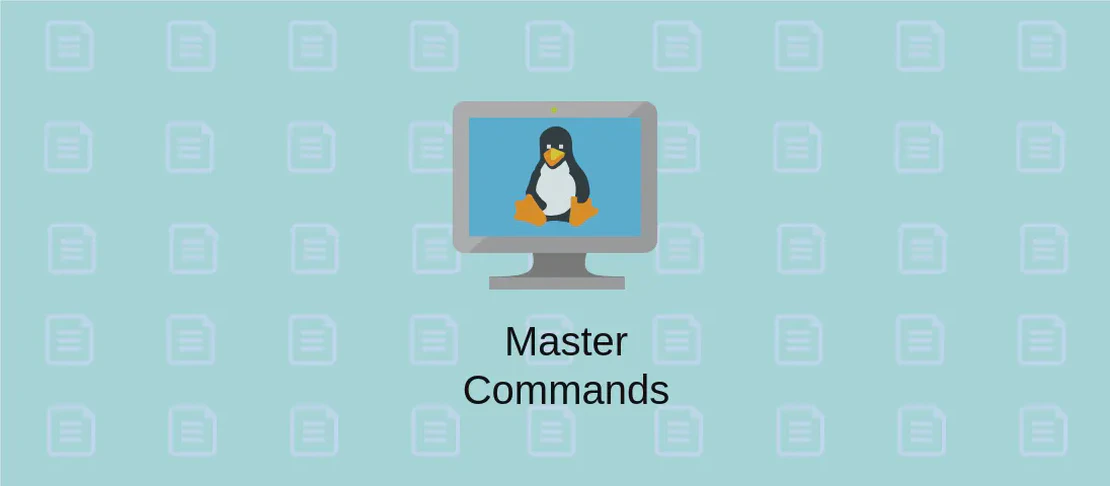
Mastering Perl Command Line Options (with examples)
The Perl programming language is renowned for its flexibility and power, especially in text processing tasks. The Perl interpreter allows users to execute Perl scripts directly from the command line, providing a wide range of command-line options and arguments that can significantly enhance productivity for developers. It is commonly used for various tasks such as pattern matching, one-liners, file processing, and more. Below are some practical use cases to demonstrate how Perl can be leveraged efficiently from the command line.
Use case 1: Print lines from stdin
matching regex1 and case insensitive regex2
Code:
perl -n -e 'print if m/regex1/ and m/regex2/i'
Motivation:
This example is particularly useful when you want to filter lines of input based on two conditions: one that matches a regular expression exactly (regex1
) and another that matches a regular expression case-insensitively (regex2
). This dual filtering can be handy in log processing or when searching through large data sets for information that meets multiple criteria.
Explanation:
perl
: Invokes the Perl interpreter.-n
: Puts a loop around your script, which processes each line of input fromstdin
, one by one.-e
: Allows you to provide a script to execute right from the command line.print if m/regex1/ and m/regex2/i
: Within the loop,print
the line if it matchesregex1
andregex2
(case-insensitive). Them//
syntax is used for pattern matching, and the/i
modifier ensures case insensitivity forregex2
.
Example output:
When executing the above with a stream of text through stdin
, only the lines matching both criteria will be displayed.
Use case 2: Say first match group, using a regexp, ignoring space in regex
Code:
perl -n -E 'say $1 if m/before ( group_regex ) after/x'
Motivation:
This command can be very useful when you’re interested in extracting parts of a string that match a specific pattern, while the patterns have variations in spacing. For instance, if you’re parsing structured text and need to pull out specific data embedded between consistent markers, this command will efficiently extract and print only those matched groups.
Explanation:
perl
: Calls the Perl interpreter.-n
: As before, this option wraps your script in a loop that processes each input line.-E
: Like-e
, but automatically enables features of the current Perl version, including printing.say $1
:say
is a feature similar toprint
but adds a newline at the end.$1
is used to refer to the first captured match group.if m/before ( group_regex ) after/x
: The/x
modifier makes the pattern ignore whitespace, allowing the regular expression to be formatted for readability.
Example output:
Given input with segments surrounded by known markers, this command will output only the portion that falls within those markers.
Use case 3: In-place, with backup, substitute all occurrences of regex with replacement
Code:
perl -i'.bak' -p -e 's/regex/replacement/g' path/to/files
Motivation:
Performing in-place edits on multiple files is a frequent requirement, especially when batch updating a large codebase or data set. Creating backups of the original files is crucial for safety, allowing for rollback if the changes aren’t as expected.
Explanation:
perl
: Initiates the Perl interpreter.-i'.bak'
: Enables in-place editing of files and creates a backup with the extension.bak
before making any changes.-p
: Automatically loops over every line of input files, printing every line after processing.-e
: Provides the script directly in the command line.s/regex/replacement/g
: Performs a global substitution ofregex
withreplacement
throughout each line.
Example output:
The specified files at path/to/files
will be updated, and each original file will have a backup with .bak
appended to its name.
Use case 4: Use Perl’s inline documentation
Code:
perldoc perlrun ; perldoc module ; perldoc -f splice; perldoc -q perlfaq1
Motivation:
Understanding and utilizing Perl’s extensive documentation can significantly enhance your effectiveness as a developer. The perldoc
utility provides easy access to various Perl manual pages and specific function or syntax documentation, ensuring that you have the necessary knowledge and tools at your disposal.
Explanation:
perldoc perlrun
: Displays the manual page for Perl’s command-line options and script execution.perldoc module
: Opens the documentation for any installed module, wheremodule
is the specific module’s name.perldoc -f splice
: Gives information about the Perl functionsplice
.perldoc -q perlfaq1
: Provides answers to frequently asked questions, withperlfaq1
focusing on one specific section.
Example output:
The command output will include detailed documentation and explanations for the specified topics, helping users understand their utility and potential applications.
Conclusion:
Mastering these Perl command-line options can dramatically improve the efficiency of text processing and file manipulation tasks. Whether you’re extracting information with regular expressions, editing files in-place, or referencing Perl’s inline documentation, these techniques are indispensable tools in a Perl programmer’s toolkit.