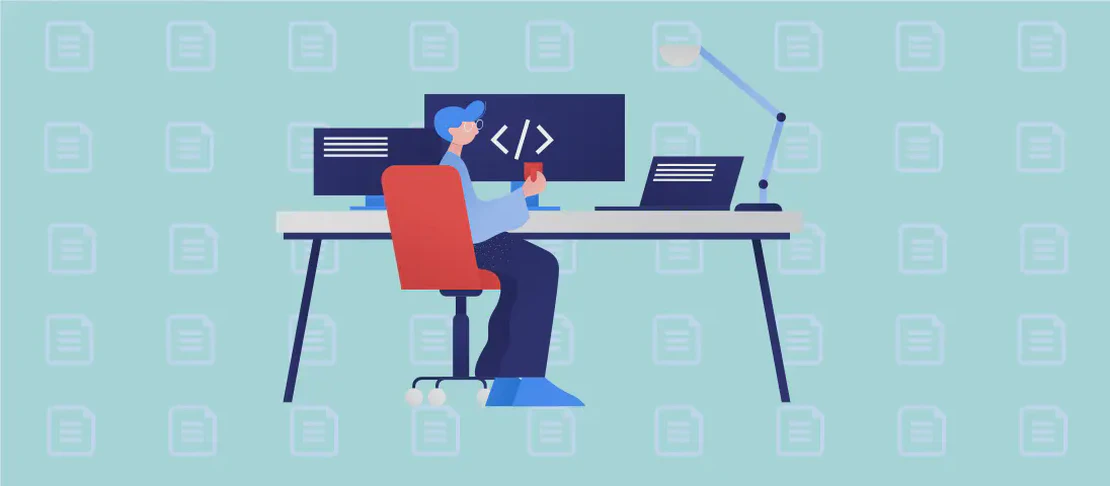
A Comprehensive Guide to Using the 'rename' Command (with Examples)
The ‘rename’ command, part of the Perl package on Arch Linux, is a powerful tool for batch renaming files through regular expressions. This utility is incredibly useful in scenarios where a large number of files need to be renamed based on specific patterns or conversions. It provides flexibility and precision in renaming tasks, making it an indispensable tool for users looking to automate the renaming process.
Use case 1: Renaming Files Using a Perl Common Regular Expression
Code:
rename 's/foo/bar/' *
Motivation: Suppose you are managing a large collection of files that need to be renamed consistently. For example, if you have several files with the word “foo” in their names and you want to replace it with “bar” for branding purposes or organizational needs, manually renaming each file can be tedious and time-consuming. The rename
command automates this task efficiently.
Explanation: In this command, 's/foo/bar/'
is a Perl regular expression where s
stands for substitute. It replaces occurrences of ‘foo’ with ‘bar’ in each filename. The asterisk (*
) acts as a wildcard, telling the command to apply this operation to all files in the current directory.
Example Output: Before rename - foo.txt
, foo_report.pdf
; After rename - bar.txt
, bar_report.pdf
.
Use case 2: Performing a Dry Run to Display Potential Renames
Code:
rename -n 's/foo/bar/' *
Motivation: Sometimes, you might want to preview the changes that will occur without actually making them. This is essential to ensure you have the correct regular expression pattern and target files before executing the rename operation.
Explanation: The -n
flag stands for a dry run. It lists the file renames that would take place if the command were executed without the -n
option. This is a safe way to double-check your command’s impact.
Example Output: (Dry run with no actual changes) - Would rename foo.txt
to bar.txt
, and foo_report.pdf
to bar_report.pdf
.
Use case 3: Forcing Rename Operation to Overwrite Existing Files
Code:
rename -f 's/foo/bar/' *
Motivation: During file renaming, there may be conflicts where the destination filename already exists. In cases where overwriting the existing file is necessary, the force option prevents the operation from aborting due to these conflicts.
Explanation: The -f
flag ensures that if a file with the target name already exists, it will be overwritten by the renamed file. This is particularly useful in environments where filename conflicts are common and need to be resolved automatically.
Example Output: If bar.txt
already exists, foo.txt
will overwrite bar.txt
without errors.
Use case 4: Converting Filenames to Lower Case
Code:
rename 'y/A-Z/a-z/' *
Motivation: File naming conventions often require consistency in case. For instance, a set of files in various cases can lead to confusion or compatibility issues, especially across different operating systems. Converting all filenames to lowercase can help maintain uniformity.
Explanation: The expression 'y/A-Z/a-z/'
in the command is a transliteration replacing all uppercase letters with their lowercase counterparts in each of the filenames. The wildcard *
applies this conversion to all files in the current directory.
Example Output: Before rename - FileOne.txt
, AnotherFile.JPG
; After rename - fileone.txt
, anotherfile.jpg
.
Use case 5: Replacing Whitespace with Underscores
Code:
rename 's/\s+/_/g' *
Motivation: Many filesystems and applications handle filenames with spaces inconsistently, which can lead to issues during file transfers or scripting tasks. Replacing spaces with underscores ensures safer file storage and manipulation.
Explanation: In this command, 's/\s+/_/g'
is a regular expression where \s+
matches one or more whitespace characters, and _
replaces them with underscores. The g
stands for global, meaning the replacement will occur for all such matches in each filename.
Example Output: Before rename - My File.txt
, Another Document.pdf
; After rename - My_File.txt
, Another_Document.pdf
.
Conclusion:
The ‘rename’ command offers a variety of options to efficiently handle complex file renaming tasks. By understanding and utilizing the power of regular expressions along with several flags, users can automate renaming processes, enhancing productivity and ensuring consistency across large batches of files. Whether it’s adjusting capitalization, replacing patterns, or ensuring files follow a specific naming convention, ‘rename’ proves to be an indispensable tool.