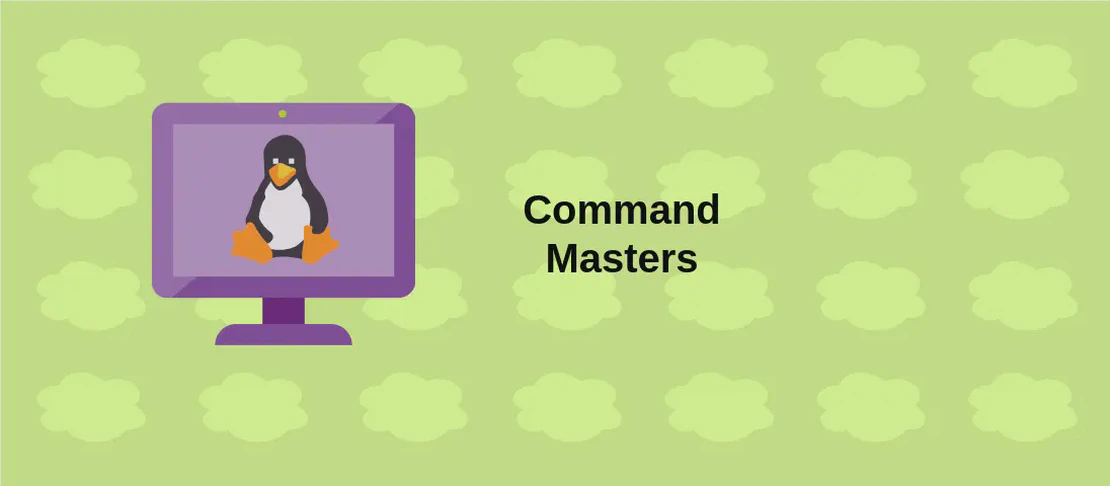
How to Use the Command 'pest' (with examples)
Pest is a PHP testing framework designed for simplicity and elegance. It provides a streamlined and intuitive interface for writing tests, making it an attractive alternative to other PHP testing frameworks like PHPUnit. With Pest, developers can write tests quickly and clearly, improving code quality and reliability in PHP projects.
Use case 1: Initialize a standard Pest configuration in the current directory
Code:
pest --init
Motivation:
Initializing Pest in your project is the first step towards setting up a comprehensive testing environment. By running this command, you bootstrap your project with the necessary configuration files that are essential for executing tests smoothly. This helps establish a solid foundation for writing and organizing your test cases as your project grows.
Explanation:
pest
: The command initiates the Pest framework.--init
: This argument creates the default configuration files needed for Pest. It sets up your directory with essential files likepest.php
, allowing you to quickly start adding and running tests with minimal setup overhead.
Example Output:
Pest configuration initialized in your project. Ready to write your first test!
Use case 2: Run tests in the current directory
Code:
pest
Motivation:
Running your tests is a crucial part of the development process. As you make changes to your code, you will want to frequently execute your test suite to ensure that everything is working as expected. Using Pest for this task helps ensure that any regressions or bugs are caught early in the development cycle.
Explanation:
pest
: Invoking Pest without additional arguments will run all the tests in the current directory. This command gathers and executes all tests it finds, providing feedback on their success or failure.
Example Output:
Running tests...
. OK
All tests passed (1 test)
Use case 3: Run tests annotated with the given group
Code:
pest --group name
Motivation:
When working on a large project with numerous tests, it can be beneficial to focus on a specific subset. Grouping tests lets developers categorize them by functionality, feature, or module, thus allowing targeted test execution. This results in faster, more focused feedback, especially during rapid development cycles.
Explanation:
pest
: Executes the test runner.--group name
: Thegroup
option specifies a set of tests marked with a particular annotation. Thename
refers to the specific group of tests you aim to execute, and only tests within this group will be run.
Example Output:
Running tests in group "name"...
. OK
All tests in group "name" passed
Use case 4: Run tests and print the coverage report to stdout
Code:
pest --coverage
Motivation:
Understanding which parts of your code are covered by tests can be crucial in determining areas that might need more testing. Code coverage reports provide visibility into the robustness of your test suite, enabling you to improve test coverage and, subsequently, code reliability.
Explanation:
pest
: The test runner command.--coverage
: This option generates a code coverage report and prints it tostdout
. It allows developers to review the sections of code that tests cover, helping improve test coverage.
Example Output:
Running tests...
. OK
Coverage report:
Lines: 85.71% (6/7)
Methods: 100.00% (2/2)
Classes: 100.00% (1/1)
Use case 5: Run tests with coverage and fail if the coverage is less than the minimum percentage
Code:
pest --coverage --min=80
Motivation:
Maintaining a standardized level of code coverage ensures that a certain portion of your codebase is tested at all times. By setting a minimum coverage percentage, teams can enforce quality gates, ensuring that new code is adequately tested before integration.
Explanation:
pest
: Runs the tests.--coverage
: Generates a coverage report.--min=80
: This argument sets a minimum threshold for test coverage. If the current coverage percentage falls below 80%, the test suite will fail, indicating further test writing is needed.
Example Output:
Running tests...
. OK
Coverage report:
Lines: 75.00% (6/8)
Methods: 100.00% (2/2)
Classes: 100.00% (1/1)
Coverage check failed! Minimum required is 80%
Use case 6: Run tests in parallel
Code:
pest --parallel
Motivation:
Modern software projects can have extensive test suites that take a significant amount of time to run. Running tests in parallel can drastically reduce the time spent on testing by leveraging multiple CPU cores, thereby increasing productivity and accelerating the development process.
Explanation:
pest
: Executes the test suite.--parallel
: This option runs tests simultaneously across different processors or cores, which can lead to a substantial reduction in test execution time as opposed to sequentially running tests.
Example Output:
Running tests in parallel...
. . OK (Executed in parallel)
All tests passed
Use case 7: Run tests with mutations
Code:
pest --mutate
Motivation:
Mutation testing is a method used to determine the effectiveness of your test suites. It involves making small changes (mutations) to your code and checking if the test suite can detect the issues these changes might introduce. It helps in enhancing test cases to catch more potential faults.
Explanation:
pest
: Initiates the Pest test runner.--mutate
: This experimental feature runs mutation tests to evaluate the strength and thoroughness of existing tests by introducing minor code modifications and observing if tests fail as expected.
Example Output:
Running mutation tests...
Mutation results:
- Mutations: 5
- Killed Mutants: 4
- Alive Mutants: 1
The test suite needs improvement to catch all mutations.
Conclusion:
Pest PHP is an increasingly popular testing framework praised for its simplicity and efficiency. Each command not only provides developers the ability to execute tests quickly but also offers tools for improving code coverage and test suite effectiveness through parallel execution and mutation testing. By incorporating these commands into your development workflow, you can significantly enhance the quality and reliability of your PHP applications.