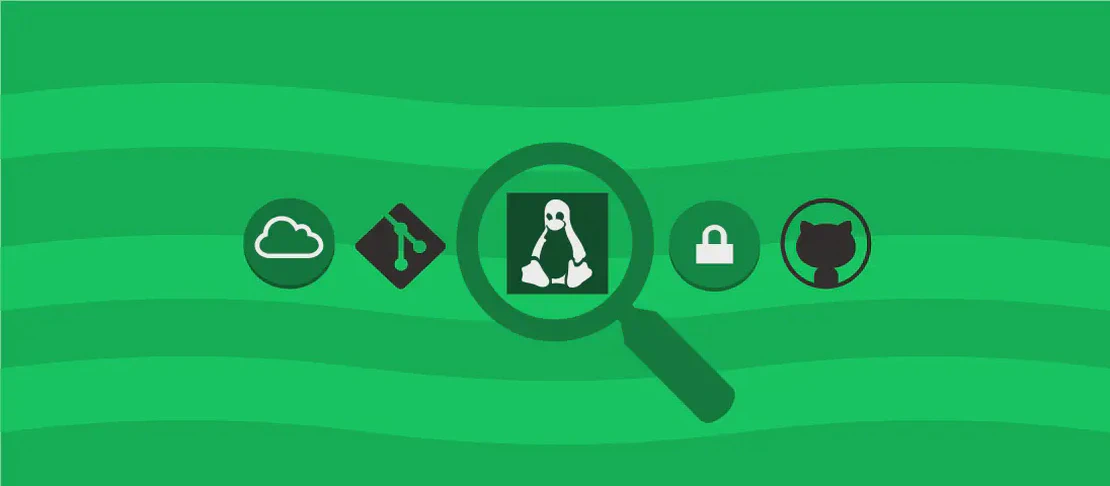
Phing: Mastering PHP Build Automation (with examples)
Phing is a robust build tool tailored for PHP projects, drawing its inspiration from Apache Ant. Essentially, it automates common tasks in software development such as file operations, running tests, and deploying applications. By using predefined tasks and offering the flexibility to create custom tasks, Phing streamlines the workflow and enhances productivity.
Use case 1: Perform the default task in the build.xml file
Code:
phing
Motivation:
Running the default task defined in the build.xml
is ideal when you want to quickly execute the primary sequence of build steps with a single command. This is especially useful for developers maintaining consistent build environments, ensuring that all necessary operations like cleaning, compiling, testing, and packaging are handled automatically.
Explanation:
phing
: This command runs Phing, which looks for thebuild.xml
file in the current directory and executes the default task specified within it.
Example Output:
Build sequence initiated...
Task: clean started
Files cleaned successfully.
Task: compile started
Compilation completed.
Task: package started
JAR file created.
Build completed successfully.
Use case 2: Initialize a new build file
Code:
phing -i path/to/build.xml
Motivation:
Creating a new build file is essential for establishing a structured framework for project tasks. The initialization command facilitates the setup of a new build script, which serves as the backbone of your automation processes, detailing what needs to be done and in what sequence.
Explanation:
-i
: This flag indicates the initialization of a new build file.path/to/build.xml
: Specifies the path where the newbuild.xml
file should be created.
Example Output:
New build file created at path/to/build.xml.
Template build tasks added. Customize them to suit your project needs.
Use case 3: Perform a specific task
Code:
phing task_name
Motivation:
Executing a specific task allows you to focus on particular operations within the build process. This is beneficial when working on fixes or enhancements, letting developers run only the tasks relevant to their current work without delving into the entire build sequence.
Explanation:
task_name
: Replace this with the actual name of the task you wish to execute from thebuild.xml
. It directs Phing to skip the default task and run only the named task.
Example Output:
Executing task: test
Unit tests initiated...
All tests passed successfully.
Use case 4: Use the given build file path
Code:
phing -f path/to/build.xml task_name
Motivation:
Specifying a custom build file path is crucial when managing multiple projects or configurations. It allows you to explicitly run tasks from a different build.xml
, granting the flexibility to handle diverse project setups or environments effectively.
Explanation:
-f
: This option specifies the build file to use.path/to/build.xml
: The location of the desired build file.task_name
: The specific task to run from the specified build file.
Example Output:
Using build file at path/to/build.xml.
Task: deploy started.
Deployment successful to development environment.
Use case 5: Log to the given file
Code:
phing -logfile path/to/log_file task_name
Motivation:
Logging build processes is fundamental for auditing, debugging, and retrospective analysis. By directing logs to a file, developers can keep detailed records of task executions, outcomes, and any anomalies encountered during the build.
Explanation:
-logfile
: This flag indicates that Phing should log the output to a specified file.path/to/log_file
: The file path where logs should be written.task_name
: The specific task whose output should be logged.
Example Output:
Task: backup initiated.
Back up completed.
Log written to path/to/log_file.
Use case 6: Use custom properties in the build
Code:
phing -Dproperty=value task_name
Motivation:
Custom properties allow for dynamic and flexible builds. By setting properties, developers can alter the behavior of tasks within a build script without modifying the build.xml
. This improves adaptability and efficiency, especially in multi-environment scenarios.
Explanation:
-Dproperty=value
: Defines a property and assigns it a value, making it accessible within the build script.task_name
: The task to execute with the specified property settings.
Example Output:
Executing task: release
Property version set to 2.0.0
Release build created for version 2.0.0.
Use case 7: Specify a custom listener class
Code:
phing -listener class_name task_name
Motivation:
Using a custom listener class is advantageous for developers requiring bespoke logging, event handling, or other custom notifications during the build process. This provides a means to extend Phing’s functionality and integrate it with other systems or workflows.
Explanation:
-listener
: Option to specify a custom listener class.class_name
: The name of the listener class to use during the task.task_name
: The specific task to execute with the listener.
Example Output:
Build listener: CustomBuildListener activated
Task: analyze started.
Code analysis completed.
Notification sent using CustomBuildListener.
Use case 8: Build using verbose output
Code:
phing -verbose task_name
Motivation:
Verbose output is invaluable for debugging and understanding detailed task executions. It provides comprehensive insights into the build processes, aiding developers in troubleshooting issues and optimizing the build tasks.
Explanation:
-verbose
: Flag to enable detailed output for every step in the build process.task_name
: The specific task to run with verbose logging.
Example Output:
Initializing build: detailed output enabled.
Step 1: Cleaning Initiated - Removing temporary files
Step 2: Compilation - Compiling source files
Step 3: Testing - Running PHP unit tests
All steps executed with detailed logging.
Conclusion:
Phing, with its versatile command options, empowers developers and teams to automate, streamline, and control the complex processes involved in building PHP projects. Whether initializing new build files, executing specific tasks, or applying custom configurations, Phing serves as a reliable tool in the arsenal of PHP developers.