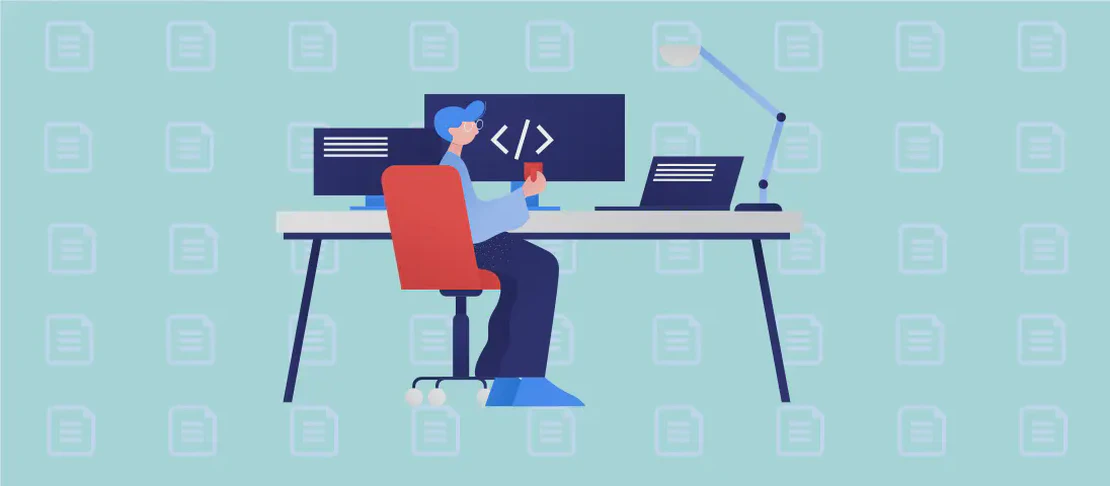
How to Use the PHP Artisan Command (with Examples)
The PHP Artisan Command-Line Interface (CLI) is a powerful tool bundled with the Laravel framework. It helps developers manage and streamline a variety of tasks related to Laravel. Artisan offers a range of commands that assist with application development, from generating boilerplate code to optimizing performance. This article explores some of the common use cases of the php artisan
command and demonstrates how to leverage its capabilities effectively.
Use Case 1: Starting the Built-in Web Server
Code:
php artisan serve
Motivation:
Starting a web server is one of the first things you’ll want to do while developing a Laravel application. The Artisan serve
command spins up PHP’s built-in web server, allowing you to develop your application without setting up a more complex server infrastructure, like Apache or Nginx. This is particularly useful in the development phase of a project for testing and rapid iteration.
Explanation:
php
: Invokes the PHP CLI, the necessary tool for executing PHP scripts.artisan
: Refers to Laravel’s command-line utility.serve
: Specifies the command to start the built-in development server. By default, it runs onhttp://localhost:8000
.
Example Output:
When you run php artisan serve
, you’ll see something like:
Starting Laravel development server: http://127.0.0.1:8000
[Tue Oct 10 12:34:56 2023] PHP 8.1.12 Development Server (http://127.0.0.1:8000) started
Listening on http://127.0.0.1:8000
Press Ctrl-C to quit.
Use Case 2: Starting an Interactive Shell with Tinker
Code:
php artisan tinker
Motivation:
Tinker
is a robust REPL (Read-Eval-Print Loop) for Laravel, allowing developers to interact with their application code in real-time. It is immensely valuable for testing simple queries, exploring API responses, and debugging without altering your main application code. Using tinker
, you can quickly experiment with your models, run queries, and much more directly from the command line.
Explanation:
php
: Initiates the PHP scripting interface.artisan
: References the command-line tool provided by Laravel.tinker
: Activates the interactive shell, integrating the powerful PsySH to provide a deeply interactive experience for handling PHP code.
Example Output:
Once you enter php artisan tinker
, the output might look like this:
Psy Shell v0.10.8 (PHP 8.1.12 — cli) by Justin Hileman
>>>
You’ll see the >>>
prompt, indicating that you can now interactively execute PHP code.
Use Case 3: Generating an Eloquent Model with Additional Components
Code:
php artisan make:model ModelName --all
Motivation:
For rapid application development, generating a model with all its essential components ensures consistency and accelerates initial setup. This command is effective in setting up new modules, leading to organized and testable code by automatically creating elements like migrations and controllers. This automation alleviates the need for repetitive manual code writing.
Explanation:
php
: Calls upon the PHP command-line interface.artisan
: Takes you into Laravel’s command tool.make:model
: Command to create a new Eloquent model class in Laravel.ModelName
: Placeholder for the desired name of your model. It should be replaced by the actual model name you wish to create.--all
: A flag that directs Artisan to not only create the model but also generate a migration file, factory, and resource controller, covering multiple facets of model handling comprehensively in one go.
Example Output:
Executing this command for a model named “Post”:
Model created successfully.
Factory created successfully.
Created Migration: 2023_10_10_123456_create_posts_table
Controller created successfully.
This output confirms that all relevant files have been generated for the “Post” model.
Use Case 4: Displaying Available Artisan Commands
Code:
php artisan help
Motivation:
Understanding the breadth of tasks Artisan can help with is crucial for any Laravel developer. The help
command provides an overview of all the available commands, facilitating their discovery and utilization. Using php artisan help
acts as an entry point for developers to locate specific commands or uncover unknown ones.
Explanation:
php
: Engages the PHP interpreter.artisan
: Specifies using Laravel’s artisan tools.help
: Lists all commands available within artisan, offering descriptions and categorizations that can guide users to the relevant actions they seek to perform.
Example Output:
When you run php artisan help
, you will receive a comprehensive list of commands, similar to the snippet below:
Usage:
command [options] [arguments]
Options:
-h, --help Display help for the given command
-q, --quiet Do not output any message
Available commands:
clear-compiled Remove the compiled class file
make:controller Create a new controller class
...
Conclusion:
The php artisan
command-line interface is a cornerstone of Laravel development, streamlining numerous tasks from setting up the development environment to automating code generation. By familiarizing yourself with its capabilities, you can significantly enhance your efficiency and effectiveness as a Laravel developer, focusing more on crafting innovative solutions rather than redundant setup tasks. Whether you’re serving a local development server with serve
, exploring code with tinker
, or leveraging the make
suite for scaffolding, Artisan provides the tools you need to succeed.