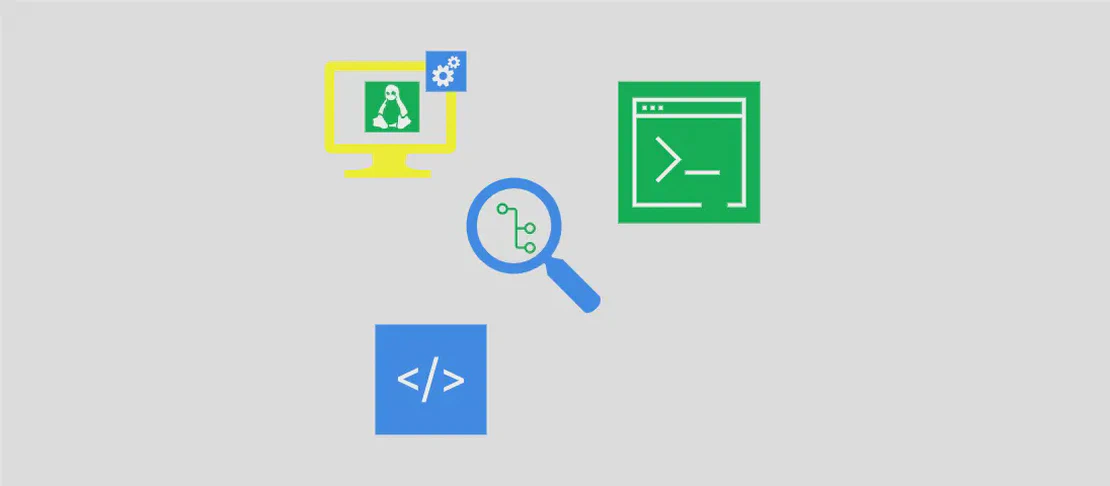
How to use the command 'php' (with examples)
PHP, which stands for Hypertext Preprocessor, is a widely-used open-source scripting language designed specifically for web development but also used as a general-purpose programming language. This article will explore various use cases of the PHP command-line interface (CLI), which allows you to execute PHP scripts directly from the command line, making it an essential tool for developers who work with PHP.
Use case 1: Parsing and Executing a PHP Script
Code:
php path/to/file
Motivation: When developing a PHP application, you may want to test your scripts without deploying them on a web server. Running scripts directly from the command line allows you to quickly check functionality and perform tasks such as database migrations or seeding.
Explanation: The command consists of ‘php’ which initiates the PHP CLI and ‘path/to/file’ which specifies the path to the PHP file to be executed.
Example Output: If the PHP script contained echo statements, you would see their output directly in the terminal. For instance, if the file outputs “Hello, World!”, you would see this in the terminal:
Hello, World!
Use case 2: Checking Syntax on a PHP Script
Code:
php -l path/to/file
Motivation: Before running a PHP script, it’s essential to check for syntax errors to avoid runtime errors that could halt execution. The lint option helps verify that your code is free of syntax errors.
Explanation: Here, ‘-l’ stands for ’lint’, a process of checking the source code for syntax warnings and errors. ‘path/to/file’ specifies which file to lint.
Example Output: If your script is free of syntax errors, the output will be:
No syntax errors detected in path/to/file
If there are syntax errors, you will receive detailed error messages showing the line numbers and nature of the errors.
Use case 3: Running PHP Interactively
Code:
php -a
Motivation: The interactive mode is useful for experimenting with PHP code snippets without having to create and save a file. It provides an environment similar to that offered by interpreters in other programming languages such as Python.
Explanation: The ‘-a’ flag stands for ‘interactive’ mode. This tells the PHP CLI to enter an interactive shell where you can type and execute PHP code line by line.
Example Output: Entering interactive mode will show a prompt where you can type PHP code:
Interactive shell
php >
Use case 4: Running Inline PHP Code
Code:
php -r "code"
Motivation: Sometimes you need to execute small PHP code snippets quickly without creating a new file. The ‘-r’ option allows you to execute code directly from the command line.
Explanation: The ‘-r’ option stands for ‘run’. It tells the PHP interpreter to run the code enclosed in the double quotes. Note that you don’t need to use PHP opening tags (<?php ?>
) in this inline execution.
Example Output: If the code snippet is php -r "echo 'Hello, CLI!';"
, the output will be:
Hello, CLI!
Use case 5: Starting a PHP-built-in Web Server
Code:
php -S host:port
Motivation: During the development of web applications, it’s practical to test your PHP application using a lightweight server. PHP’s built-in server is perfect for this purpose because it is easy to set up and does not require installing additional software.
Explanation: The ‘-S’ option tells PHP to start a server. ‘host:port’ specifies the address and port number the server will listen on. For example, ’localhost:8000’ starts the server on your local machine at port 8000.
Example Output: Once the server is initiated successfully, the terminal will show:
PHP 7.X.X Development Server started at [date] on http://localhost:8000
You can then navigate to ‘http://localhost:8000’ in your web browser to view your application.
Use case 6: Listing Installed PHP Extensions
Code:
php -m
Motivation: Knowing which extensions are installed is crucial for PHP development, as different projects may have different requirements. You need to ensure that all necessary extensions are enabled before deploying your application.
Explanation: The ‘-m’ option instructs PHP to list all the installed PHP extensions.
Example Output: The output lists all the available PHP modules:
[PHP Modules]
bcmath
calendar
ctype
...
[Zend Modules]
Use case 7: Displaying Information About the Current PHP Configuration
Code:
php -i
Motivation: When troubleshooting, it’s often necessary to know more about how PHP is configured on your system. The ‘-i’ command provides a comprehensive view of your PHP configuration.
Explanation: The ‘-i’ option outputs extensive information about the PHP configuration and environment, similar to calling phpinfo()
in a PHP script.
Example Output: The command will output detailed configuration, including loaded configuration files, PHP settings, and loaded modules:
phpinfo()
PHP Version => 7.X.X
System => [system details]
...
Configuration File (php.ini) Path => [path]
Loaded Configuration File => [path]
Use case 8: Displaying Information About a Specific PHP Function
Code:
php --rf function_name
Motivation: Understanding the particular function’s purposes, parameters, and behavior can be essential when writing or debugging code. Knowing how functions work helps avoid misuse and errors.
Explanation: The ‘–rf’ stands for “Reflection function” which retrieves the documentation and details about the specific function ‘function_name’. This is especially useful for understanding built-in functions without referencing external documentation.
Example Output: When requesting information about the ‘strpos’ function, it will display details similar to this:
Function [ <internal:standard> function strpos ] {
...
- Parameters: $haystack, $needle [, $offset = 0 ]
...
}
Conclusion:
The PHP command-line interface offers an array of functionalities tailored for developing and troubleshooting PHP applications efficiently. From executing scripts and testing small snippets to exploring installed modules and inspecting configurations, the PHP CLI is a powerful tool that extends PHP capabilities beyond simple web server execution.