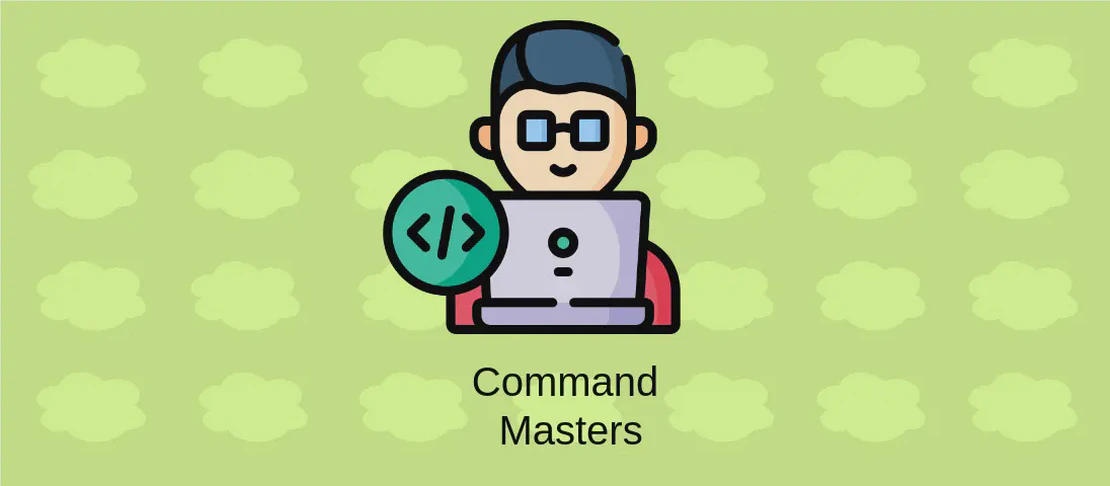
Utilizing 'phpcbf' for Code Quality Enhancement (with examples)
PHP Code Beautifier and Fixer (phpcbf
) is a powerful command-line tool for automatically fixing coding standard issues in PHP code, which are identified by PHP CodeSniffer (phpcs
). It ensures consistent style and formatting in PHP codebases, greatly reducing the time developers need to spend on code review and maintenance tasks. The tool supports various coding standards such as PEAR, PSR2, and more, facilitating a consistent coding environment across different projects and teams.
Use case 1: Fix issues in the specified directory (defaults to the PEAR standard)
Code:
phpcbf path/to/directory
Motivation: When working with a PHP codebase, consistent styling is essential for maintaining readability and collaboration. By running phpcbf
on a directory, developers can quickly fix any code that doesn’t adhere to the default PEAR coding standards without manually going through each file, thus simplifying the process of adhering to a common standard.
Explanation:
phpcbf
: The command to run the PHP Code Beautifier and Fixer.path/to/directory
: This represents the directory that contains the PHP files you want to clean up. It is important to specify the path correctly to ensure all intended files are processed.
Example Output: The command may output a list of file paths that have been processed and fixed. An example output might look like this:
Changing directory into /path/to/directory
Fixed 50 violations in 5 files
Time: 2.34 secs; Memory: 12MB
Use case 2: Display a list of installed coding standards
Code:
phpcbf -i
Motivation: Before fixing any code, it’s important to know which coding standards are available on your system. This command helps developers identify which standards they can use, facilitating better planning and management of coding style adherence.
Explanation:
phpcbf
: The command for the PHP Code Beautifier and Fixer.-i
: This option instructsphpcbf
to display a list of all installed coding standards rather than performing any fixes.
Example Output: You may see an output listing the available standards. For example:
The installed coding standards are MyStandard, PSR12, PEAR, Zend, Squiz, PSR1, and PSR2
Use case 3: Specify a coding standard to validate against
Code:
phpcbf path/to/directory --standard standard
Motivation: Different projects may require adherence to different coding standards. By specifying the standard, developers ensure compliance with the project’s particular guidelines, which may differ from the default PEAR standard.
Explanation:
phpcbf
: This is the command to initiate the fixing process withphpcbf
.path/to/directory
: Indicates the directory to be processed.--standard standard
: Specifies the desired coding standard to enforce, such as PSR2, Zend, or a custom standard.
Example Output: Output shows changes made according to the specified standard:
Changing directory into /path/to/directory
Fixed 30 violations in 3 files based on the PSR12 standard
Time: 1.85 secs; Memory: 11MB
Use case 4: Specify comma-separated file extensions to include when sniffing
Code:
phpcbf path/to/directory --extensions php,inc
Motivation: Some projects might include PHP scripts with various file extensions, not just .php
. Specifying file extensions ensures that all relevant files are included in the review process, providing a comprehensive fix across the codebase.
Explanation:
phpcbf
: Executes the PHP Code Beautifier and Fixer command.path/to/directory
: The location of files needing fixes.--extensions php,inc
: Restricts processing to files with.php
and.inc
extensions, thereby excluding other file types.
Example Output: Processed files with specified extensions:
Fixed 25 violations in 2 files of types .php and .inc
Time: 1.22 secs; Memory: 10MB
Use case 5: A comma-separated list of files to load before processing
Code:
phpcbf path/to/directory --bootstrap path/to/file1,path/to/file2
Motivation: Before processing the files, certain scripts might need to be loaded for configuration or environmental setup. This use case allows developers to ensure that necessary scripts are executed, enabling more contextually aware and relevant fixes.
Explanation:
phpcbf
: Command used to run the code fixer.path/to/directory
: Directory containing the PHP files.--bootstrap path/to/file1,path/to/file2
: Specifies additional PHP files to be loaded initially, providing preparatory context for code analysis.
Example Output: Confirmation of bootstrap files loaded and fixes applied:
Loaded bootstrap files: /path/to/file1 and /path/to/file2
Fixed 40 violations in 4 files
Time: 3.10 secs; Memory: 13MB
Use case 6: Don’t recurse into subdirectories
Code:
phpcbf path/to/directory -l
Motivation: Sometimes, fixes are only needed at the root level of a directory without affecting files in subdirectories. This command helps target specific areas without altering unintended files, offering more control over what gets processed.
Explanation:
phpcbf
: Utilizes the PHP Code Beautifier and Fixer.path/to/directory
: Indicates the specific directory for processing.-l
: Tellsphpcbf
not to recurse, acting only on the files found directly in the specified directory.
Example Output: Output illustrating only top-level files processed:
Fixed 10 violations in 1 file at top level
Time: 0.87 secs; Memory: 9MB
Conclusion:
Incorporating phpcbf
into your development workflow can dramatically improve the quality and consistency of your PHP codebase by automating code style fixes. Whether dealing with large projects or maintaining smaller scripts, phpcbf
saves valuable time and effort by ensuring that code adheres to specified standards programmatically, vastly reducing potential human error in manual code reviews. By understanding and utilizing its various options and arguments effectively, developers enhance their productivity while ensuring a clean, manageable codebase.