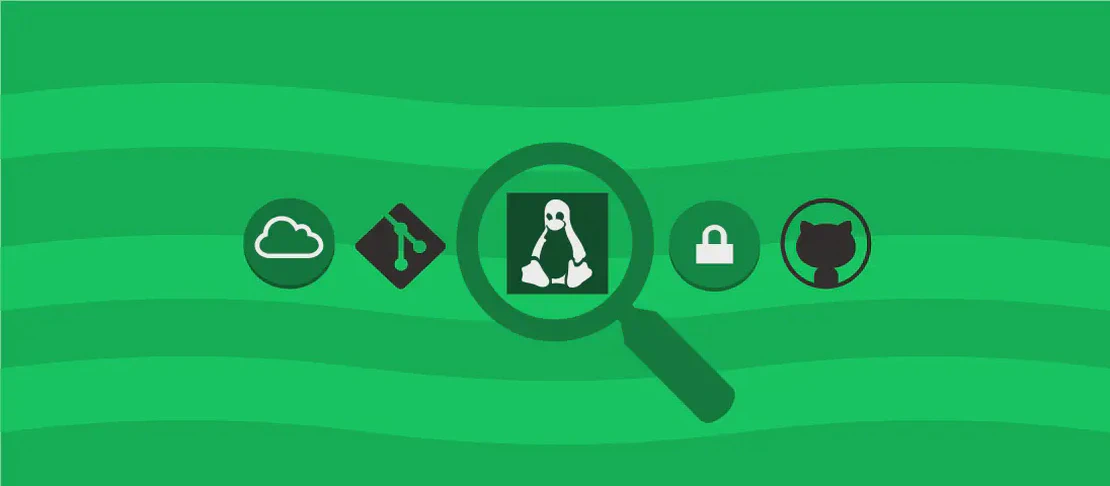
How to Use the Command 'phpcs' (with Examples)
PHP_CodeSniffer (phpcs
) is a powerful tool used by programmers, particularly those working with PHP, JavaScript, and CSS, to ensure that their code adheres to specified coding standards. The tool helps automatically detect and highlight code that doesn’t comply with chosen coding guidelines, thereby maintaining a consistent code style across projects and possibly catching bugs related to improper formatting. The command performs a “sniff” operation on the code to determine if there are any coding standard violations, outputs reports in various formats, and allows configuration of different code standards and file processing rules.
Use case 1: Sniff the specified directory for issues (defaults to the PEAR standard)
Code:
phpcs path/to/directory
Motivation:
In any software development project, adhering to coding standards is critical for maintaining code readability, reliability, and consistency among different developers. By running phpcs
on a directory, a developer can quickly and easily identify portions of code that may not comply with these standards, thus allowing them to rectify issues before the code is merged into a shared codebase.
Explanation:
phpcs
: This is the command to invoke PHP_CodeSniffer.path/to/directory
: Represents the path to the directory where the source code files are located that need to be checked. By default, PHP_CodeSniffer will use the PEAR coding standard unless otherwise specified. The directory path can be an absolute path or relative to the location from which the command is executed.
Example output:
FILE: path/to/directory/example.php
----------------------------------------------------------------------
FOUND 1 ERROR AFFECTING 1 LINE
----------------------------------------------------------------------
14 | ERROR | [x] Line indented incorrectly; expected 4 spaces, found 5
----------------------------------------------------------------------
Time: 30ms; Memory: 10Mb
Use case 2: Display a list of installed coding standards
Code:
phpcs -i
Motivation:
When working within a diverse development environment or when switching between multiple projects, it is crucial for developers to be aware of the available coding standards that can be enforced using PHP_CodeSniffer. This command provides a quick list of all installed coding standards, aiding developers in selecting the appropriate standard for a given project.
Explanation:
phpcs
: Initiates the PHP_CodeSniffer tool.-i
: This argument stands for “installed coding standards” and instructs PHP_CodeSniffer to display the list of coding standards that have been installed on the system. This offers instant insight into which standards are available for use.
Example output:
The installed coding standards are MySource, PEAR, PSR1, PSR12, Squiz, Zend
Use case 3: Specify a coding standard to validate against
Code:
phpcs path/to/directory --standard=PSR12
Motivation:
Different projects or organizations may adhere to different coding guidelines. By specifying a particular coding standard, such as PSR-12 for PHP, developers can ensure their code complies with industry or organizational benchmarks, promoting consistency and quality in the codebase.
Explanation:
phpcs
: Executes the code sniffing process.path/to/directory
: The path to the directory or file to be checked.--standard=PSR12
: This option specifies that the PSR-12 coding standard should be applied during the sniffing process. PSR-12 is one such widely recognized standard used for PHP coding, and specifying it ensures that code complies with its rules.
Example output:
FILE: path/to/directory/example.php
----------------------------------------------------------------------
FOUND 3 ERRORS AFFECTING 3 LINES
----------------------------------------------------------------------
13 | ERROR | [x] PSR-12: Files must not have trailing whitespace.
48 | ERROR | [x] PSR-12: Each class must be in a namespace of at least one level (a vendor name)
98 | ERROR | [x] PSR-12: Lines should not exceed 120 characters; contains 132 characters
----------------------------------------------------------------------
Time: 45ms; Memory: 10Mb
Use case 4: Specify comma-separated file extensions to include when sniffing
Code:
phpcs path/to/directory --extensions=php,css
Motivation:
In multi-language projects, developers often need to check only specific types of files. The --extensions
flag allows them to focus on relevant files, optimizing the sniffing process to save time by ignoring unnecessary files and ensuring attention is given only to pertinent code types such as PHP and CSS.
Explanation:
phpcs
: Starts the code analysis.path/to/directory
: The directory containing the source code files.--extensions=php,css
: By providing this option, the developer tells PHP_CodeSniffer to process only the files with the extensions.php
and.css
within the specified directory. This selects the relevant files and excludes others, such as JavaScript or HTML files, from being sniffed.
Example output:
Processing paths: path/to/directory
Only processing .php and .css files...
No violations found
Use case 5: Specify the format of the output report (e.g., full
, xml
, json
, summary
)
Code:
phpcs path/to/directory --report=summary
Motivation:
Different projects or teams might require different reporting formats based on their workflow or tools. The ability to specify an output format such as summary
, xml
, or json
provides the flexibility to integrate with other tools (e.g., continuous integration pipelines) and enables teammates to view reports in the manner most useful to them.
Explanation:
phpcs
: Triggers PHP_CodeSniffer.path/to/directory
: Points to the target directory for sniffing.--report=summary
: This argument specifies that the output should be a summary report. This means PHP_CodeSniffer will provide a concise list of findings, rather than a detailed per-line report, enabling quick assessment of code health at a glance.
Example output:
A TOTAL OF 3 ERRORS WERE FOUND IN 2 FILES
SUMMARY REPORT
----------------------------------------------------------------------
FOUND 2 ERRORS AND 0 WARNINGS AFFECTING 1 FILE
----------------------------------------------------------------------
path/to/directory/file1.php
14 | ERROR | [x] Line indented incorrectly; expected 4 spaces, found 5
32 | ERROR | [x] Missing function doc comment
FOUND 1 ERROR AND 0 WARNINGS AFFECTING 1 FILE
----------------------------------------------------------------------
path/to/directory/file2.php
18 | ERROR | [x] Missing function doc comment
Use case 6: Set configuration variables to be used during the process
Code:
phpcs path/to/directory --config-set ignoreWarningsOnExit 1
Motivation:
Customization is key to effective tool use, and PHP_CodeSniffer allows users to set configuration variables that alter how it behaves. By setting options like ignoreWarningsOnExit
, a developer can tailor PHP_CodeSniffer to fit more neatly into their workflow, for instance, by ensuring that only errors (and not warnings) trigger a failed build status in an automated process.
Explanation:
phpcs
: Initiates the sniffing operation.path/to/directory
: Identifies the directory containing the target files.--config-set ignoreWarningsOnExit 1
: This setting configures PHP_CodeSniffer to ignore warnings. The argumentignoreWarningsOnExit
with a value of1
means that the tool will not consider warnings when determining the exit status, suitable for situations where developers are only concerned with more severe issues.
Example output:
Config value "ignoreWarningsOnExit" added successfully
Use case 7: A comma-separated list of files to load before processing
Code:
phpcs path/to/directory --bootstrap=path/to/file1.php,path/to/file2.php
Motivation:
In complex projects, there are often dependencies or setup routines required before certain files should be processed for sniffing. The --bootstrap
parameter allows developers to specify files that should be automatically included, facilitating proper context or environment setup that might be needed for accurate sniffing results.
Explanation:
phpcs
: Executes the PHP_CodeSniffer utility.path/to/directory
: Specifies the directory for code review.--bootstrap=path/to/file1.php,path/to/file2.php
: Indicates files to be loaded prior to performing the sniffing task. This permits pre-loading of necessary scripts, which might set configurations or load dependencies required for the code check.
Example output:
Bootstrap file "path/to/file1.php" loaded
Bootstrap file "path/to/file2.php" loaded
No violations found
Use case 8: Don’t recurse into subdirectories
Code:
phpcs path/to/directory -l
Motivation:
In cases where developers only wish to review the code within a specific directory without processing subdirectories, perhaps to focus on a newly added module or avoid old code, the -l
option (short for “local only”) is invaluable. It allows a concentrated analysis of files only directly present in the given directory.
Explanation:
phpcs
: Specifies the use of the PHP_CodeSniffer.path/to/directory
: Points out the directory to be scanned.-l
: This flag limits the sniffing operation to the specified directory alone, preventing recursion into subdirectories. This option is particularly useful for a quick check or when the directory contains a large directory tree.
Example output:
Processing all files in: path/to/directory (local only)
No violations found
Conclusion:
Using PHP_CodeSniffer to enforce coding standards provides a systematic approach to maintaining code quality and consistency across PHP, JavaScript, and CSS files. The command offers numerous options to customize its behavior, from specifying code standards and file types to tailoring reports and pre-loading necessary scripts. By leveraging these diverse capabilities, developers can ensure their code not only functions properly but also meets established style guidelines, making it more sustainable, readable, and less error-prone over time.